1. Write a program that asks the user to enter three names, and then displays the names sorted alphabetically in descending order. For example, if the user entered "Charlie", “Leslie", and "Andy", the program would display: Leslie Charlie Andy
Need help with step 4. Below are my work for steps 1 and 2 and 3 are the screenshot codes.
THIS IS MY CODE FOR STEP 1 -
import java.util.Scanner;//to readinput
public class Main
{
public static void main(String[] args) {
String s1,s2,s3;//to store 3 names
Scanner sc = new Scanner(System.in);//to read input
//reading 3 names from user
System.out.println("Enter three names :");
s1=sc.next();
s2=sc.next();
s3=sc.next();
System.out.println("Descending order:");
//printing 3 names, in descending order
if(s1.compareTo(s2)>=0 && s2.compareTo(s3)>=0)//if s1>=s2>=s3
{
System.out.println(s1+"\n"+s2+"\n"+s3);
}
else if(s1.compareTo(s3)>=0 && s3.compareTo(s2)>=0)//if s1>=s3>=s2
{
System.out.println(s1+"\n"+s3+"\n"+s2);
}
else if(s2.compareTo(s1)>=0 && s1.compareTo(s3)>=0)//if s2>=s1>=s3
{
System.out.println(s2+"\n"+s1+"\n"+s3);
}
else if(s2.compareTo(s3)>=0 && s3.compareTo(s1)>=0)//if s2>=s3>=s1
{
System.out.println(s2+"\n"+s3+"\n"+s1);
}
else if(s3.compareTo(s1)>=0 && s1.compareTo(s2)>=0)//if s3>=s1>=s2
{
System.out.println(s3+"\n"+s1+"\n"+s2);
}
else if(s3.compareTo(s2)>=0 && s2.compareTo(s1)>=0)//if s3>=s2>=s1
{
System.out.println(s3+"\n"+s2+"\n"+s1);
}
}
}
THIS IS MY CODE FOR STEP 2 -
public static boolean isLeapYear(int year) { return year % 4 == 0 && (year % 100 != 0 || year % 400 == 0); }
public static void main(String[] args) { Scanner keyboard = new Scanner(System.in);
int year; do {
System.out.print("Enter an year(0 to exit): ");
year = keyboard.nextInt();
if (year > 0) {
if (isLeapYear(year)) {
System.out.println(year + " is a leap year");
} else {
System.out.println(year + " is not a leap year"); }
}
} while (year != 0);
}
}
![1 public class Main
2-{
3 public static void main(String args[])
4- {
5 /i for rows and j for columns
6 //row denotes the number of rows you want to print
7 int i, j, row - 7;
8 int x-row2;
9 //Outer Loop work for rows
10 - if(x==®){
11
System.out.print("the given integer is even ");
12 }
13- if(x<=-1){
System.out.println("exit...");
14
15
// Terminate JVM
System.exit(@);
16
17
18 }
19 - if (x--1 && row >0) {
20 for (i=0; i<row; i++)
21- {
22 //inner Loop work for space
23 for (j-row-i; j>l; j--)
24- {
25 //prints space between two stars
26 System.out.print(" ");
27 }
28 //inner Loop for columns
29 for (j-@; j<=i; j++ )
30-{
31 //prints star
32 System.out.print("* ");
33 }
34 //throws the cursor in a new Line after printing each Line
35 System.out.println();
36 }
37 } }
38](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F93f9cbfc-8de6-44b0-bf57-24b8a06d5fd9%2Fcbbc773d-7623-4d8f-9373-448bc30e5de7%2Fwd4wc9h_processed.png&w=3840&q=75)
![1. Write a program that asks the user to enter three names, and then displays the names sorted
alphabetically in descending order. For example, if the user entered "Charlie", “Leslie", and
"Andy", the program would display:
Leslie
Charlie
Andy
Note that these three names are just an example. The names could be any words.
2. Write a program that asks the user to enter a year, and then displays whether that year is a leap
year or not. Note that a year is a leap year if the year is a multiple of 400, or it is a multiple of
4 and not a multiple of 100.
3. Write a program to repeatedly read integers from the standard input until it reads -1 and exits.
For each number before -1, if it is positive and odd, do the following:
• Output a triangle like the one below with the integer being the width of the triangle's bottom
or the number of asterisks of the bottom row. For instance, if the integer is 7, you would
display the triangle below:
***
*****
Or if the integer is not positive or not odd, do nothing and continue to read another integer.
4. A segment of an array is defined as a collection of consecutive elements in that array. The length
of a segment is the number of elements in the segment. A repeating segment is a segment
with all the elements in it having the same value. The following method returns the length
of the longest repeating segment in a given int array. Complete the implementation of the
method.
public int getLength0fLongestRepeatingSegment (int [] array) {
// add your implementation here
2
}
3](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F93f9cbfc-8de6-44b0-bf57-24b8a06d5fd9%2Fcbbc773d-7623-4d8f-9373-448bc30e5de7%2Fhnpv7h9_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

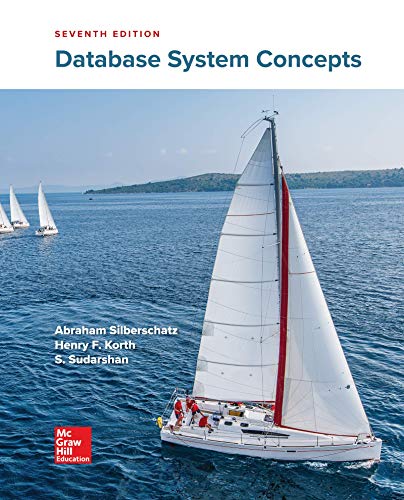
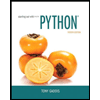
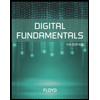
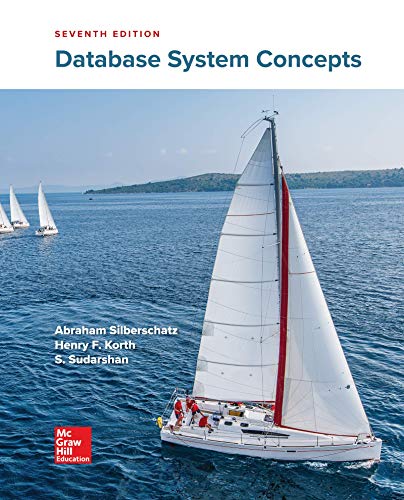
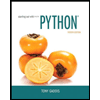
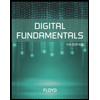
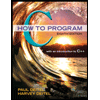
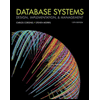
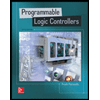