Answer this in C++: Given main(), complete the Calculator class (in files Calculator.h and Calculator.cpp) that emulates basic functions of a calculator: add, subtract, multiple, divide, and clear. The class has one private data member called value for the calculator's current value. Implement the following constructor and public member functions as listed below: Calculator() - default constructor to set the data member to 0.0 void Add(double val) - add the parameter to the data member void Subtract(double val) - subtract the parameter from the data member void Multiply(double val) - multiply the data member by the parameter void Divide(double val) - divide the data member by the parameter void Clear( ) - set the data member to 0.0 double GetValue( ) - return the data member Given two double input values num1 and num2, the program outputs the following values: The initial value of the data member, value The value after adding num1 The value after multiplying by 3 The value after subtracting num2 The value after dividing by 2 The value after calling the clear() method Ex: If the input is: 10.0 5.0 the output is: 0.0 10.0 30.0 25.0 12.5 0.0 main.cpp #include #include #include "Calculator.h" using namespace std; int main() { Calculator calc; double num1; double num2; cin >> num1; cin >> num2; cout << fixed << setprecision(1); // 1. The initial value cout << calc.GetValue() << endl; // 2. The value after adding num1 calc.Add(num1); cout << calc.GetValue() << endl; // 3. The value after multiplying by 3 calc.Multiply(3); cout << calc.GetValue() << endl; // 4. The value after subtracting num2 calc.Subtract(num2); cout << calc.GetValue() << endl; // 5. The value after dividing by 2 calc.Divide(2); cout << calc.GetValue() << endl; // 6. The value after calling the clear() method calc.Clear(); cout << calc.GetValue() << endl; return 0; } Calculator.h #ifndef CALCULATORH #define CALCULATORH class Calculator { public: // TODO: Declare default constructor // TODO: Declare member functions - // Add(), Subtract(), Multiply(), Divide(), Clear(), GetValue() private: // TODO: Declare private data member - value }; #endif Calculator.cpp #include #include "Calculator.h" using namespace std; // TODO: Define default constructor // TODO: Define member functions - // Add(), Subtract(), Multiply(), Divide(), Clear(), GetValue()
Answer this in C++: Given main(), complete the Calculator class (in files Calculator.h and Calculator.cpp) that emulates basic functions of a calculator: add, subtract, multiple, divide, and clear. The class has one private data member called value for the calculator's current value. Implement the following constructor and public member functions as listed below: Calculator() - default constructor to set the data member to 0.0 void Add(double val) - add the parameter to the data member void Subtract(double val) - subtract the parameter from the data member void Multiply(double val) - multiply the data member by the parameter void Divide(double val) - divide the data member by the parameter void Clear( ) - set the data member to 0.0 double GetValue( ) - return the data member Given two double input values num1 and num2, the program outputs the following values: The initial value of the data member, value The value after adding num1 The value after multiplying by 3 The value after subtracting num2 The value after dividing by 2 The value after calling the clear() method Ex: If the input is: 10.0 5.0 the output is: 0.0 10.0 30.0 25.0 12.5 0.0 main.cpp #include #include #include "Calculator.h" using namespace std; int main() { Calculator calc; double num1; double num2; cin >> num1; cin >> num2; cout << fixed << setprecision(1); // 1. The initial value cout << calc.GetValue() << endl; // 2. The value after adding num1 calc.Add(num1); cout << calc.GetValue() << endl; // 3. The value after multiplying by 3 calc.Multiply(3); cout << calc.GetValue() << endl; // 4. The value after subtracting num2 calc.Subtract(num2); cout << calc.GetValue() << endl; // 5. The value after dividing by 2 calc.Divide(2); cout << calc.GetValue() << endl; // 6. The value after calling the clear() method calc.Clear(); cout << calc.GetValue() << endl; return 0; } Calculator.h #ifndef CALCULATORH #define CALCULATORH class Calculator { public: // TODO: Declare default constructor // TODO: Declare member functions - // Add(), Subtract(), Multiply(), Divide(), Clear(), GetValue() private: // TODO: Declare private data member - value }; #endif Calculator.cpp #include #include "Calculator.h" using namespace std; // TODO: Define default constructor // TODO: Define member functions - // Add(), Subtract(), Multiply(), Divide(), Clear(), GetValue()
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter10: Classes And Data Abstraction
Section: Chapter Questions
Problem 29SA
Related questions
Question
Answer this in C++:
Given main(), complete the Calculator class (in files Calculator.h and Calculator.cpp) that emulates basic functions of a calculator: add, subtract, multiple, divide, and clear. The class has one private data member called value for the calculator's current value. Implement the following constructor and public member functions as listed below:
- Calculator() - default constructor to set the data member to 0.0
- void Add(double val) - add the parameter to the data member
- void Subtract(double val) - subtract the parameter from the data member
- void Multiply(double val) - multiply the data member by the parameter
- void Divide(double val) - divide the data member by the parameter
- void Clear( ) - set the data member to 0.0
- double GetValue( ) - return the data member
Given two double input values num1 and num2, the program outputs the following values:
- The initial value of the data member, value
- The value after adding num1
- The value after multiplying by 3
- The value after subtracting num2
- The value after dividing by 2
- The value after calling the clear() method
Ex: If the input is:
10.0
5.0
the output is:
0.0
10.0
30.0
25.0
12.5
0.0
main.cpp
#include <iostream>
#include <iomanip>
#include "Calculator.h"
using namespace std;
int main() {
Calculator calc;
double num1;
double num2;
cin >> num1;
cin >> num2;
cout << fixed << setprecision(1);
// 1. The initial value
cout << calc.GetValue() << endl;
// 2. The value after adding num1
calc.Add(num1);
cout << calc.GetValue() << endl;
// 3. The value after multiplying by 3
calc.Multiply(3);
cout << calc.GetValue() << endl;
// 4. The value after subtracting num2
calc.Subtract(num2);
cout << calc.GetValue() << endl;
// 5. The value after dividing by 2
calc.Divide(2);
cout << calc.GetValue() << endl;
// 6. The value after calling the clear() method
calc.Clear();
cout << calc.GetValue() << endl;
return 0;
}
Calculator.h
#ifndef CALCULATORH
#define CALCULATORH
class Calculator {
public:
// TODO: Declare default constructor
// TODO: Declare member functions -
// Add(), Subtract(), Multiply(), Divide(), Clear(), GetValue()
private:
// TODO: Declare private data member - value
};
#endif
Calculator.cpp
#include <iostream>
#include "Calculator.h"
using namespace std;
// TODO: Define default constructor
// TODO: Define member functions -
// Add(), Subtract(), Multiply(), Divide(), Clear(), GetValue()
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 7 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
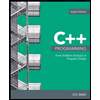
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
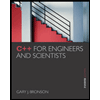
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
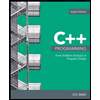
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
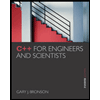
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr