You’ve been hired by Ford Car Company to write a C++ console application that determines the number of months to repay a car loan. Use a validation loop to prompt for and get from the user the car loan amount in the range $2,000-6,000. Then use a validation loop to prompt for and get from the user the monthly payment in the range $300-800. Then use a validation loop to prompt for and get from the user the annual interest rate in the range 3.5-8.5%. Convert the valid rate to a monthly rate and to a decimal number with formula: rate / 12 / 100 Loop to show the loan being repaid and keep track of the number of months it takes to do so. Before the loop, initialize the balance to the loan amount and months to zero. Within the loop, calculate the interest portion of each monthly payment, the principal portion of the payment, and the new balance with formulas: interest = balance * rate; principal = payment - interest; balance = balance - principal; Continue to loop while the balance is greater than zero. After the loop, print the number of months it took to repay the loan. Format the output in columns and show all real numbers to two decimal places. Here is sample output: Welcome to Ford Car Company ------------------------- Enter the car loan amount ($2K-6K): 1000 ERROR: '1000.00' is an invalid loan amount. Enter the car loan amount ($2K-6K): 3000 Enter the monthly payment ($300-800): 350 Enter the annual interest rate (3.5-8.5%): 4.5 Month Balance($) Payment($) Interest($) Principal($) 1 2661.25 350.00 11.25 338.75 2 2321.23 350.00 9.98 340.02 3 1979.93 350.00 8.70 341.30 4 1637.36 350.00 7.42 342.58 5 1293.50 350.00 6.14 343.86 6 948.35 350.00 4.85 345.15 7 601.91 350.00 3.56 346.44 8 254.16 350.00 2.26 347.74 9 -94.88 350.00 0.95 349.05 Months to repay loan: 9 End of Ford Car Company Run the program with the following inputs: Loan amount Monthly payment Annual interest rate 6,000 100 3,000 700 4.5 256.82 8 5 4,000 292.91 4 5,000 319.18 3
You’ve been hired by Ford Car Company to write a C++ console application that determines the number of months to repay a car loan. Use a validation loop to prompt for and get from the user the car loan amount in the range $2,000-6,000. Then use a validation loop to prompt for and get from the user the monthly payment in the range $300-800. Then use a validation loop to prompt for and get from the user the annual interest rate in the range 3.5-8.5%. Convert the valid rate to a monthly rate and to a decimal number with formula:
rate / 12 / 100
Loop to show the loan being repaid and keep track of the number of months it takes to do so. Before the loop, initialize the balance to the loan amount and months to zero. Within the loop, calculate the interest portion of each monthly payment, the principal portion of the payment, and the new balance with formulas:
interest = balance * rate;
principal = payment - interest;
balance = balance - principal;
Continue to loop while the balance is greater than zero. After the loop, print the number of months it took to repay the loan. Format the output in columns and show all real numbers to two decimal places. Here is sample output:
Welcome to Ford Car Company
-------------------------
Enter the car loan amount ($2K-6K): 1000
ERROR: '1000.00' is an invalid loan amount.
Enter the car loan amount ($2K-6K): 3000
Enter the monthly payment ($300-800): 350
Enter the annual interest rate (3.5-8.5%): 4.5
Month Balance($) Payment($) Interest($) Principal($)
1 2661.25 350.00 11.25 338.75
2 2321.23 350.00 9.98 340.02
3 1979.93 350.00 8.70 341.30
4 1637.36 350.00 7.42 342.58
5 1293.50 350.00 6.14 343.86
6 948.35 350.00 4.85 345.15
7 601.91 350.00 3.56 346.44
8 254.16 350.00 2.26 347.74
9 -94.88 350.00 0.95 349.05
Months to repay loan: 9
End of Ford Car Company
Run the program with the following inputs:
Loan amount |
Monthly payment |
Annual interest rate |
6,000 |
100 |
|
3,000 |
700 |
4.5 |
|
256.82 |
8 |
|
|
5 |
4,000 |
292.91 |
4 |
5,000 |
319.18 |
3 |

Step by step
Solved in 3 steps with 5 images

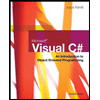
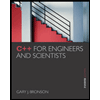
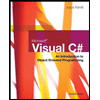
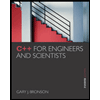