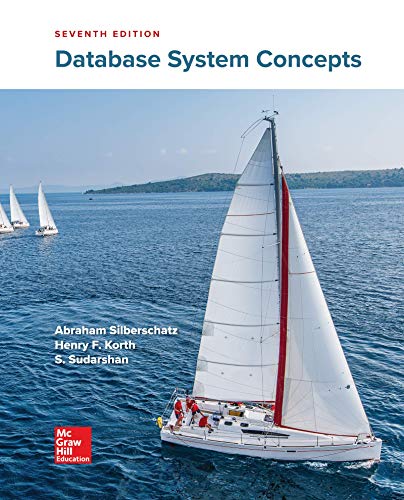
Concept explainers
write a program in python
Write a program that will allow a student to enter their name and then ask them to solve 10 mathematical equations. The program should display two random numbers that are to be added, such as:
247
+ 129
The program should allow the student to enter the answer. The program should then display whether their answer was right or wrong, and accumulate the right values. After the 10 questions are asked, calculate the average that was correct. Then display the student name, the number correct, and the average correct in both decimal and percentage format.
In addition to any system functions you may use, you might consider the following functions:
- A function that allows the student to enter their name.
- A function that gets two random numbers, anywhere from 1 to 500.
- A function that displays the equation and asks the user to enter their answer.
- A function that checks to see if the answer is correct and accumulates the number correct.
- A function that calculates the results.
- A function that displays the student name, the number correct, and the average correct.
. Make sure the inputs to your program are validated.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- The Lottery Problem: • The lottery draws a number that is the winning number • The number should be between 0 and 999 • Have someone play • The person tells how many tickets they want • The person always does “quick pick” (let the computer pick the number for them) and gets one number between 0 and 999 for each ticket bought • The program reports how many tickets win of the ones bought • NOTE: A ticket “wins” if it matches the winning number previously chosen • Write working code with at least one function and one sub other than “main”arrow_forwardPythonarrow_forward//PROBLEM 1/* This program should play a game with the user asking her to guess a target number (randomly generated integer between 1 and 10). The user gets 3 attempts. If she gets it wrong, the program tells her to go up or down, depending on whether her number was less or more then the target. The output may look like this, for example: ============= Guess the number between 1 and 10 in THREE attempts. Enter your first guess: 4 Nope. Go up. Enter your second guess: 6 Nope. Go down. Enter your third and final guess: 5 You got it! CONGRATULATIONS! ============= or like this: ============= Guess the number between 1 and 10 in THREE attempts. Enter your first guess: 4 Nope. Go up. Enter your second guess: 6 Nope. Go up. Enter your third and final guess: 8 GAME OVER! You lost! The number was 9arrow_forward
- IN PYTHON Write a script which uses the input function to read a string, an int, and a float, as input from keyboard prompts the user to enter his/her name as string, his/her age as integer value, and his/her income as a decimal. For example your output will display as: Mark is 30 years old and his income is 2000 Please show code.arrow_forwardJAVA Create a program using JAVA that asks the user to enter the amount that he or she has budgeted for a month. A loop should then prompt the user to enter each of his or her expenses for the month and keep a running total. When the loop finishes, the program should display the first and last name, each entry, and the total amount that the user is over or under budget. EXAMPLE: Paul RuddMonthly Budget Starting Balance: $1,000.00 Restaurant: - $300.00Remaining balance: $800.00 Electric: -$100.00Remaining balance: $600.00 Final Balance: $600.00arrow_forward17. Math Tutor Write a program that can be used as a math tutor for a young student. The program should display two random numbers to be added, such as 247 +129 The program should then pause while the student works on the problem. When the student is ready to check the answer, he or she can press a key and the program will display the correct solution: 247 +129 376arrow_forward
- mystery_value = 5 #You may modify the lines of code above, but don't move them!#When you Submit your code, we'll change these lines to#assign different values to the variables. #Write a program that divides mystery_value by mystery_value#and prints the result. If that operation results in an#error, divide mystery_value by (mystery_value + 5) and then#print the result. If that still fails, multiply mystery_value#by 5 and print the result. You may assume one of those three#things will work.##You may not use any conditionals.# #Add your code here!arrow_forwardCount of Guess Create a JAVA program to generate a random number in the range [1,10] and then prompt the user to guess a number until the user guesses the correct random number generated. The program should print the number of attempts in which the user enters the correct number.arrow_forwardPart 1: Complete the survey. Part 2: Peek-a-boo is a fun game that little kids like to play. To simulate this game on the computer, write a program that will generate a random number between 1 and 4. Then, will print to the screen the animal name associated to that number. The animal names used will be: pig when a 1 is generated cow when a 2 is generated chicken when a 3 is generated horse when a 4 is generated If your program generates a 3, the output will be: chicken The player will then enter a 1 if they would like to play again or anything else to exit the program. If the player enters "1 1 1 0", the output will be: horse chicken cow horse For coding simplicity, follow each output animal by a space, even the last one. Hint: To make testing easier, seed your random number generator with 0.arrow_forward
- Python questionarrow_forwardThe Café Noir Coffee Shop wants some market research on its customers. When a customer places an order, a clerk asks for the customer’s zip code and age. The clerk enters that data as well as the number of items the customer orders. The program operates continuously until the clerk enters a 0 for zip code at the end of the day. When the clerk enters an invalid zip code (more than 5 digits) or an invalid age (defined as less than 10 or more than 110), the program reprompts the clerk continuously. When the clerk enters fewer than 1 or more than 12 items, the program reprompts the clerk two more times. If the clerk enters a high value on the third attempt, the program accepts the high value, but if the clerk enters a negative value onthe third attempt, an error message is displayed and the order is not counted. At the end of the program, display a count of the number of items ordered by customers from the same zip code as the coffee shop (54984), and a count from other zip codes. Also…arrow_forwardP7arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
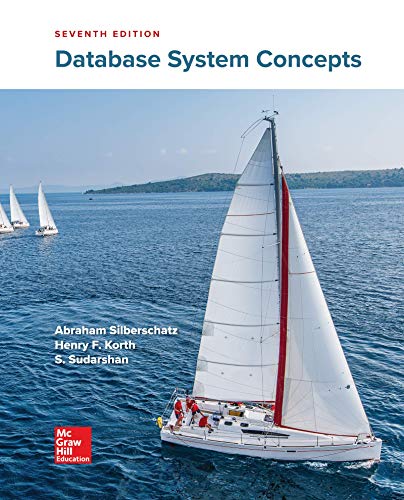
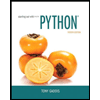
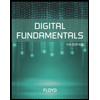
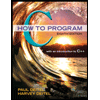
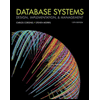
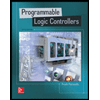