Write the implementation of a class called Shape (square, triangle, parallelogram...etc), that consists of a linked list of Points. - Class shape has the following private attributes: String variable to store the name of the shape. Two pointer variables of type nodeType to point to the first and last nodes. Integer variable to store the length of the list of points. - Integer variable to store the maximum size of the list of points according to the shape. - For this class you should provide the following public member functions: A constructor that takes the shape name and the maximum number of points in the shape as parameters. A copy constructor. A function to overload assignment (=) operator. A destructor that frees all dynamically allocated memory when the object is destroyed. A function to calculate the circumference of a shape. A function to print the existing points in the shape.
Write the implementation of a class called Shape (square, triangle, parallelogram...etc), that consists of a linked list of Points. - Class shape has the following private attributes: String variable to store the name of the shape. Two pointer variables of type nodeType to point to the first and last nodes. Integer variable to store the length of the list of points. - Integer variable to store the maximum size of the list of points according to the shape. - For this class you should provide the following public member functions: A constructor that takes the shape name and the maximum number of points in the shape as parameters. A copy constructor. A function to overload assignment (=) operator. A destructor that frees all dynamically allocated memory when the object is destroyed. A function to calculate the circumference of a shape. A function to print the existing points in the shape.
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
100%
![Write the implementation of a class called Shape (square, triangle, parallelogram.etc), that consists of a linked list
of Points.
> Class shape has the following private attributes:
String variable to store the name of the shape.
Two pointer variables of type node Type to point to the first and last nodes.
Integer variable to store the length of the list of points.
Integer variable to store the maximum size of the list of points according to the shape.
- For this class you should provide the following public member functions:
A constructor that takes the shape name and the maximum number of points in the shape as
parameters.
A copy constructor.
A function to overload assignment (=) operator.
A destructor that frees all dynamically allocated memory when the object is destroyed.
A function to calculate the circumference of a shape.
A function to print the existing points in the shape.
A function to overload the [] operator to return a reference to the point in the specified index
passed as argument if the index is within a valid range otherwise return NULL.
A function to overload the + operator to add a point at the beginning of the list, ensure that number
of nodes after insertion will not exceed maximum number of nodes allowed for this shape.
Overload the += operator to add a point at the end of the list, ensure that number of nodes after
insertion will not exceed maximum number of nodes allowed for this shape.
- Overload the following operators as nonmember functions
A function to overload prefix –- operator to delete a point from the beginning of the list, ensure
not to delete from an empty list.
A function to overload postfix -- operator to delete a node from the end of the list, ensure not to
delete from an empty list.
- A function to overload - operator to delete a point at a specified index. Make sure that the
specified index is within valid range. And use [] operator in this function.
- A function to overload > operator to compare the circumference of two shapes. (first you should
ensure that the two shape have same number of sides and all the shape points are entered)
A function to overload == operator to check if the two shapes are identical return true otherwise
return false.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F4183265f-2db3-48a3-a060-b257e6cf60d8%2F31ee1004-905b-447e-a0ad-82f042d0235a%2F5tq3qpf_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Write the implementation of a class called Shape (square, triangle, parallelogram.etc), that consists of a linked list
of Points.
> Class shape has the following private attributes:
String variable to store the name of the shape.
Two pointer variables of type node Type to point to the first and last nodes.
Integer variable to store the length of the list of points.
Integer variable to store the maximum size of the list of points according to the shape.
- For this class you should provide the following public member functions:
A constructor that takes the shape name and the maximum number of points in the shape as
parameters.
A copy constructor.
A function to overload assignment (=) operator.
A destructor that frees all dynamically allocated memory when the object is destroyed.
A function to calculate the circumference of a shape.
A function to print the existing points in the shape.
A function to overload the [] operator to return a reference to the point in the specified index
passed as argument if the index is within a valid range otherwise return NULL.
A function to overload the + operator to add a point at the beginning of the list, ensure that number
of nodes after insertion will not exceed maximum number of nodes allowed for this shape.
Overload the += operator to add a point at the end of the list, ensure that number of nodes after
insertion will not exceed maximum number of nodes allowed for this shape.
- Overload the following operators as nonmember functions
A function to overload prefix –- operator to delete a point from the beginning of the list, ensure
not to delete from an empty list.
A function to overload postfix -- operator to delete a node from the end of the list, ensure not to
delete from an empty list.
- A function to overload - operator to delete a point at a specified index. Make sure that the
specified index is within valid range. And use [] operator in this function.
- A function to overload > operator to compare the circumference of two shapes. (first you should
ensure that the two shape have same number of sides and all the shape points are entered)
A function to overload == operator to check if the two shapes are identical return true otherwise
return false.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
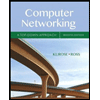
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
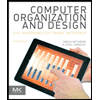
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
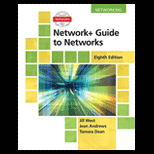
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
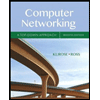
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
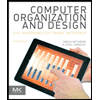
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
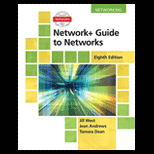
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
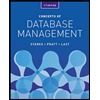
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
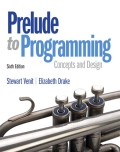
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
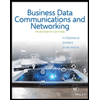
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY