You are given two files mystring1.h download and mystring1.cpp download which defines a String class (noted that upper case S, C++ is case sensitive) implemented by using static array as the data member. The purpose of this is to rewrite this class by replacing the static array with a pointer (dynamic array). You are required to modify the String class accordingly: Data: we wish to allow our String class to accommodate strings of different sizes. To that end, instead of storing a string’s characters in a fixed-size array, each String object should contain the following data as part of its internal representation: (1) a character pointer meant to point to a dynamically allocated array of characters. (2) a length field that will hold the current length of the string at any given moment. Operations: same as the methods in String class, plus the “big three”( destructor, copy constructor, and assignment operator overloading). Modularity: The String class code must be put in its own module, i.e., a file mystring2.h (the header file) should hold the class definition (use conditional compilation directives) and a file mystring2.cpp (the implementation file) should hold the program code for all the functions defined in the header file. Upload two files: mystring2.cpp and mystring2.h. I have attached mystring1.h and mystring1.cpp to the picture.
You are given two files mystring1.h download and mystring1.cpp download which defines a String class (noted that upper case S, C++ is case sensitive) implemented by using static array as the data member. The purpose of this is to rewrite this class by replacing the static array with a pointer (dynamic array). You are required to modify the String class accordingly:
Data: we wish to allow our String class to accommodate strings of different sizes. To that end, instead of storing a string’s characters in a fixed-size array, each String object should contain the following data as part of its internal representation:
(1) a character pointer meant to point to a dynamically allocated array of characters.
(2) a length field that will hold the current length of the string at any given moment.
Operations: same as the methods in String class, plus the “big three”( destructor, copy constructor, and assignment operator overloading).
Modularity: The String class code must be put in its own module, i.e., a file mystring2.h (the header file) should hold the class definition (use conditional compilation directives) and a file mystring2.cpp (the implementation file) should hold the program code for all the functions defined in the header file. Upload two files: mystring2.cpp and mystring2.h.
I have attached mystring1.h and mystring1.cpp to the picture.
![mystring1 (1) - Notepad
O X
File Edit Format View Help
File: mystring1.cpp
N=
W Implementation file for user-defined String class.
#inc hude "mystring1.h"
String:String()
contents0] = 0
len - 0;
String: String(const char s)
len - strien(sk:
strepy(contents, s);
void String:append(const String &str)
strcatcontents, str.contents);
Jen+ str.len:
bool String:operator (const String &str) const
return stremp(contents, str.contents) 0:
bool String:operator !(const String &str) const
return strempcontents, str.contents) ! 0;
bool String:operator >(const String &str) const
return stremp(contents, str.contents)> 0;
bool String:operator <(const String &str) const
return stremp(contents, str.contents) < 0;
bool String:operator >=(const String &str) const
return stremp(contents, str.contents)> 0;
String String:operator -(const String kstr)
append str)
return *this
void String prin ostream &outh const
out contents
int String:length const
return len;
char Stringoperator ()(int i) const
if (i<0|| i ken)
cerr << "can't access location"<<i
"of string
ce condents e < endl:
return contentii:
Costream & operator(ostream kout, const String & s) overkoad ostream operator ""- External
s.printout);
return out
Ln 53, Col 15
60%
Windows (CRLF)
UTF-8
1:05 AM
O Type here to search
6/3/2021](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F7df0ddbc-181a-4ca1-bfba-4d912965d547%2F1ef29102-d0bd-4e47-85ce-4b0c3aadeed1%2Fjn70lrq_processed.png&w=3840&q=75)
![I mystring1.h - Notepad
O X
File Edit Format View Help
//File: mystring1.h
// Interface file for user-defined String class.
#ifndef MYSTRING H
#define _MYSTRING_H
#include <iostream>
#include <cstring> // for strlen(), etc.
using namespace std;
#define MAX_STR_LENGTH 200
class String {
public:
String();
String(const char s[]); // a conversion constructor
void append(const String &str);
// Relational operators
bool operator =(const String &str) const;
bool operator !=(const String &str) const;
bool operator >(const String &str) const;
bool operator <(const String &str) const;
bool operator >=(const String &str) const;
String operator +=(const String &str);
void print(ostream &out) const;
int length() const;
char operator [](int i) const; // subscript operator
private:
char contents[MAX_STR_LENGTH+1];
int len;
};
ostream & operator<<(ostream &out, const String & r); // overload ostream operator "<<" - External!
#endif /* not defined MYSTRING_H */
Ln 13, Col 1
100%
Windows (CRLF)
UTF-8
梦 $
1:02 AM
O Type here to search
6/3/2021](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F7df0ddbc-181a-4ca1-bfba-4d912965d547%2F1ef29102-d0bd-4e47-85ce-4b0c3aadeed1%2Ff8cm994_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

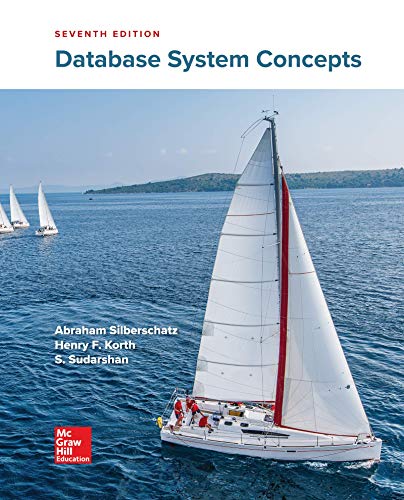
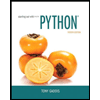
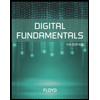
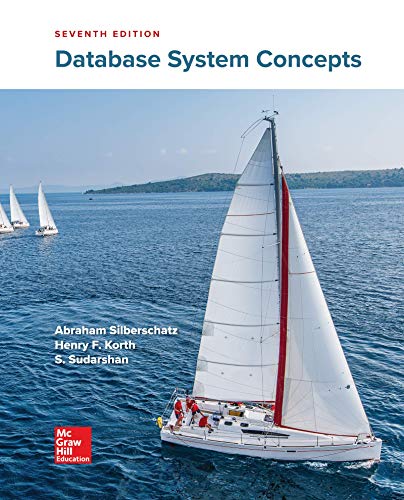
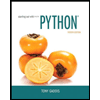
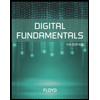
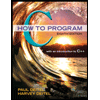
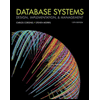
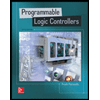