A company pays its workers on a weekly basis. The workers are of 4 types: FixedWeekly workers are paid a fixed amount regardless of the number of hours worked ByTheHour workers are paid by the hour and receive overtime pay for all hours worked in excess of 40 PercentOfSales workers are paid a percentage of their sales FixedWeeklyPercentOfSales workers receive a fixed amount plus a percentage of their sales. Create a class called Worker. This class will represent the general concept of a worker. All 4 types of workers are considered Workers. FixedWeeklyPercentOfSales workers are considered to be PercentOfSales Workers. The Worker class will be Comparable. Comparable is an interface in the Java API. You will base your comparison on their first name concatenated onto their last names. The Worker class will also be Payable. You will need to create the Payable interface. It will contain just one method called earnings(). Two Workers will be considered equal if their earnings are the same. A Worker has a first name, last name, and social security number. A FixedWeekly worker has a first name, last name, social security number and fixed weekly salary. A ByTheHour worker has a first name, last name, social security number, an hourly wage, and number of hours worked. A PercentOfSales worker has a first name, last name, social security number, gross sales amount, and commission rate. A FixedWeeklyPercentOfSales worker has a first name, last name, social security number, gross sales amount, commission rate and a fixed weekly salary. The Worker class will contain an appropriate 3 parameter constructor and a default constructor, appropriate accessors and mutators, toString, equals, and compareTo methods. It will also contain an earnings method which must be implemented by the subclasses. The constructor will validate that the social security number is in the proper format (999-99-9999). If not in the proper format, the constructor will assign XXX-XX-XXXX for the social security number. (you can do this using appropriate methods of the String class or by using what are called Regular Expressions…it might be easier to just use methods from the String class rather than learning about Regular Expressions The FixedWeekly class will contain an appropriate 4 parameter constructor and a default constructor, appropriate accessors and mutators, earnings, toString, equals, and compareTo methods. The constructor will validate that the fixed weekly salary is not negative. If the salary figure is negative the fixed weekly salary value will be set to 0. The ByTheHour class will contain an appropriate 5 parameter constructor and a default constructor, The PercentOfSales class will contain an appropriate 5 parameter constructor and a default constructor, appropriate accessors and mutators, earnings, toString, equals, and compareTo methods. The constructor will validate that the gross sales amount is not negative and that the commission rate is a value between 0.0 and 1.0 inclusive. If these conditions are not met then set the appropriate variable values to 0. The FixedWeeklyPercentOfSales class will contain an appropriate 6 parameter constructor and a default constructor, appropriate accessors and mutators, earnings, toString, equals, and compareTo methods. The constructor will validate that the fixed weekly salary amount is not negative. If the fixed weekly salary is negative set the appropriate variable value to 0. Write a class to test your inheritance hierarchy. In that class do the following: Create 4 Worker objects. Use the below information The Test Data is in a File called workers.txt and contains the following data: fixed Harry Clark 111-11-1111 800.00 hour Katie Brown 222-22-2222 16.75 40 percent Joan White, 333-33-3333 10000 .06 fxdpercent Bob Green 444-44-4444 5000 .04 300 The first token in each line in the data file represents a code for the type of Worker fixed – FixedWeekly hour – ByTheHour percent – PercentOfSales fxdpercent - FixedWeeklyPercentOfSales Reading the data file (Suggested approach…..if you use a different approach make sure you thoroughly document your approach) store each line in an array of Strings Tokenize (split) the string Instantiate the correct type of Worker object based on a worker code store the object in an array of Workers so that you can access them polymorphically. Process these objects polymorphically displaying a string representation of each Worker and their earnings as shown at the end of this document (i.e. loop through your array of Workers, this could have been a file that contained thousands of Workers) You will need to perform special processing on FixedWeeklyPercentOfSales objects in that we have decided to give them a 10% permanent increase to their FixedWeekly salary. Display each worker and their class type as shown at the end of this document.
