You are working for a financial advisor who creates portfolios of financial securities for his clients. A portfolio is a conglomeration of various financial assets, such as stocks and bonds, that together create a balanced collection of investments. For example: Client-A purchases 100 shares of IBM $1 par value common stock on Jan. 20, 2020, for a total purchase price of $10,000 USD. Dividends for the year have been declared at $5 per share of common. Client-A purchases 5 bonds is1sued by Intel Corporation with a face value of $1000 per bond and a stated interest rate of 7.5% on Jan 3, 2020, for a total purchase price of $5,000 USD. The bonds mature on Dec. 31, 2025. It is your job to create an object-oriented application that will allow the financial advisor to maintain the portfolios for his/her clients. You will need to create several classes to maintain this information: Security, Stock, Bond, Portfolio, and Date. The characteristics of stocks and bonds in a portfolio are shown below: Stocks: Bonds: Purchase date (Date) Purchase date (Date) Purchase price (double) Purchase price (double) Quantity purchased (int) Quantity purchased (int) Ticker symbol (string) Issuer (string) Par value (int) Face value (int) Stock type (i.e. Common or Preferred) (enum) Stated interest rate (double) Dividends per share (double) Maturity date (Date) C++ does not have a built in data type for Date. Therefore, you may use this Date class in your program: #pragma once #include #include #include class Date{ friend std::ostream& operator<<(std::ostream&, Date); public: Date(int d=0, int m=0, int yyyy=0) { setDate(d, m, yyyy); } ~Date() {} void setDate(int d, int m, int yyyy){ day = d; month = m; year = yyyy; } private: int day; int month; int year; }; std::ostream& operator<<(std::ostream& output, Date d){ output << d.month << "/" << d.day << "/" << d.year; return output; Security class (base class) The class should contain data members that are common to both Stocks and Bonds. Write appropriate member functions to store and retrieve information in the member variables above. Be sure to include a constructor and destructor. Stock class and Bond class. (derived classes) Each class should be derived from the Security class. Each should have the member variables shown above that are unique to each class. Each class should contain a function called calcIncome that calculates the amount a client receives as dividend or interest income for each security purchase. Note that the calcIncome algorithm has been simplified for this assignment. In real life, interest is paid on most bonds semi-annually, and dividends are declared by a company once per year. In our example, we are assuming that dividends are known at the time of the stock purchase and are not subject to change. We are also assuming an annual (as opposed to semi-annual) payment of interest on bonds. To calculate a stock puchase’s dividend income, use the following formula: income = dividends per share * number of shares. To calculate a bond purchase’s annual income, use this formula: income = number of bonds in purchase * the face value of the bonds * the stated interest rate. In each derived class, the << operator should be overloaded to output, neatly formatted, all of the information associated with a stock or bond, including the calculated income. The < operator should also be overloaded in each derived class to enable sorting of the vectors using the sort function. This function is part of the library. Write member functions to store and retrieve information in the appropriate member variables. Be sure to include a constructor and destructor in each derived class. Design a Portfolio class The portfolio has a name data member. The class contains a vector of Stock objects and a vector of Bond objects. Main() You should write a main() program that creates a portfolio and presents a menu to the user that looks like this: “S” should allow the user to record the purchase of some stocks and add the purchase to the Stocks list. Likewise, “B” should allow the user to record the purchase of some bonds and add the purchase to the Bonds list. “L” should list all of the securities in the portfolio, first displaying all of the Stocks, sorted by ticker symbol, followed by all of the bonds, sorted by issuer. The user should be able to add stocks, add bonds, and list repeatedly until he or she selects “Q” to quit.
Can Someone help me create this code?
You are working for a financial advisor who creates portfolios of financial securities for his clients. A portfolio is a conglomeration of various financial assets, such as stocks and bonds, that together create a balanced collection of investments.
For example:
Client-A purchases 100 shares of IBM $1 par value common stock on Jan. 20, 2020, for a total purchase price of $10,000 USD. Dividends for the year have been declared at $5 per share of common.
Client-A purchases 5 bonds is1sued by Intel Corporation with a face value of $1000 per bond and a stated interest rate of 7.5% on Jan 3, 2020, for a total purchase price of $5,000 USD. The bonds mature on Dec. 31, 2025.
It is your job to create an object-oriented application that will allow the financial advisor to maintain the portfolios for his/her clients. You will need to create several classes to maintain this information: Security, Stock, Bond, Portfolio, and Date.
The characteristics of stocks and bonds in a portfolio are shown below:
Stocks: Bonds:
Purchase date (Date) Purchase date (Date)
Purchase price (double) Purchase price (double)
Quantity purchased (int) Quantity purchased (int)
Ticker symbol (string) Issuer (string)
Par value (int) Face value (int)
Stock type (i.e. Common or Preferred) (enum) Stated interest rate (double)
Dividends per share (double) Maturity date (Date)
C++ does not have a built in data type for Date. Therefore, you may use this Date class in your program:
#pragma once
#include <iostream>
#include <cstdlib>
#include <cctype>
class Date{
friend std::ostream& operator<<(std::ostream&, Date);
public:
Date(int d=0, int m=0, int yyyy=0) {
setDate(d, m, yyyy);
}
~Date() {}
void setDate(int d, int m, int yyyy){
day = d;
month = m;
year = yyyy;
}
private:
int day;
int month;
int year;
};
std::ostream& operator<<(std::ostream& output, Date d){
output << d.month << "/" << d.day << "/" << d.year;
return output;
Security class (base class)
The class should contain data members that are common to both Stocks and Bonds.
Write appropriate member functions to store and retrieve information in the member variables above. Be sure to include a constructor and destructor.
Stock class and Bond class. (derived classes)
Each class should be derived from the Security class. Each should have the member variables shown above that are unique to each class.
Each class should contain a function called calcIncome that calculates the amount a client receives as dividend or interest income for each security purchase. Note that the calcIncome
To calculate a stock puchase’s dividend income, use the following formula: income = dividends per share * number of shares. To calculate a bond purchase’s annual income, use this formula: income = number of bonds in purchase * the face value of the bonds * the stated interest rate.
In each derived class, the << operator should be overloaded to output, neatly formatted, all of the information associated with a stock or bond, including the calculated income.
The < operator should also be overloaded in each derived class to enable sorting of the
Write member functions to store and retrieve information in the appropriate member variables. Be sure to include a constructor and destructor in each derived class.
Design a Portfolio class
The portfolio has a name data member.
The class contains a vector of Stock objects and a vector of Bond objects.
Main()
You should write a main() program that creates a portfolio and presents a menu to the user that looks like this:
“S” should allow the user to record the purchase of some stocks and add the purchase to the Stocks list. Likewise, “B” should allow the user to record the purchase of some bonds and add the purchase to the Bonds list. “L” should list all of the securities in the portfolio, first displaying all of the Stocks, sorted by ticker symbol, followed by all of the bonds, sorted by issuer. The user should be able to add stocks, add bonds, and list repeatedly until he or she selects “Q” to quit.
