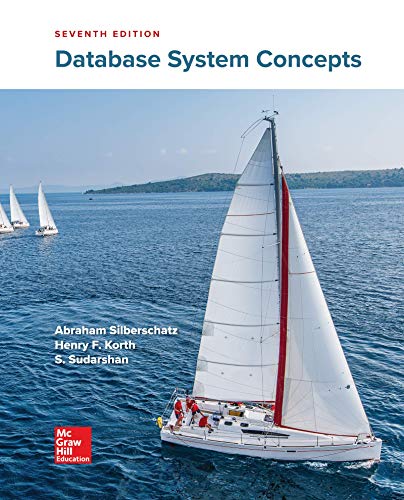
Concept explainers
I need help with coding C++ :
Write the definition of a class swimmingPool, to implement the properties of a swimming pool. Your class should have the instance variables to store the length (in feet), width (in feet), depth (in feet), the rate (in gallons per minute) at which the water is filling the pool, and the rate (in gallons per minute) at which the water is draining from the pool. Add appropriate constructors to initialize the instance variables. Also, add member functions to do the following: determine the amount of water needed to fill an empty or partially filled pool, determine the time needed to completely or partially fill or empty the pool, and add or drain water for a specific amount of time, if the water in the pool exceeds the total capacity of the pool, output "Pool overflow" to indicate that the water has breached capacity. The header file for the swimmingPool class has been provided for reference.
Write a program to test your swimmingPool class.
An example of the program is shown below:
Pool data: Length: 30 Width: 15 Depth: 10 Total water in the pool: 0 To completely fill the pool: Enter water fill-in rate: 10 Time to fill the pool is approximately: 56 hour(s) and 6 minute(s)
There are three tabs: main.cpp, swimmingPool.h, and swimmingPoollmp.cpp
swimmingPool.h:

Trending nowThis is a popular solution!
Step by stepSolved in 5 steps with 5 images

- Ro-Sham-Bo. Believe it or not, the classic game of Rock-PaperScissors has many other names. One of them is Ro-Sham-Bo. For this assignment, “Ro” will represent “Rock”, “Sham” will represent Paper, and “Bo” will represent Scissors. You will create a RoshamboPlayer class in C++. It will have three attributes: PlayerName: String RoLimit: int ShamBoLimit: int It will also have an overloaded constructor that sets those three values. It will have two functions: playRound that takes in a string and returns a boolean value, and getName that takes in nothing and returns the PlayerName string. In your driver class for this assignment, you will create two RoshamboPlayer objects with the following values: p1: RoLimit == 30, ShamBoLimit == 60 p2: RoLimit = 40, ShamBoLimit = 85 You may name them whatever you like. You will prompt the user to choose one of these two to play against. Then you will create a loop that prompt the user to either play a round of Roshambo, or quit the game. If the user…arrow_forwardFor C++ The following class represents a telephone number in the United States: enum PhoneType (HOME, OFFICE, CELL); class Phone { public: Phone(int newAreaCode, int newNumber, PhoneType newType); void Write() const; private: int areaCode; int number; PhoneType type; } We want to use inheritance to derive an international phone number class, called InternPhone, from the Phone class above. The InternPhone class needs to add a country code, represented as an integer, used to identify the country. The public operations the InternPhone class are responsible for are: Write() Reimplements the Write function from the base class. Paramaterized Constructor Requires four parameters. Write the class declaration for the InternPhone class. Provide an implementation for the InternPhone Provide an implementation for the InternPhone Write()arrow_forwardDesign, code in Java, test and document a base class, Student. The Student class willhave the following information, and all of these should be defined as Private:A. Title of the student (eg Mr, Miss, Ms, Mrs etc)B. A first name (given name)C. A last name (family name/surname)D. Student number (ID) – an integer number (of type long)E. A date of birth (in day/month/year format – three ints) - (Do NOT use the Date class fromJAVA)The student class will have at least the following constructors and methods:(i) two constructors - one without any parameters (the default constructor), and one withparameters to give initial values to all the instance variables.(ii) only necessary set and get methods for a valid class design.(iii) method to write the output of all the instance variables in the class to the screen (which willbe overridden in the respective child classes, as you have more instance variables thatrequired to be output).(iv) an equals method which compares two student objects and…arrow_forward
- Python:Please read the following. The Game class The Game class brings together an instance of the GameBoard class, a list of Player objects who are playing the game, and an integer variable “n” indicating how many tokens must be aligned to win (as in the name “Connect N”). The game class has a constructor and two additional methods. The most complicated of these, play, is provided for you and should not be modified. However, you must implement the constructor and the add_player method. __init__(self,n,height,width) The Game constructor needs to initialize three instance variables. The first, self.n, simply holds the integer value “n” provided as a parameter. The second, self.board, should be a new instance of the GameBoard class (described below), built using the provided height and width as dimensions. The third, self.players, should be an initially empty list. add_player(self,name,symbol) The add_player method is provided a name string and a symbol string. These two values should be…arrow_forwardI need the answer as soon as possiblearrow_forwardI've gotten stuck on this practice problem. I need help figuring this out. You need to make a Pet class that holds the following information as private data members: the name of the pet (e.g. "Spot", "Fluffy", or any word a user enters) the type of pet (e.g. dog, cat, snake, hamster, or any word a user enters) level of hungriness of the pet (2 means hungry, 1 means content, 0 means full) Your class also needs to have the following functions: a default constructor that sets the pet to be a dog named Buddy, with level of hungriness being “content” a parameterized constructor to allow having any type of pet the order of the parameters is: name of the pet, type of pet, hungriness level a PrintInfo function that prints the info for the pet according to the sample output below a TimePasses function that increases the hungry level of the pet by one (unless its hungry level is already 2, meaning hungry). a FeedPet function that changes the hungry level of the pet to 0 (Full) You must…arrow_forward
