4. Recursively Multiply (20 points) Write a recursive function that multiplies its two integer arguments (x and y) recursively. The function should return the value of x times y. Hint: multiplication can be performed as repeated addition, e.g.: 7x4=4+4+4+ 4 +4+4 +4 = 28
4. Recursively Multiply (20 points) Write a recursive function that multiplies its two integer arguments (x and y) recursively. The function should return the value of x times y. Hint: multiplication can be performed as repeated addition, e.g.: 7x4=4+4+4+ 4 +4+4 +4 = 28
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter15: Recursion
Section: Chapter Questions
Problem 1TF
Related questions
Question
Python
Test:
import recursive_functions
import math
def main():
# Test factorial
print('Testing factorial.')
assert recursive_functions.factorial(0) == 1
assert recursive_functions.factorial(1) == math.factorial(1)
== 1
assert recursive_functions.factorial(2) == math.factorial(2)
== 2
assert recursive_functions.factorial(5) == math.factorial(5)
== 120
assert recursive_functions.factorial(7) == math.factorial(7)
== 5040
print('All tests pass for `factorial` ()\n')
# Test sum_recursively
print('Testing sum_recursively.')
assert recursive_functions.sum_recursively(0) == 0
assert recursive_functions.sum_recursively(1) ==
sum(range(1+1)) == 1
assert recursive_functions.sum_recursively(2) ==
sum(range(2+1)) == 3
assert recursive_functions.sum_recursively(10) ==
sum(range(10+1)) == 55
print('All tests pass for `sum_recursively` () ')
# Test sumlist_recursively(l)
print('Testing sumlist_recursively.')
assert recursive_functions.sumlist_recursively([1,2,3]) ==
sum([1,2,3])
assert
recursive_functions.sumlist_recursively([42,16,99,102,1]) ==
sum([42,16,99,102,1])
assert recursive_functions.sumlist_recursively([17,13,9,5,1])
== sum([17,13,9,5,1])
print('All tests pass for r_sumlist ()\n')
# Test multiply_recursively
print('Testing multiply_recursively.')
assert recursive_functions.multiply_recursively(5, 1) == 5*1
== 5
assert recursive_functions.multiply_recursively(7, 4) == 7*4
== 28
import math
def main():
# Test factorial
print('Testing factorial.')
assert recursive_functions.factorial(0) == 1
assert recursive_functions.factorial(1) == math.factorial(1)
== 1
assert recursive_functions.factorial(2) == math.factorial(2)
== 2
assert recursive_functions.factorial(5) == math.factorial(5)
== 120
assert recursive_functions.factorial(7) == math.factorial(7)
== 5040
print('All tests pass for `factorial` ()\n')
# Test sum_recursively
print('Testing sum_recursively.')
assert recursive_functions.sum_recursively(0) == 0
assert recursive_functions.sum_recursively(1) ==
sum(range(1+1)) == 1
assert recursive_functions.sum_recursively(2) ==
sum(range(2+1)) == 3
assert recursive_functions.sum_recursively(10) ==
sum(range(10+1)) == 55
print('All tests pass for `sum_recursively` () ')
# Test sumlist_recursively(l)
print('Testing sumlist_recursively.')
assert recursive_functions.sumlist_recursively([1,2,3]) ==
sum([1,2,3])
assert
recursive_functions.sumlist_recursively([42,16,99,102,1]) ==
sum([42,16,99,102,1])
assert recursive_functions.sumlist_recursively([17,13,9,5,1])
== sum([17,13,9,5,1])
print('All tests pass for r_sumlist ()\n')
# Test multiply_recursively
print('Testing multiply_recursively.')
assert recursive_functions.multiply_recursively(5, 1) == 5*1
== 5
assert recursive_functions.multiply_recursively(7, 4) == 7*4
== 28
print('All tests pass for `multiply_recursively` ()\n')
# Test reverse_recursively
print('Testing reverse_recursively.')
assert recursive_functions.reverse_recursively([1, 2, 3, 4])
== [4, 3, 2, 1]
life = ['born', 'grow up', 'grow old']
assert recursive_functions.reverse_recursively(life) == ['grow
old', 'grow up', 'born']
print('All tests pass for `reverse_recursively` ()\n')
main()
# Test reverse_recursively
print('Testing reverse_recursively.')
assert recursive_functions.reverse_recursively([1, 2, 3, 4])
== [4, 3, 2, 1]
life = ['born', 'grow up', 'grow old']
assert recursive_functions.reverse_recursively(life) == ['grow
old', 'grow up', 'born']
print('All tests pass for `reverse_recursively` ()\n')
main()
![4. Recursively Multiply (20 points)
Write a recursive function that multiplies its two integer arguments (x and y)
recursively. The function should retum the value of x times y. Hint: multiplication can
be performed as repeated addition, e.g.:
7x4 = 4 + 4 +4+ 4 + 4 +4 + 4 = 28
5. Recursively Reverse a List (20 points)
Write a recursive function that reverses a list. For example, given [1, 2, 3, 4], the function
would return this list [4, 3, 2, 1]. It does not print anything.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fb97d67e1-dda9-48ae-b6f6-b8910f9a7c16%2F7ff0f1c0-4d95-4972-a07e-ea70df6f2db6%2Fsoc0v1v_processed.jpeg&w=3840&q=75)
Transcribed Image Text:4. Recursively Multiply (20 points)
Write a recursive function that multiplies its two integer arguments (x and y)
recursively. The function should retum the value of x times y. Hint: multiplication can
be performed as repeated addition, e.g.:
7x4 = 4 + 4 +4+ 4 + 4 +4 + 4 = 28
5. Recursively Reverse a List (20 points)
Write a recursive function that reverses a list. For example, given [1, 2, 3, 4], the function
would return this list [4, 3, 2, 1]. It does not print anything.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
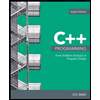
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
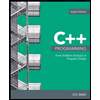
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning