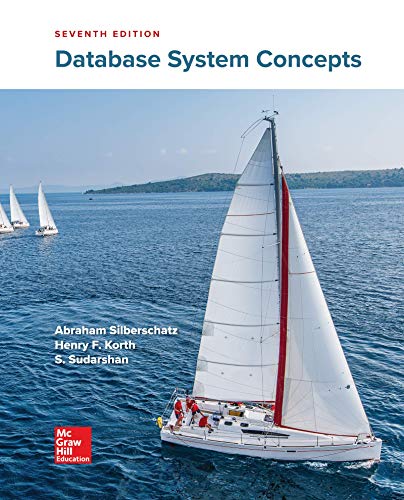
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
![Write a recursive function that returns True if the numerical array passed as input is palindrome,
and False otherwise. An array is palidrome if its elements, when flipped, produce an array that is
identical to the original one. For example:
[1,2,3,2,1] is palindrome
[1,2,3,4,3] is not palindrome
Fill in the following code skeleton. It is composed of four parts: three base cases and the recursive
call. Write code for all the parts. Assume that the input arrays can have any size, and that the
elements are always positive integers.](https://content.bartleby.com/qna-images/question/d8694f02-79fe-4b76-9079-b1456b2e1bd2/35796166-0a03-46f7-8f2d-855a4cd293b3/nxnjfn9_thumbnail.png)
Transcribed Image Text:Write a recursive function that returns True if the numerical array passed as input is palindrome,
and False otherwise. An array is palidrome if its elements, when flipped, produce an array that is
identical to the original one. For example:
[1,2,3,2,1] is palindrome
[1,2,3,4,3] is not palindrome
Fill in the following code skeleton. It is composed of four parts: three base cases and the recursive
call. Write code for all the parts. Assume that the input arrays can have any size, and that the
elements are always positive integers.
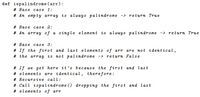
Transcribed Image Text:def ispalindrome (arr):
# Base case 1:
# An empty array is always palindrome -> return True
# Base case 2:
# An arraY of a single element is always palindrome -> return True
# Base case 3:
# If the f irst and last elements of arr are not identical,
# the array is not palindrome -> return False
# If we get here it 's because the first and last
# elements are identical, therefore:
# Recursive call:
# Call ispalindrome () dropping the first and last
# elements of arr
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Digital Sum The digital sum of a number n is the sum of its digits. Write a recursive function digitalSum(n:int) -> int that takes a positive integer n and returns its digital sum. For example, digitalSum (2019) should return 12 because 2+0+1+9=12. Your Answer: 1 # Put your answer here 2 Submitarrow_forwardWrite a recursive function for printing n box shapes [ ] in a row. Hint: Print one [], then print n - 1 of them. Complete the following file: boxes.cpp 1 #include 2 using namespace std; 3 4 void print_boxes(int n) 5 { 6. if (. . .) { . cout << ; } 7 8 print_boxes(. . .);| 9 }arrow_forwardUse C++arrow_forward
- in C programing Write a recursive function that returns 1 if an array of size n is in sorted order and 0 otherwise. Note: If array a stores 3, 6, 7, 7, 12, then isSorted(a, 5) should return 1 . If array b stores 3, 4, 9, 8, then isSorted(b,4) should return 0.int isSorted(int *array, int n){arrow_forwardWrite a recursive function that displays a string reversely on the console using the following header: def reverseDisplay(value):For example, reverseDisplay("abcd") displays dcba. Write a test programthat prompts the user to enter a string and displays its reversal.arrow_forwardWrite a recursive function that parses a hex number as a string into a decimal integer. The function header is as follows:def hexToDecimal(hexString):Write a test program that prompts the user to enter a hex string and displays its decimal equivalent.arrow_forward
- Finish the splitOdd10 using recursion to determine if the elements of an array can be split into two groups such that the sum of one group is a non-zero multiple of 10 and the sum of the other group is oddarrow_forward1. Given, nCr= n! (n-r)!r!" Write a function to compute nCr without recursion.arrow_forwardDesign and implement a recursive program(in java) to determine and print the Nth line of Pascal's triangle, as shown below. Each interior value is the sum of the two values above it. Hint: Use an array to store the values on each line.arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
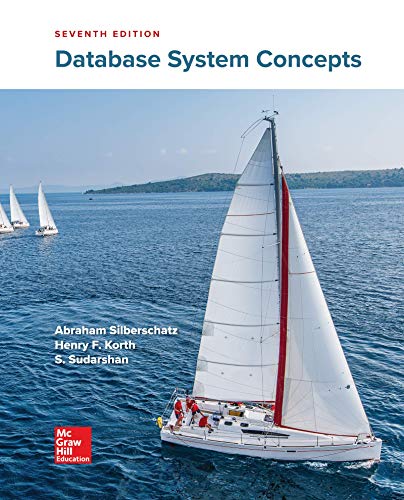
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
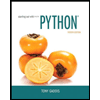
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
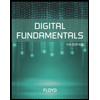
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
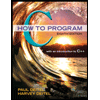
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
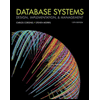
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
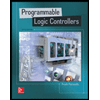
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education