Create an Inventory class named, Inventory.cpp, that can hold information and calculate data for items in a retail store’s inventory. The class should have the following private member variables: Variable Name Description itemNumber An integer that holds the item’s item number. quantity An integer for holding the quantity of the items on hand. cost A double for holding the wholesale per-unit cost of the item totalCost A double for holding the total inventory cost of the item (calculated as quantity times cost). The class should have the following public member functions: Member Function Description Default Constructor Sets all the member variables to 0. Constructor #2 Accepts an item’s number, cost, and quantity as arguments. The function should copy these values to the appropriate member variables. setItemNumber Accepts an integer argument that is copied to the itemNumber member variable. setQuantity Accepts an integer argument that is copied to the quantity member variable. setCost Accepts a double argument that is copied to the cost member variable. setTotalCost Calculates the total inventory cost for the item (quantity times cost) and stores the result in totalCost. getItemNumber Returns the value in itemNumber. getQuantity Returns the value in quantity. getCost Returns the value in cost. getTotalCost Returns the value in totalCost. Test the class with the main program, Program7.cpp, provided. You are allowed to modify only line 149. If you modify any other part of the program, you will not receive credit for Program 7. Do not accept negative values for item number, quantity, or cost.
Create an Inventory class named, Inventory.cpp, that can hold information and calculate
data for items in a retail store’s inventory.
The class should have the following private member variables:
Variable Name Description
itemNumber An integer that holds the item’s item number.
quantity An integer for holding the quantity of the items on hand.
cost A double for holding the wholesale per-unit cost of the item
totalCost A double for holding the total inventory cost of the item
(calculated as quantity times cost).
The class should have the following public member functions:
Member Function Description
Default Constructor Sets all the member variables to 0.
Constructor #2 Accepts an item’s number, cost, and quantity as arguments.
The function should copy these values to the appropriate
member variables.
setItemNumber Accepts an integer argument that is copied to the
itemNumber member variable.
setQuantity Accepts an integer argument that is copied to the quantity
member variable.
setCost Accepts a double argument that is copied to the cost
member variable.
setTotalCost Calculates the total inventory cost for the item (quantity
times cost) and stores the result in totalCost.
getItemNumber Returns the value in itemNumber.
getQuantity Returns the value in quantity.
getCost Returns the value in cost.
getTotalCost Returns the value in totalCost.
Test the class with the main
Do not accept negative values for item number, quantity, or cost.
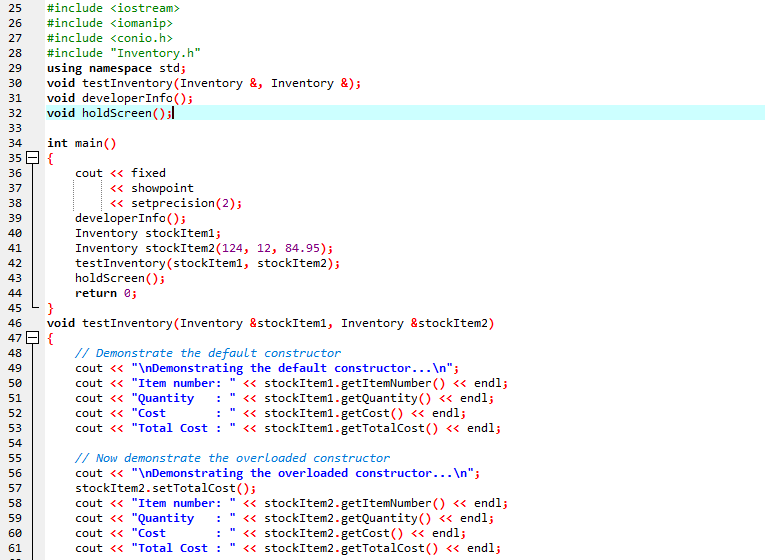
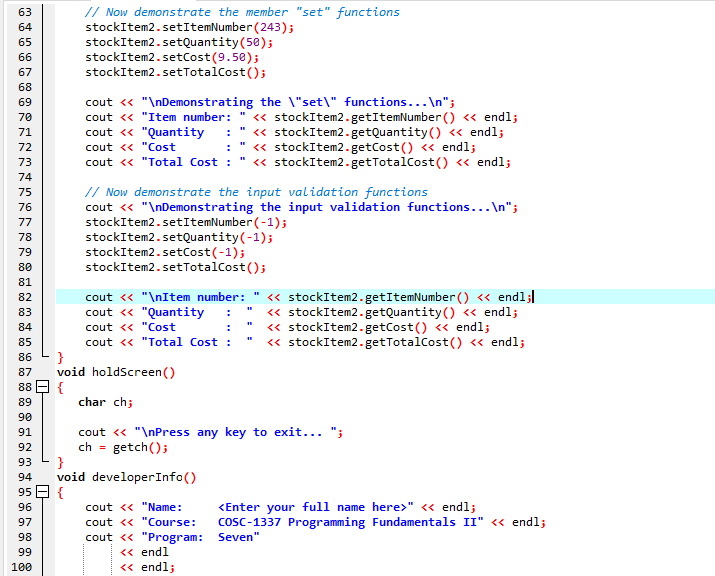

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

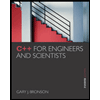
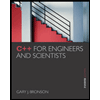