1. Implement a recursive function called firstTwo that returns the sum of the firsttwo digits in a positive number. If there is only one digit, that digit is returned.Note this function is returning an integer, not printing. There should be no use ofcout within your function.Title line: int firstTwo(int n)ex. firstTwo(8325) returns 11ex. cout << firstTwo(428); // prints 62. Implement a function called findMinGap that will return the smallest gap betweenadjacent entries of an integer array. A gap between two numbers is the absolutevalue of their difference. For example, if an array contains the elements {10, 14,-5, -3, 0, 5, 7}, the minimum gap is 2 (between -5 and -3).Title line: int findMinGap(int array[], int length)3. Implement a function called findLargestIndex which returns the index of the rowwith the largest sum.ex. int array[3][6] = {{3, 6, 8, 2, 4, 1}, // sum = 24{2, 4, 5, 1, 3, 4}, // sum = 19{1, 0, 9, 0, 1, 0}}; // sum = 11If the findLargestIndex function was called using this array, it would return 0, asit is returning the index of the row, not the sum.Note that the number of columns is required when passing a 2d array to a function.Assume that 2D arrays passed to this function will have 6 columns.Title line: int findLargestIndex(int array[][6], int rows, int columns);You may use the following main function to test your code:int main() {cout << firstTwo(8325) << endl;int array[3][6] = {{3, 6, 8, 2, 4, 1},{2, 4, 5, 1, 3, 4},{1, 0, 9, 0, 1, 0}};cout << findLargestIndex(array, 3, 6) << endl;int a2[] = {10, 14, -5, -3, 0, 5, 7};
1. Implement a recursive function called firstTwo that returns the sum of the first
two digits in a positive number. If there is only one digit, that digit is returned.
Note this function is returning an integer, not printing. There should be no use of
cout within your function.
Title line: int firstTwo(int n)
ex. firstTwo(8325) returns 11
ex. cout << firstTwo(428); // prints 6
2. Implement a function called findMinGap that will return the smallest gap between
adjacent entries of an integer array. A gap between two numbers is the absolute
value of their difference. For example, if an array contains the elements {10, 14,
-5, -3, 0, 5, 7}, the minimum gap is 2 (between -5 and -3).
Title line: int findMinGap(int array[], int length)
3. Implement a function called findLargestIndex which returns the index of the row
with the largest sum.
ex. int array[3][6] = {{3, 6, 8, 2, 4, 1}, // sum = 24
{2, 4, 5, 1, 3, 4}, // sum = 19
{1, 0, 9, 0, 1, 0}}; // sum = 11
If the findLargestIndex function was called using this array, it would return 0, as
it is returning the index of the row, not the sum.
Note that the number of columns is required when passing a 2d array to a function.
Assume that 2D arrays passed to this function will have 6 columns.
Title line: int findLargestIndex(int array[][6], int rows, int columns);
You may use the following main function to test your code:
int main() {
cout << firstTwo(8325) << endl;
int array[3][6] = {{3, 6, 8, 2, 4, 1},
{2, 4, 5, 1, 3, 4},
{1, 0, 9, 0, 1, 0}};
cout << findLargestIndex(array, 3, 6) << endl;
int a2[] = {10, 14, -5, -3, 0, 5, 7};

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

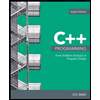
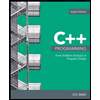