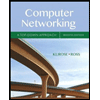
Task 1: Find the longest word
Implement the function findLongestWord that takes as an argument an array of words and returns the longest one. If there are 2 with the same length, it should return the first occurrence. Write the JS code in a separate file.
You can use the following array to test your solution:
var words = ['mystery', 'brother', 'aviator', 'crocodile', 'pearl', 'orchard', 'crackpott'];

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

Task 2:
Implement the function Searchingstrings that allow to search a sub-string in a given string and display the: First occurrence, Last occurrence, First occurrence from a given index and Last occurrence from a given index.
You can use the following String methods:
string. IndexOf(searchvalue, start) return the position of the first occurrence of specified character(s) in a string.
string.lastIndexOf(searchvalue, start) return the position of the last occurrence of specified character(s) in a string.
You can use the following string to test your solution:
var letters = "abcdefghijklmnopqrstuvwxyzabcdefghijklm";
Write the code in a separate.js and separate.html file.
Task 2:
Implement the function Searchingstrings that allow to search a sub-string in a given string and display the: First occurrence, Last occurrence, First occurrence from a given index and Last occurrence from a given index.
You can use the following String methods:
string. IndexOf(searchvalue, start) return the position of the first occurrence of specified character(s) in a string.
string.lastIndexOf(searchvalue, start) return the position of the last occurrence of specified character(s) in a string.
You can use the following string to test your solution:
var letters = "abcdefghijklmnopqrstuvwxyzabcdefghijklm";
Write the code in a separate.js and separate.html file.
- 1. declare an array to hold 12 floating point numbers: 2. Now that we have our array, set all 12 numbers to -100. 3. Change all even positions (all lines with an even index: 0,2,4, etc) to 96.8Use a function call to print the array.arrow_forward2arrow_forwardCreate a function named "onlyOdd".The function should accept a parameter named "numberArray".Use the higher order function filter() to create a new array that only containsthe odd numbers. Return the new array.// Use this array to test your function:const testingArray = [1, 2, 4, 17, 19, 20, 21];arrow_forward
- Write a function named swapFrontback that takes as input an array ofintegers and an integer that specifies how many entries are in the array. Thefunction should swap the first element in the array with the last elements inthe array. The function should check if the array is empty to prevent errors.The header file for the swapFrontback() is:swapFrontback.h:double swapFrontback (int [] arr,int size);Write a driver (main.c) to test your function with arrays of different lengthand with varrying front and back numbers. Print the array elements beforeand after the swap in the main.c.Sample Run: Original Array: 1 2 3 4 5 6 FrontBack Array: 6 2 3 4 5 1arrow_forwardC++arrow_forward1- Write a user-defined function that accepts an array of integers. The function should generate the percentage each value in the array is of the total of all array values. Store the % value in another array. That array should also be declared as a formal parameter of the function. 2- In the main function, create a prompt that asks the user for inputs to the array. Ask the user to enter up to 20 values, and type -1 when done. (-1 is the sentinel value). It marks the end of the array. A user can put in any number of variables up to 20 (20 is the size of the array, it could be partially filled). 3- Display a table like the following example, showing each data value and what percentage each value is of the total of all array values. Do this by invoking the function in part 1.arrow_forward
- mergeAndRemove(int[], int[]) This is a public static function that takes a int[] and int[] for the parameters and returns an int[]. Given two arrays of integers. Your job is to combine them into a single array and remove any duplicates, finally return the merged array.arrow_forward/* (name header) #include // Function declaration int main() { int al[] int a2[] = { 7, 2, 10, 9 }; int a3[] = { 2, 10, 7, 2 }; int a4[] = { 2, 10 }; int a5[] = { 10, 2 }; int a6[] = { 2, 3 }; int a7[] = { 2, 2 }; int a8[] = { 2 }; int a9[] % { 5, 1, б, 1, 9, 9 }; int al0[] = { 7, 6, 8, 5 }; int all[] = { 7, 7, 6, 8, 5, 5, 6 }; int al2[] = { 10, 0 }; { 10, 3, 5, 6 }; %3D std::cout « ((difference (al, 4) « ((difference(a2, 4) <« ((difference (a3, 4) « ((difference(a4, 2) <« ((difference (a5, 2) <« ((difference (a6, 2) <« ((difference (a7, 2) <« ((difference(a8, 1) « ((difference (a9, 6) « ((difference(a10, 4) " <« ((difference(al1, 7) « ((difference (al2, 2) == 10) ? "OK\n" : "X\n"); << "al: << "a2: %3D 7)? "OK" : "X") << std::endl == 8) ? "OK\n" : "X\n") 8) ? "OK\n" : "X\n") == 8) ? "OK\n" : "X\n") == 8) ? "OK\n" : "X\n") == 1) ? "OK\n" : "X\n") == 0) ? "OK\n" : "X\n") 0) ? "OK\n" : "X\n") == 8) ? "OK\n" : "X\n") == 3) ? "OK\n" : "X\n") == 3) ? "OK\n" : "X\n") == << "a3: == << "a4: <<…arrow_forwardCODE IN JAVA design a program that grades arithmetic quizzes as follows: (Use the below startup code for Quizzes.java and provide code as indicated by the comments) 1. Ask the user how many questions are in the quiz. 2. Use a for loop to load the array. Ask the user to enter the key (that is, the correct answers). There should be one answer for each question in the quiz, and each answer should be an integer. They can be entered on a single line, e.g., 34, 7, 13, 100, 8 might be the key for a 5-question quiz. You will need to store the key in an array called "key". 3. Ask the user to enter the student's answers for the quiz to be graded. There needs to be one answer for each question. Note that each answer can simply be compared to the key as it is entered. If the answer is correct, add 1 to a correct answer counter. need this in a separate for loop from the loop used to load the array. 4. When the user has entered all of the answers to be graded, print the number correct…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
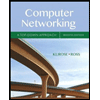
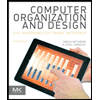
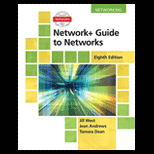
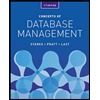
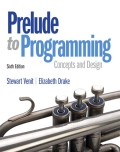
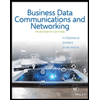