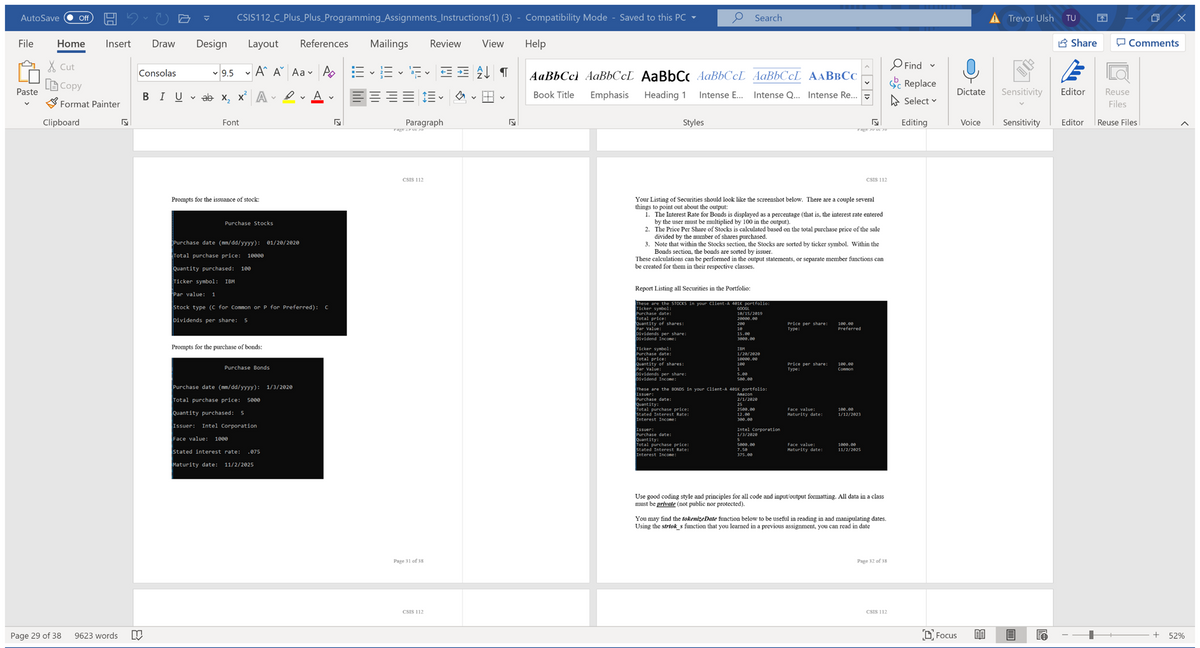
![CSIS 112
CSIS 112
values as a character array, and then tokenize each part into a meaningful day, month, and year
that can be used to initialize a Date variable:
Overloaded « in each derived class to output a stock or bond
Portfolio class
Correct Data Members
Add new stock functionality
Add new bond functionality
Functions to Sort each vector
Function to display all securities data
Date class
void someFunction() //function that uses tokenizeDate
{
int m, d, y;
char charDate[20]; //holds the date the user entered in char array
Date realDate; //date object; holds date after tokenization
std::cout << "\nEnter a date (mm/dd/yyyy): ";
std::cin >> charDate;
ciass
Class is included and used appropriately
Style:
Separation of header and implementation files; Appropriate use of inheritance
and composition; modular code, no globals
tokenizeDate(charDate, m, d, y); //separates char array into month, day, and year
realDate.setDate(d, m, y); //sets the date of the object using the parsed values
}
void tokenizeDate(char cDate, int& month, int& day, int& year)
Review the submission instructions document prior to uploading your work to Blackboard.
char seps[] - "/";
char* token - NULL;
char next_token - NULL;
Submit this assignment by 11:59 p.m. (ET) on Monday of Module/Week 7.
token - NULL;
next_token - NULL;
// Establish string and get the tokens:
token = strtok_s(cDate, seps, &next_token);
month = atoi(token);
token = strtok_s (NULL, seps, &next_token);
day - atoi(token);
token - strtok_s (NULL, seps, &next_token);
year = atoi(token);
}
To give you an idea of the general criteria that will be used for grading, here is a checklist that
you might find helpful:
Executes without crashing
Appropriate Internal Documentation
Security class
Correct Data Members
Correct functions created
Appropriate constructor(s)/destructor(s)
Stock and Bond classes
Derived from Security
Correct Data Members
Correct functions created
Appropriate constructor(s)/destructor(s)
Overloaded < operator. Implemented in the Stock class to compare two ticker
symbols for stock objects. Implemented in the Bond class to compare two
issuers.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F546860fe-98c5-4cbb-83c9-6bb3ac138cc4%2F6a259a75-8311-4233-888d-26e832a7dde0%2Fdxlizjm_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 6 steps

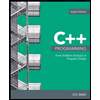
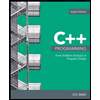