import java.util.Scanner; public class StudentScores { public static void main (String [] args) { Scanner scnr = new Scanner(System.in); final int SCORES_SIZE = 4; int[] oldScores = new int[SCORES_SIZE]; int[] newScores = new int[SCORES_SIZE]; int i; for (i = 0; i < oldScores.length; ++i) { oldScores[i] = scnr.nextInt(); } /* Your solution goes here */for (i=0; i < oldScores.length; i++) { newScores[i]= oldScores[i]; } // student code ends here for (i = 0; i < newScores.length; ++i) { System.out.print(newScores[i] + " "); } System.out.println(); } }
import java.util.Scanner; public class StudentScores { public static void main (String [] args) { Scanner scnr = new Scanner(System.in); final int SCORES_SIZE = 4; int[] oldScores = new int[SCORES_SIZE]; int[] newScores = new int[SCORES_SIZE]; int i; for (i = 0; i < oldScores.length; ++i) { oldScores[i] = scnr.nextInt(); } /* Your solution goes here */for (i=0; i < oldScores.length; i++) { newScores[i]= oldScores[i]; } // student code ends here for (i = 0; i < newScores.length; ++i) { System.out.print(newScores[i] + " "); } System.out.println(); } }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
My issue:
I don't know how to shift left after swapping arrays.
My code:
import java.util.Scanner;
public class StudentScores {
public static void main (String [] args) {
Scanner scnr = new Scanner(System.in);
final int SCORES_SIZE = 4;
int[] oldScores = new int[SCORES_SIZE];
int[] newScores = new int[SCORES_SIZE];
int i;
for (i = 0; i < oldScores.length; ++i) {
oldScores[i] = scnr.nextInt();
}
/* Your solution goes here */for (i=0; i < oldScores.length; i++) {
newScores[i]= oldScores[i];
}
// student code ends here
for (i = 0; i < newScores.length; ++i) {
System.out.print(newScores[i] + " ");
}
System.out.println();
}
}
![CHALLENGE
7.7.2: Copy and modify array elements.
ACTIVITY
Write a loop that sets newScores to oldScores shifted once left, with element 0 copied to the
end. Ex: If oldScores = {10, 20, 30, 40}, then newScores = {20, 30, 40, 10}.
Note: These activities may test code with different test values. This activity will perform two
tests, both with a 4-element array (int oldScores[4]). See "How to Use zyBooks".
Also note: If the submitted code tries to access an invalid array element, such as newScores[9]
for a 4-element array, the test may generate strange results. Or the test may crash and report
"Program end never reached", in which case the system doesn't print the test case that caused
the reported message.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc14768d3-3359-407d-85b4-624ecac227c9%2Fe98beab2-a62b-4013-9c85-8f5ac735cd9d%2Fnb90hnl_processed.png&w=3840&q=75)
Transcribed Image Text:CHALLENGE
7.7.2: Copy and modify array elements.
ACTIVITY
Write a loop that sets newScores to oldScores shifted once left, with element 0 copied to the
end. Ex: If oldScores = {10, 20, 30, 40}, then newScores = {20, 30, 40, 10}.
Note: These activities may test code with different test values. This activity will perform two
tests, both with a 4-element array (int oldScores[4]). See "How to Use zyBooks".
Also note: If the submitted code tries to access an invalid array element, such as newScores[9]
for a 4-element array, the test may generate strange results. Or the test may crash and report
"Program end never reached", in which case the system doesn't print the test case that caused
the reported message.
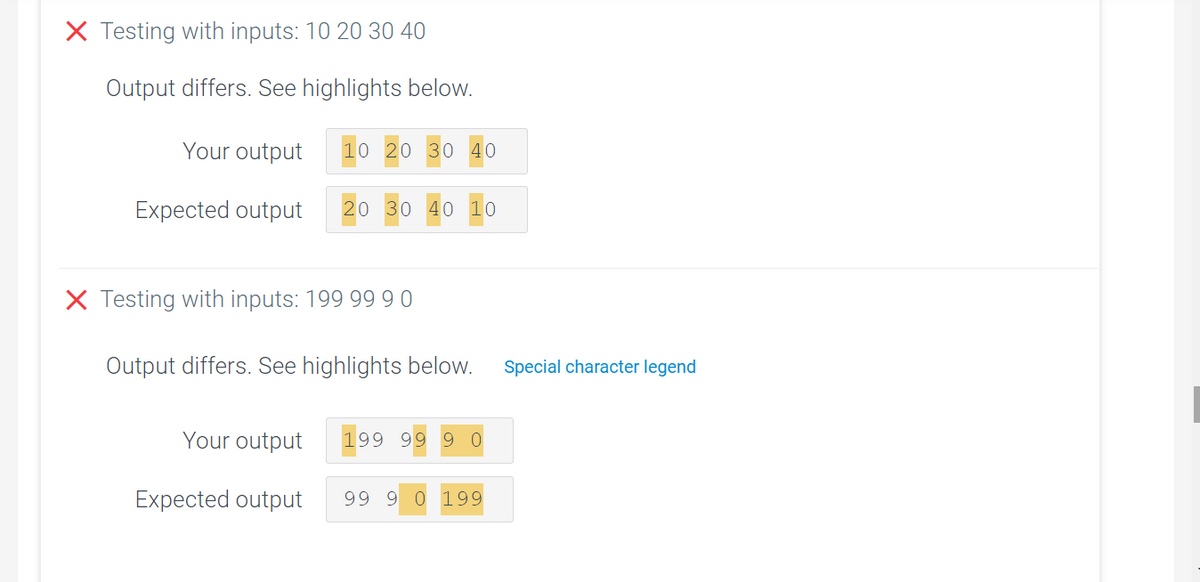
Transcribed Image Text:X Testing with inputs: 10 20 30 40
Output differs. See highlights below.
Your output
10 20 30 40
Expected output
20 30 40 10
X Testing with inputs: 199 9990
Output differs. See highlights below.
Special character legend
Your output
199 99 9
Expected output
99 9 0 199
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
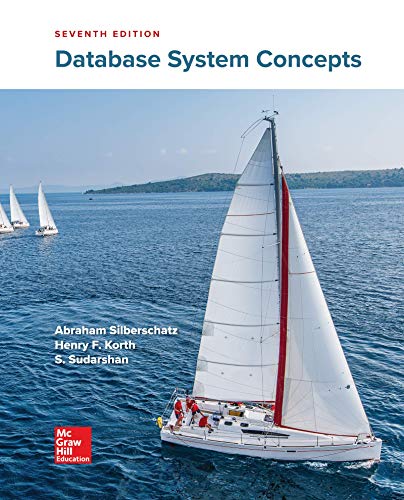
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
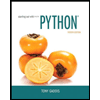
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
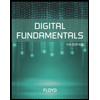
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
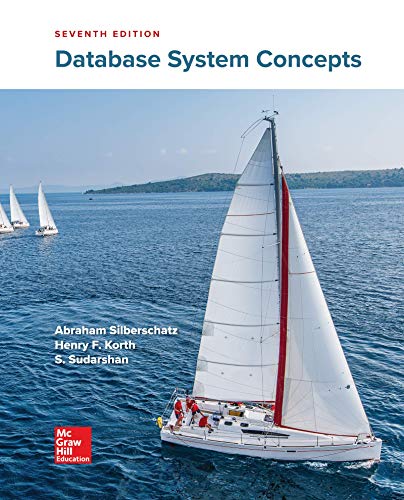
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
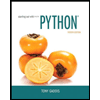
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
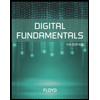
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
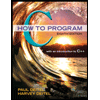
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
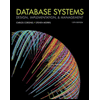
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
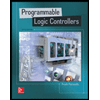
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education