In C++ /** * Performs an index-based bubble sort on any indexable container object. * * @tparam IndexedContainer must support `operator[]` and `size()`, e.g. * `std::vector`. Container * elements must support `operator<` and `operator=`. * * @param values the object to sort */ template void bubble_sort(IndexedContainer &values){ //TODO } /** * Sorts the elements in the range `[first,last)` into ascending order, using * the bubble-sort * algorithm. The elements are compared using `operator<`. * * @tparam Iterator a position iterator that supports the [standard * bidirectional iterator * operations](http://www.cplusplus.com/reference/iterator/BidirectionalIterator/) * * @param first the initial position in the sequence to be sorted * @param last one element past the final position in the sequence to be sorted */ template void bubble_sort(Iterator first, Iterator last) { //TODO }
In C++ /** * Performs an index-based bubble sort on any indexable container object. * * @tparam IndexedContainer must support `operator[]` and `size()`, e.g. * `std::vector`. Container * elements must support `operator<` and `operator=`. * * @param values the object to sort */ template void bubble_sort(IndexedContainer &values){ //TODO } /** * Sorts the elements in the range `[first,last)` into ascending order, using * the bubble-sort * algorithm. The elements are compared using `operator<`. * * @tparam Iterator a position iterator that supports the [standard * bidirectional iterator * operations](http://www.cplusplus.com/reference/iterator/BidirectionalIterator/) * * @param first the initial position in the sequence to be sorted * @param last one element past the final position in the sequence to be sorted */ template void bubble_sort(Iterator first, Iterator last) { //TODO }
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
In C++
/**
* Performs an index-based bubble sort on any indexable container object.
*
* @tparam IndexedContainer must support `operator[]` and `size()`, e.g.
* `std::vector `. Container
* elements must support `operator<` and `operator=`.
*
* @param values the object to sort
*/
template <typename IndexedContainer>
void bubble_sort(IndexedContainer &values){
//TODO
}
/**
* Sorts the elements in the range `[first,last)` into ascending order, using
* the bubble-sort
* algorithm . The elements are compared using `operator<`.
*
* @tparam Iterator a position iterator that supports the [standard
* bidirectional iterator
* operations](http://www.cplusplus.com/reference/iterator/BidirectionalIterator/)
*
* @param first the initial position in the sequence to be sorted
* @param last one element past the final position in the sequence to be sorted
*/
template <typename Iterator>
void bubble_sort(Iterator first, Iterator last) {
//TODO
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
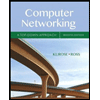
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
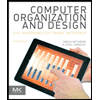
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
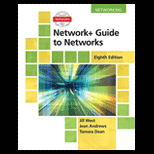
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
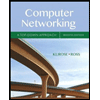
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
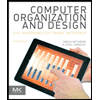
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
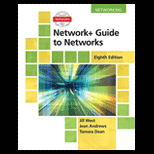
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
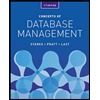
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
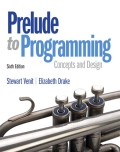
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
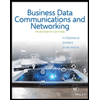
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY