I have the whole thing almost done just need the area that says ":::::Need help here::::". Thank you! Please complete the following function getGrade, which accepts only one parameter (int score), and must return its proper grade (i.e., char grade) to the caller. This function must determine the proper grade based on the score received as follows: grade = 'A' if score == 4 ; grade = 'B' if score == 3 ; grade = 'C' if score == 2 ; grade = 'D' if score == 1 ; grade = 'F' if score == 0 ; Otherwise, grade = '?' ; char getGrade( int score) // function returns a char to the caller { // parameter 1: int score (4 for example) // return: char grade (‘A’ for example) char grade = '?' ; // This is the only variable you can use ::::::::::::: Enter your code here ::::::::::::::::::::::::::::::::: return grade ; } // end char getGrade( ) void main() // To test function getGrade( ) { cout << "score 4 -> grade = " << getGrade(4) << endl; cout << "score 3 -> grade = " << getGrade(3) << endl; cout << "score 2 -> grade = " << getGrade(2) << endl; cout << "score 1 -> grade = " << getGrade(1) << endl; cout << "score 0 -> grade = " << getGrade(0) << endl; cout << "score 5 -> grade = " << getGrade(5) << endl; } // end main() The output of the above program should be as follows: score 4 -> grade = A score 3 -> grade = B score 2 -> grade = C score 1 -> grade = D score 0 -> grade = F score 5 -> grade = ?
I have the whole thing almost done just need the area that says ":::::Need help here::::". Thank you!
Please complete the following function getGrade, which accepts only one parameter (int score), and must return its proper grade (i.e., char grade) to the caller. This function must determine the proper grade based on the score received as follows:
grade = 'A' if score == 4 ;
grade = 'B' if score == 3 ;
grade = 'C' if score == 2 ;
grade = 'D' if score == 1 ;
grade = 'F' if score == 0 ;
Otherwise, grade = '?' ;
char getGrade( int score) // function returns a char to the caller
{ // parameter 1: int score (4 for example)
// return: char grade (‘A’ for example)
char grade = '?' ; // This is the only variable you can use
::::::::::::: Enter your code here :::::::::::::::::::::::::::::::::
return grade ;
} // end char getGrade( )
void main() // To test function getGrade( )
{ cout << "score 4 -> grade = " << getGrade(4) << endl;
cout << "score 3 -> grade = " << getGrade(3) << endl;
cout << "score 2 -> grade = " << getGrade(2) << endl;
cout << "score 1 -> grade = " << getGrade(1) << endl;
cout << "score 0 -> grade = " << getGrade(0) << endl;
cout << "score 5 -> grade = " << getGrade(5) << endl;
} // end main()
The output of the above program should be as follows:
score 4 -> grade = A
score 3 -> grade = B
score 2 -> grade = C
score 1 -> grade = D
score 0 -> grade = F
score 5 -> grade = ?

Step by step
Solved in 2 steps with 2 images

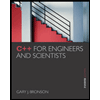
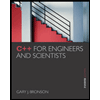