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
A company pays its workers on a weekly basis. The workers are of 4 types:
- FixedWeekly workers are paid a fixed amount regardless of the number of hours worked
- ByTheHour workers are paid by the hour and receive overtime pay for all hours worked in excess of 40
- PercentOfSales workers are paid a percentage of their sales
- FixedWeeklyPercentOfSales workers receive a fixed amount plus a percentage of their sales.
Create a class called Worker. This class will represent the general concept of a worker. All 4 types of workers are considered Workers. FixedWeeklyPercentOfSales workers are considered to be PercentOfSales Workers. The Worker class will be Comparable. Comparable is an interface in the Java API. You will base your comparison on their first name concatenated onto their last names. The Worker class will also be Payable. You will need to create the Payable interface. It will contain just one method called earnings(). Two Workers will be considered equal if their earnings are the same.
A Worker has a first name, last name, and social security number. A FixedWeekly worker has a first name, last name, social security number and fixed weekly salary. A ByTheHour worker has a first name, last name, social security number, an hourly wage, and number of hours worked. A PercentOfSales worker has a first name, last name, social security number, gross sales amount, and commission rate. A FixedWeeklyPercentOfSales worker has a first name, last name, social security number, gross sales amount, commission rate and a fixed weekly salary.
The Worker class will contain an appropriate 3 parameter constructor and a default constructor, appropriate accessors and mutators, toString, equals, and compareTo methods. It will also contain an earnings method which must be implemented by the subclasses. The constructor will validate that the social security number is in the proper format (999-99-9999). If not in the proper format, the constructor will assign XXX-XX-XXXX for the social security number. (you can do this using appropriate methods of the String class or by using what are called Regular Expressions…it might be easier to just use methods from the String class rather than learning about Regular Expressions
The FixedWeekly class will contain an appropriate 4 parameter constructor and a default constructor, appropriate accessors and mutators, earnings, toString, equals, and compareTo methods. The constructor will validate that the fixed weekly salary is not negative. If the salary figure is negative the fixed weekly salary value will be set to 0.
The ByTheHour class will contain an appropriate 5 parameter constructor and a default constructor,
The PercentOfSales class will contain an appropriate 5 parameter constructor and a default constructor, appropriate accessors and mutators, earnings, toString, equals, and compareTo methods. The constructor will validate that the gross sales amount is not negative and that the commission rate is a value between 0.0 and 1.0 inclusive. If these conditions are not met then set the appropriate variable values to 0.
The FixedWeeklyPercentOfSales class will contain an appropriate 6 parameter constructor and a default constructor, appropriate accessors and mutators, earnings, toString, equals, and compareTo methods.
The constructor will validate that the fixed weekly salary amount is not negative. If the fixed weekly salary is negative set the appropriate variable value to 0.
Write a class to test your inheritance hierarchy. In that class do the following:
- Create 4 Worker objects. Use the below information
- The Test Data is in a File called workers.txt and contains the following data:
fixed Harry Clark 111-11-1111 800.00
hour Katie Brown 222-22-2222 16.75 40
percent Joan White, 333-33-3333 10000 .06
fxdpercent Bob Green 444-44-4444 5000 .04 300
The first token in each line in the data file represents a code for the type of Worker
- fixed – FixedWeekly
- hour – ByTheHour
- percent – PercentOfSales
- fxdpercent - FixedWeeklyPercentOfSales
- Reading the data file (Suggested approach…..if you use a different approach make sure you thoroughly document your approach)
- store each line in an array of Strings
- Tokenize (split) the string
- Instantiate the correct type of Worker object based on a worker code
- store the object in an array of Workers so that you can access them polymorphically.
- Process these objects polymorphically displaying a string representation of each Worker and their earnings as shown at the end of this document (i.e. loop through your array of Workers, this could have been a file that contained thousands of Workers)
- You will need to perform special processing on FixedWeeklyPercentOfSales objects in that we have decided to give them a 10% permanent increase to their FixedWeekly salary.
- Display each worker and their class type as shown at the end of this document.

Trending now
This is a popular solution!
Step by step
Solved in 8 steps with 1 images

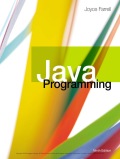
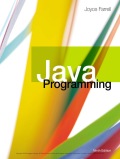