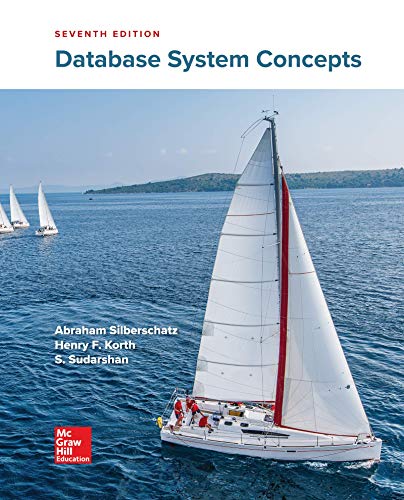
This is in C++.
Given a ListItem class, complete main() using the built-in list type to create a linked list called shoppingList. The
EX INPUT
milk
bread
eggs
waffles
cereal
-1
Ex OUTPUT
milk
bread
eggs
waffles
cereal
main.cpp
#include "ListItem.h"
#include <string>
#include <list>
#include <iostream>
using namespace std;
int main (int argc, char* argv[]) {
// TODO: Declare a list called shoppingList of type ListItem
string item;
// TODO: Read inputs (items) and add them to the shoppingList list
// Read inputs until a -1 is input
// TODO: Print the shoppingList list using the PrintNodeData() function
return 0;
}
ListItem.h (marked as read only)
#ifndef LISTITEMH
#define LISTITEMH
#include <string>
using namespace std;
class ListItem {
public:
ListItem();
ListItem(string itemInit);
// Print this node
void PrintNodeData();
private:
string item;
};
#endif
ListItem.cpp (read only)
#include "ListItem.h"
#include <iostream>
ListItem::ListItem() {
item = "";
}
ListItem::ListItem(string itemInit) {
item = itemInit;
}
// Print this node
void ListItem::PrintNodeData() {
cout << item << endl;
}
Thank you

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

why could you use while(true) without setting a boolean to true?
why could you use while(true) without setting a boolean to true?
- Write a function that will accept a list of integers and return the sum of the contents of the list(……if the list contains 1,2,3 it will return 6…..)arrow_forwardThe function list_combination takes two lists as arguments, list_one and list_two. Return from the function the combination of both lists - that is, a single list such that it alternately takes elements from both lists starting from list_one. You can assume that both list_one and list_two will be of equal lengths. As an example if list_one = [1,3,5] and if list_two = [2,4,6]. The function must return [1,2,3,4,5,6].arrow_forwardprogram7.pyThis assignment requires the main function and a custom value-returning function. The value-returning function takes a list of random integers as its only argument and returns a smaller list of only the elements that end with 7. This value-returning function must use a list comprehension to create this smaller list.In the main function, code these steps in this sequence: Set random seed to 42 import randomrandom.seed(42) create an empty list that will the hold random integers. use a loop to add 50 random integers to the list. All integers should be between 200 and 250, inclusive. Duplicates are okay. sort the list in ascending order and then use another loop to display all 50 sorted integers on one line separated by spaces. print a slice showing list elements indexed 5 through 10, inclusive. print a second slice showing the final 5 elements in the sorted list. execute the custom function with the entire original list as its sole argument. report the number of elements in…arrow_forward
- PLEASE DO NOT USE BUILT IN FILES OR FUNCTIONS! Write a program in c++ and make sure it works, that reads a list of students (first names only) from a file. It is possible for the names tobe in unsorted order in the file but they have to be placed in sorted order within the linked list.The program should use a doubly linked list.Each node in the doubly linked list should have the student’s name, a pointer to the next student, and apointer to the previous student. Here is a sample visual. The head points to the beginning of the list. Thetail points to the end of the list.When inserting consider all the following conditions:if(!head){ //no other nodes}else if (strcmp(data, head->name)<0){ //smaller than head}else if (strcmp(data, tail->name)>0){ //larger than tail}else{ //somewhere in the middle} When deleting a student consider all the following conditions:student may be at the head, the tail or in the middleBelow, you will find a sample of what the…arrow_forwardDebug the program debug_me.py. The program should test each of the users in the provided list. · If the list is empty, it should print “There are no users.” · If the user is “Admin,” the program should print “Hello all powerful one.” · Otherwise, for normal users, it should print “You are a normal user.” Test the program with an empty list to confirm correct operation for that case.arrow_forwardWrite a function that accepts two arguments, a list and a number n. The list contains numbers. The function return a list of all the numbers from the input list that are greater than number n. greater_than_n(list, n)arrow_forward
- QUESTION 4 in phython language Write a program that creates a list of N elements (Hint: use append() function), swaps the first and the last element and prints the list on the screen. N should be a dynamic number entered by the user. Sample output: How many numbers would you like to enter?: 5 Enter a number: 34 Enter a number: 67 Enter a number: 23 Enter a number: 90 Enter a number: 12 The list is: [12, 67,23, 90, 34]arrow_forwardWrite a function called find_duplicates which accepts one list as a parameter. This function needs to sort through the list passed to it and find values that are duplicated in the list. The duplicated values should be compiled into another list which the function will return. No matter how many times a word is duplicated, it should only be added once to the duplicates list. NB: Only write the function. Do not call it. For example: Test Result random_words = ("remember","snakes","nappy","rough","dusty","judicious","brainy","shop","light","straw","quickest", "adventurous","yielding","grandiose","replace","fat","wipe","happy","brainy","shop","light","straw", "quickest","adventurous","yielding","grandiose","motion","gaudy","precede","medical","park","flowers", "noiseless","blade","hanging","whistle","event","slip") print(find_duplicates(sorted(random_words))) ['adventurous', 'brainy', 'grandiose', 'light', 'quickest', 'shop', 'straw', 'yielding']…arrow_forwardUsing c++ Contact list: Binary Search A contact list is a place where you can store a specific contact with other associated information such as a phone number, email address, birthday, etc. Write a program that first takes as input an integer N that represents the number of word pairs in the list to follow. Word pairs consist of a name and a phone number (both strings). That list is followed by a name, and your program should output the phone number associated with that name. Define and call the following function. The return value of FindContact is the index of the contact with the provided contact name. If the name is not found, the function should return -1 This function should use binary search. Modify the algorithm to output the count of how many comparisons using == with the contactName were performed during the search, before it returns the index (or -1). int FindContact(ContactInfo contacts[], int size, string contactName) Ex: If the input is: 3 Frank 867-5309 Joe…arrow_forward
- The function should be written in python language. Write a function called "zero_triples" to return all triples in a list of integers that sum to zero. You can assume the list won't contain any duplicates, and a triple should not use the same number more than once. [In]: zero_triples([1, 2, 4]) [Out]: [] [In]: zero_triples([-3, 1, 4, 2]) [Out]: [[1, 2, -3]] [In]: zero_triples([-9, 1, -3, 2, 4, 5, -4, -1]) [Out]: [[-9, 4, 5], [1, -3, 2], [-3, 4, -1], [5, -4, -1]]arrow_forwardProgramming language is latest version of pythonarrow_forwardrite a function numPairs that accepts two arguments, a target number and a list of numbers. The function then returns the count of pairs of numbers from the list that sum to the target number. In the first example the answer is 2 because the pairs (0,3) and (1,2) both sum to 3. The pair can be two of the same number, e.g. (2,2) but only if the two 2’s are separate twos in the list.In the last example below, there are three 2’s, so there are three different pairs (2,2) so there are 5 pairs total that sum to 4. Sample usage: >> numPairs( 3, [0,1,2,3] )2arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
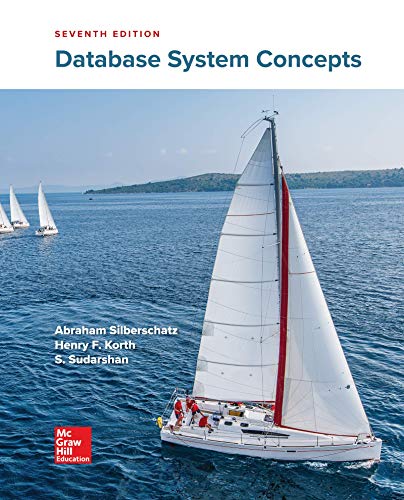
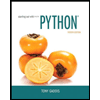
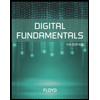
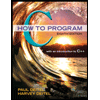
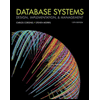
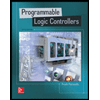