- Kindly Solve this C++ question as per the instructions. Thank you for your help! Instructions: 1- Put the class definition in Flight.h and the implementation of the constructors and functions in Flight.cpp Implement the Flight.h and Flight.cpp so that class Flight contains: 4 private instance variables: Name of data type string, which describe the three electric vehicle charger types (AC975, DL521, and AC863). Destination of data type string that shows the final destination of that flight. Hour and Minute of data type int that show the departure time. A default constructor which sets all of the numeric instance variables to zero and the String instance variables to null. A constructor with 4 parameters that sets the 4 instance variables to the corresponding values passed. Implement an accessor method for each information (Flight name, Flight destination, and Flight Time) that will return the value of the instance variable. For example, the getX() method for the instance variables…arrow_forwardWrite a class called Dog in C++. Dogs have attributes (such as number of legs and eye color), and they can run and bark and wag tails. Dogs can also optionally have a bone, and you'll have to create a Bone class for this. At a minimum, Dogs should have the following attributes: numberOfLegs (integer, must be between 0 and 4). Default to 4 (can be changed with a setter as shown below) eyeColor (a string that could be of any value) furColor (a string that could be of any value) weight (a float, must be between 1 and 200) isHappy (a boolean, defaulting to true) name (a string that could be of any value) Dogs should have a constructor and a destructor: The constructor should take one argument: a string representing the name. It should print a message noting that the dog as been created (e.g "_name_ is alive!") The destructor should print the dog's name and a message (e.g. "_name_ is no longer with us")arrow_forwardWrite a code using abstract data types, classes, and inheritance methods as in the example below. Do not use the car class exemplified, but think of any similar use of such a class and adapt it to your idea. Make sure to follow all the requirements set below: Creating the Car Class Motorvehicles Solutions, Inc. is a business that sells cars and leasing services. You are a programmer in the company's IT department, and your team is designing a program to manage all of the cars that are in the inventory. You have been asked to design a class that represents a car. The data that should be kept as attributes in the class are as follows: The name of the car's manufacturer will be assigned to the __manufact attribute. The car's model number will be assigned to the __model attribute The car's retail price will be assigned to the __retail_price attribute The car's leasing price will be assigned to the __leasing_price attribute The class will also have the following methods: An __init__…arrow_forward
- Program - Python Make a class.The most important thing is to write a simple class, create TWO different instances, and then be able to do something. For example, create a pizza instance for Suzie, ask her for the toppings from the choices, and store her pizza order. Then create a second pizza instance for Larry, ask for his toppings and store his pizza order. Then print both of their orders back out. You do not need to make your class very complicated. (Shouldn't be the pizza example but something similar)arrow_forwardFirst, you need to design, code in Java, test and document a base class, Student. The Student class willhave the following information, and all of these should be defined as Private:A. Title of the student (eg Mr, Miss, Ms, Mrs etc)B. A first name (given name)C. A last name (family name/surname)D. Student number (ID) – an integer number (of type long)E. A date of birth (in day/month/year format – three ints) - (Do NOT use the Date class fromJAVA)The student class will have at least the following constructors and methods:(i) two constructors - one without any parameters (the default constructor), and one withparameters to give initial values to all the instance variables.(ii) only necessary set and get methods for a valid class design.(iii) method to write the output of all the instance variables in the class to the screen (which willbe overridden in the respective child classes, as you have more instance variables thatrequired to be output).(iv) an equals method which compares two…arrow_forwardI am having trouble with doing this code on Python: Develop the Car class by creating an attribute purchase_price (type int) and the method print_info() that outputs the car's information. Ex: If the input is: 2011 18000 2018 where 2011 is the car's model year, 18000 is the purchase price, and 2018 is the current year, then print_info() outputs: Car's information: Model year: 2011 Purchase price: 18000 Current value: 5770 Note: print_info() should use three spaces for indentation.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
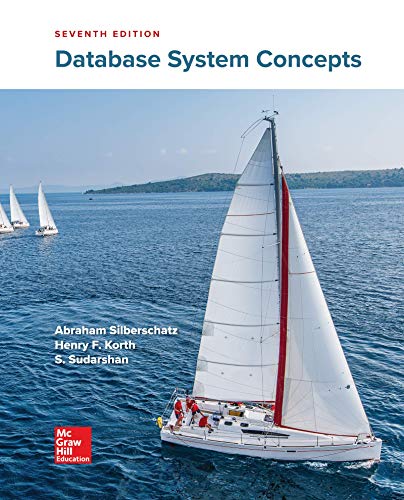
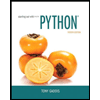
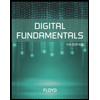
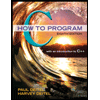
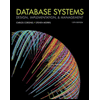
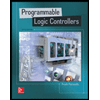