(a) Write a function named getShortestPath (graph, from, to) that takes a graph as an input and estimates the shortest distance between a node "from" to a node "to". Example 1: Input Graph: Adjacency list of the following graph. 5 A B 3 10 E D 9. 8. 2 F G 10 From: A To: G Output [(А, В), (В, С), (С, G)] That is, the output shows that the shortest path to reach "G" from "A" is through the nodes B and C.
(a) Write a function named getShortestPath (graph, from, to) that takes a graph as an input and estimates the shortest distance between a node "from" to a node "to". Example 1: Input Graph: Adjacency list of the following graph. 5 A B 3 10 E D 9. 8. 2 F G 10 From: A To: G Output [(А, В), (В, С), (С, G)] That is, the output shows that the shortest path to reach "G" from "A" is through the nodes B and C.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Python 3 code required. Need solutions for part (a) and (b)
Please do not use classes!
![Exercise # 1: Dijkstra’s Algorithm
(a) Write a function named getShortestPath (graph, from, to) that takes a graph as
an input and estimates the shortest distance between a node "from" to a node
“to".
Example 1:
Input
Graph: Adjacency list of the following graph.
6.
6.
10
E
8
2
F
10
From: A
To: G
Output
[(A, B), (B, C), (C, G)]
That is, the output shows that the shortest path to reach “G" from "A"
is through the nodes B and C.
(b)You are given a CSV file that contains a list of different cities in northern areas of Pakistan
and their distances. Not all of the cities are directly connected which is represented by
-1 in the distance matrix.
i.Load the given dataset in the form of a graph (adjacency list)
ii.Estimate the shortest distance between Islamabad and Kaghan.
Csv file:
Islamabad Taxila Murree Nathiagali Abbottabad Mansehra Balakot Kaghan Naran Chilas
Bisham Gilgit Hunza Khunjerab Pass Malam Jabba Skardu Muzaff
Islamabad
46
49
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
Taxila
46
-1
-1
74
-1
-1
-1
-1
-1
-1
-1
-1
-1
254
-1
-1
Murree
49
-1
36
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
68
Nathiagali
36
34
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
Abbottabad
-1
74
-1
34
23
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
Mansehra
-1
-1
-1
-1
23
37
-1
-1
-1
113
-1
-1
-1
-1
-1
-1
Balakot
-1
-1
-1
-1
-1
37
59
-1
-1
136
-1
-1
-1
-1
-1
-1
Kaghan
-1
-1
-1
-1
-1
-1
59
22
-1
-1
-1
-1
-1
-1
-1
-1
Naran
-1
-1
-1
-1
-1
-1
-1
22
113
-1
-1
-1
-1
-1
-1
-1
Chilas
-1
-1
-1
-1
-1
-1
-1
-1
113
199
133
306
-1
-1
-1
-1
Bisham
-1
-1
-1
-1
-1
113
136
-1
-1
199
-1
-1
-1
61
-1
-1
Gilgit
-1
-1
-1
-1
-1
-1
-1
-1
-1
133
-1
186
-1
-1
208
-1
Hunza
-1
-1
-1
-1
-1
-1
-1
-1
-1
306
-1
186
151
-1
381
-1
Khunjerab Pass -1
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
151
-1
-1
-1
Malam Jabba
-1
254
-1
-1
-1
-1
-1
-1
-1
-1
61
-1
-1
-1
-1
-1
Skardu
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
208
381
-1
-1
484
Muzaffarabad
-1
-1
68
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
484](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F7ee2210f-6539-44d4-8e38-60565143d3fa%2F78de0d97-0e72-4014-b4de-bcb6b26e0c60%2Fmlwspkm_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Exercise # 1: Dijkstra’s Algorithm
(a) Write a function named getShortestPath (graph, from, to) that takes a graph as
an input and estimates the shortest distance between a node "from" to a node
“to".
Example 1:
Input
Graph: Adjacency list of the following graph.
6.
6.
10
E
8
2
F
10
From: A
To: G
Output
[(A, B), (B, C), (C, G)]
That is, the output shows that the shortest path to reach “G" from "A"
is through the nodes B and C.
(b)You are given a CSV file that contains a list of different cities in northern areas of Pakistan
and their distances. Not all of the cities are directly connected which is represented by
-1 in the distance matrix.
i.Load the given dataset in the form of a graph (adjacency list)
ii.Estimate the shortest distance between Islamabad and Kaghan.
Csv file:
Islamabad Taxila Murree Nathiagali Abbottabad Mansehra Balakot Kaghan Naran Chilas
Bisham Gilgit Hunza Khunjerab Pass Malam Jabba Skardu Muzaff
Islamabad
46
49
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
Taxila
46
-1
-1
74
-1
-1
-1
-1
-1
-1
-1
-1
-1
254
-1
-1
Murree
49
-1
36
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
68
Nathiagali
36
34
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
Abbottabad
-1
74
-1
34
23
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
Mansehra
-1
-1
-1
-1
23
37
-1
-1
-1
113
-1
-1
-1
-1
-1
-1
Balakot
-1
-1
-1
-1
-1
37
59
-1
-1
136
-1
-1
-1
-1
-1
-1
Kaghan
-1
-1
-1
-1
-1
-1
59
22
-1
-1
-1
-1
-1
-1
-1
-1
Naran
-1
-1
-1
-1
-1
-1
-1
22
113
-1
-1
-1
-1
-1
-1
-1
Chilas
-1
-1
-1
-1
-1
-1
-1
-1
113
199
133
306
-1
-1
-1
-1
Bisham
-1
-1
-1
-1
-1
113
136
-1
-1
199
-1
-1
-1
61
-1
-1
Gilgit
-1
-1
-1
-1
-1
-1
-1
-1
-1
133
-1
186
-1
-1
208
-1
Hunza
-1
-1
-1
-1
-1
-1
-1
-1
-1
306
-1
186
151
-1
381
-1
Khunjerab Pass -1
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
151
-1
-1
-1
Malam Jabba
-1
254
-1
-1
-1
-1
-1
-1
-1
-1
61
-1
-1
-1
-1
-1
Skardu
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
208
381
-1
-1
484
Muzaffarabad
-1
-1
68
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
-1
484
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
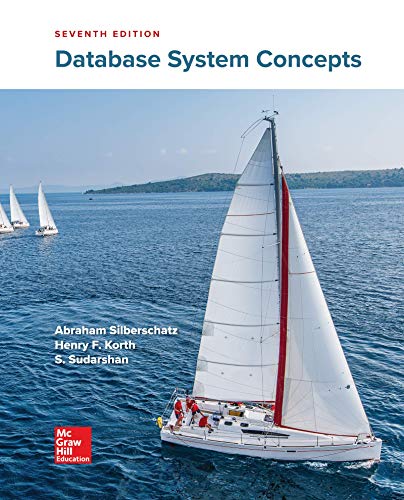
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
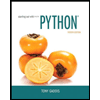
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
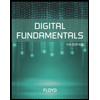
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
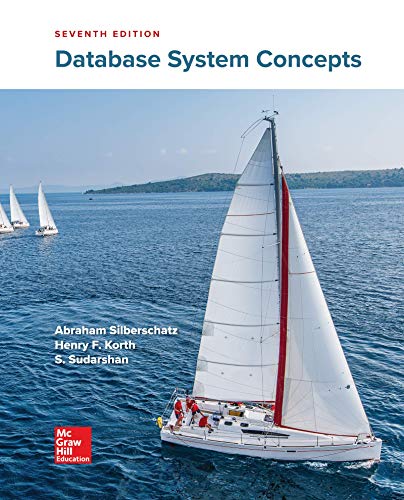
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
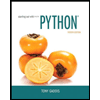
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
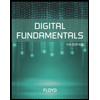
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
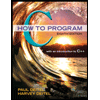
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
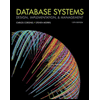
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
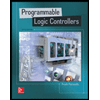
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education