(A)Write a class called Set to represent a set as an ArrayList and implement various set operations by using methods of class ArrayList. Assume that the elements of the set are integers. This class will have the following instance variables: (a) an ArrayList object called list of type Integer, (b) 1length: the actual number of elements in the list. This class will have the following methods: (1) Constructor without any parameter (default constructor), which uses a default value of 10 as capacity, creates ArrayList object list and initializes length to 0. (2) Constructor with parameter cap for capacity of the list. Create ArrayList object list of capacity equal to parameter cap and initialize length to 0. (3) Instance method getLength, that returns length. (4) Instance method isEmpty to determine whether the set is empty or not. (5) Instance method addElement that accepts a parameter element of type int. It will insert the element in the list at the end and also increment length by one. (6) Instance method isMember that accepts a parameter element of type int. If element exists in the list, it will return true, else it will return false. (7) Instance method subSet that accepts a parameter aSet of type Set. If aSet is a subset of "this obiect", it wvill return true, else it will return false.
(A)Write a class called Set to represent a set as an ArrayList and implement various set operations by using methods of class ArrayList. Assume that the elements of the set are integers. This class will have the following instance variables: (a) an ArrayList object called list of type Integer, (b) 1length: the actual number of elements in the list. This class will have the following methods: (1) Constructor without any parameter (default constructor), which uses a default value of 10 as capacity, creates ArrayList object list and initializes length to 0. (2) Constructor with parameter cap for capacity of the list. Create ArrayList object list of capacity equal to parameter cap and initialize length to 0. (3) Instance method getLength, that returns length. (4) Instance method isEmpty to determine whether the set is empty or not. (5) Instance method addElement that accepts a parameter element of type int. It will insert the element in the list at the end and also increment length by one. (6) Instance method isMember that accepts a parameter element of type int. If element exists in the list, it will return true, else it will return false. (7) Instance method subSet that accepts a parameter aSet of type Set. If aSet is a subset of "this obiect", it wvill return true, else it will return false.
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter18: Stacks And Queues
Section: Chapter Questions
Problem 16PE:
The implementation of a queue in an array, as given in this chapter, uses the variable count to...
Related questions
Question
Solve using Java and step by step following the questions
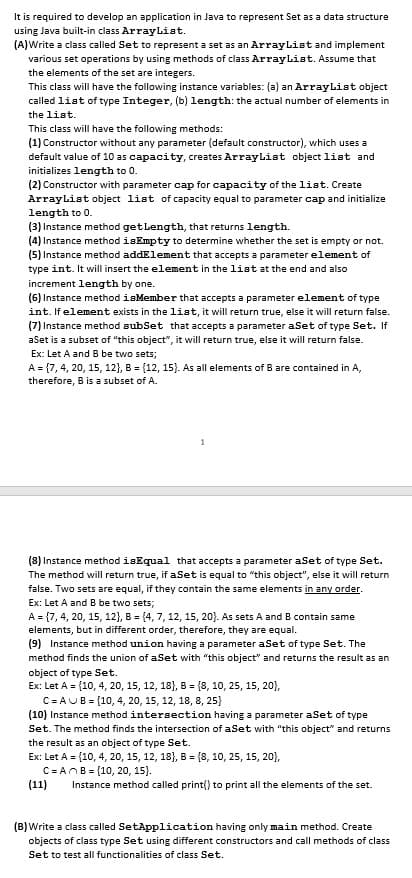
Transcribed Image Text:It is required to develop an application in Java to represent Set as a data structure
using Java built-in class ArrayList.
(A)Write a class called Set to represent a set as an ArrayList and implement
various set operations by using methods of class ArrayList. Assume that
the elements of the set are integers.
This class will have the following instance variables: (a) an ArrayList object
called list of type Integer, (b) length: the actual number of elements in
the list.
This class will have the following methods:
(1) Constructor without any parameter (default constructor), which uses a
default value of 10 as capacity, creates ArrayList object liat and
initializes length to 0.
(2) Constructor with parameter cap for capacity of the list. Create
ArrayList object list of capacity equal to parameter cap and initialize
length to 0.
(3) Instance method get Length, that returns length.
(4) Instance method isEmpty to determine whether the set is empty or not.
(5) Instance method addElement that accepts a parameter element of
type int. It will insert the element in the list at the end and also
increment length by one.
(6) Instance method isMember that accepts a parameter element of type
int. If element exists in the list, it will return true, else it will return false.
(7) Instance method subSet that accepts a parameter aSet of type Set. If
aSet is a subset of "this object", it will return true, else it will return false.
Ex: Let A and B be two sets;
A = {7, 4, 20, 15, 12}, B = {12, 15}. As all elements of B are contained in A,
therefore, B is a subset of A.
(8) Instance method isEqual that accepts a parameter aSet of type Set.
The method will return true, if aSet is equal to "this object", else it will return
false. Two sets are equal, if they contain the same elements in any order.
Ex: Let A and B be two sets;
A = {7, 4, 20, 15, 12}, B = (4, 7, 12, 15, 20}. As sets A and B contain same
elements, but in different order, therefore, they are equal.
(9) Instance method union having a parameter aSet of type Set. The
method finds the union of aSet with "this object" and returns the result as an
object of type Set.
Ex: Let A = {10, 4, 20, 15, 12, 18}, B = {8, 10, 25, 15, 20},
C= AUB = {10, 4, 20, 15, 12, 18, 8, 25}
(10) Instance method intersection having a parameter aSet of type
Set. The method finds the intersection of aSet with "this object" and returns
the result as an object of type Set.
Ex: Let A = {10, 4, 20, 15, 12, 18}, B = {8, 10, 25, 15, 20},
C= ANB = (10, 20, 15).
(11)
Instance method called print() to print all the elements of the set.
(B)Write a class called SetApplication having only main method. Create
objects of class type Set using different constructors and call methods of class
Set to test all functionalities of class Set.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
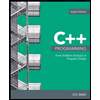
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
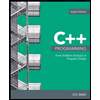
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning