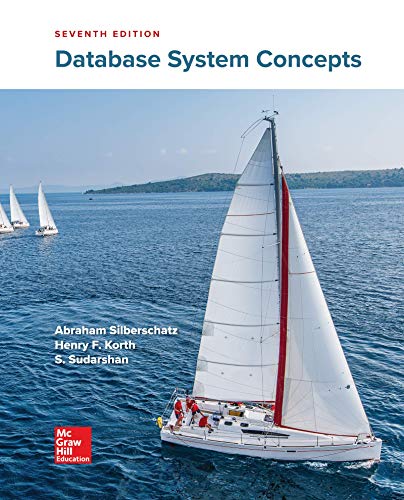
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Please write it in c# program.
Problem: BigNumber
Integer numbers in programming limit how large numbers can be processed, so double types come to
the rescue. Sometimes we need to implement bigger values. Create a class BigNumber that uses a 40-
element array of digits to store integers as large as 40 digits each. Provide methods Input, ToString, Add
and Subtract. For comparing BigNumber objects, provide the following methods: IsEqualTo,
IsNotEqualTo, IsGreaterThan, IsLessThan, IsGreaterThanOrEqualTo and IsLessThanOrEqualTo. Each of
these is a method that returns true if the relationship holds between the two BigNumber objects and
returns false if the relationship does not hold. Provide method IsZero. In the Input method, use the
string method ToCharArray to convert the input string into an array of characters, then iterate through
these characters to create your BigNumber. (Add Multiply and Divide methods For extra five points in
next exam)
Hint: use char –‘0’ to convert character to integer
Assume the user enter only digits and – sing to the left such as -56894
// Driver Class
using System;
public class BigNumberTest
{
public static void Main(string[] args)
{
BigNumber integer1 = new BigNumber ();
HugeInteger integer2 = new BigNumber();
Console.Write("Enter first BigNumber: ");
integer1.Input(Console.ReadLine());
Console.Write("Enter second BigNumber: ");
integer2.Input(Console.ReadLine());
Console.WriteLine($"BigNumber 1: {integer1}");
Console.WriteLine($"BigNumber 2: {integer2}");
BigNumber result;
// add two BigNumbers
result = integer1.Add(integer2);
Console.WriteLine($"Add result: {result}");
// subtract two BigNumbers
result = integer1.Subtract(integer2);
Console.WriteLine($"Subtract result: {result}");
// compare two BigNumbers
Console.WriteLine(
$"BigNumber 1 is zero: {integer1.IsZero()}");
Console.WriteLine(
$"BigNumber 2 is zero: {integer2.IsZero()}");
Console.WriteLine(
Integer numbers in programming limit how large numbers can be processed, so double types come to
the rescue. Sometimes we need to implement bigger values. Create a class BigNumber that uses a 40-
element array of digits to store integers as large as 40 digits each. Provide methods Input, ToString, Add
and Subtract. For comparing BigNumber objects, provide the following methods: IsEqualTo,
IsNotEqualTo, IsGreaterThan, IsLessThan, IsGreaterThanOrEqualTo and IsLessThanOrEqualTo. Each of
these is a method that returns true if the relationship holds between the two BigNumber objects and
returns false if the relationship does not hold. Provide method IsZero. In the Input method, use the
string method ToCharArray to convert the input string into an array of characters, then iterate through
these characters to create your BigNumber. (Add Multiply and Divide methods For extra five points in
next exam)
Hint: use char –‘0’ to convert character to integer
Assume the user enter only digits and – sing to the left such as -56894
// Driver Class
using System;
public class BigNumberTest
{
public static void Main(string[] args)
{
BigNumber integer1 = new BigNumber ();
HugeInteger integer2 = new BigNumber();
Console.Write("Enter first BigNumber: ");
integer1.Input(Console.ReadLine());
Console.Write("Enter second BigNumber: ");
integer2.Input(Console.ReadLine());
Console.WriteLine($"BigNumber 1: {integer1}");
Console.WriteLine($"BigNumber 2: {integer2}");
BigNumber result;
// add two BigNumbers
result = integer1.Add(integer2);
Console.WriteLine($"Add result: {result}");
// subtract two BigNumbers
result = integer1.Subtract(integer2);
Console.WriteLine($"Subtract result: {result}");
// compare two BigNumbers
Console.WriteLine(
$"BigNumber 1 is zero: {integer1.IsZero()}");
Console.WriteLine(
$"BigNumber 2 is zero: {integer2.IsZero()}");
Console.WriteLine(
$"BigNumber 1 is equal to BigNumber 2: {integer1.IsEqualTo(integer2)}");
Console.WriteLine(
$"BigNumber 1 is not equal to BigNumber 2:
{integer1.IsNotEqualTo(integer2)}");
Console.WriteLine(
$"BigNumber 1 is greater than BigNumber 2:
{integer1.IsGreaterThan(integer2)}");
Console.WriteLine(
$"BigNumber 1 is less than BigNumber 2:
{integer1.IsLessThan(integer2)}");
Console.WriteLine(
$"BigNumber 1 is greater than or equal to BigNumber 2:
{integer1.IsGreaterThanOrEqualTo(integer2)}");
Console.WriteLine(
$"BigNumber 1 is less than or equal to BigNumber 2:
{integer1.IsLessThanOrEqualTo(integer2)}");
}
}
Console.WriteLine(
$"BigNumber 1 is not equal to BigNumber 2:
{integer1.IsNotEqualTo(integer2)}");
Console.WriteLine(
$"BigNumber 1 is greater than BigNumber 2:
{integer1.IsGreaterThan(integer2)}");
Console.WriteLine(
$"BigNumber 1 is less than BigNumber 2:
{integer1.IsLessThan(integer2)}");
Console.WriteLine(
$"BigNumber 1 is greater than or equal to BigNumber 2:
{integer1.IsGreaterThanOrEqualTo(integer2)}");
Console.WriteLine(
$"BigNumber 1 is less than or equal to BigNumber 2:
{integer1.IsLessThanOrEqualTo(integer2)}");
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by stepSolved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Code these three methods.arrow_forward5. How to find all the leaders in an integer array in java? An element is leader if it is greater than all the elements to its right side. And the rightmost element is always a leader. For example int the array {16, 17, 4, 3, 5, 2}, leaders are 17, 5 and 2..arrow_forwardWrite and test a Java program that processes arrays as follows: Code a class that is called myArray consisting of the following methods: a. build and return an array of integer values (size 20 elements) b. search the array for a given element (linear search) c. Insert an element into the array after a certain element already contained in the array. This involves shifting array elements . and will require defining an array which has additional unused cells d. remove an element in an array (requires the use of searching and collapsing the array so that there are no gaps.) e. Write a method that uses a nested loop structure to create a 2-dimensional character array consisting of 6 rows and 4 columns. f. Write a method that reads the elements of the 2-D array, and displays the array contents (elements) one row per line.arrow_forward
- In JAVAarrow_forwardIn java i have some code for a method that finds the index of an integer that is passed in thet int is (target) the method also has the array called (array) and the first and last element of the array. also for this method to work the array has to be in descending order "static int find(int [] array, int first, int last, int target)" thats the method with all the variables that are passed to it my guess is that the first if statement just will have a short line that is "return -1;" because since the array has to be in descending order the first element cant be greater then the last element in the array but im confused on the rest because i cant find a way to make them one line codes with the conditions that i have to meet in the comments any help would be aprreaciatedarrow_forwardIntellij - Java Write a program which does the following: Create a class with the main() method. Take a string array input from the user in main() method. Note: Do not hardcode the string array in code. After the String array input loop, the array should be: words[] = {"today", "is", "a", "lovely", "spring","day", "not", "too", "hot", "not", "too", "cold"}; Print the contents of this array using Arrays.toString() method. Write a method called handleString() that performs the following: Takes a String[] array as method parameter (similar to main method). Loop through the array passed as parameter, and for each word in the array: If the length of the word is even, get the index of the last letter & print that index. Use length() and indexOf() String object methods. If the string length is odd, get the character at the 1st position in the string & print that letter. Use length() and charAt() String object methods.arrow_forward
- Problem: Write a method that will determine whether a given value can be made given an array of coin values. For example, each value in the array represents a coin with that value. An array with [1, 2, 3] represents 3 coins with the values, 1, 2, and 3. Determine whether or not these values can be used to make a desired value. The coins in the array are infinite and can only be used as many times as needed. Return true if the value can be made and false otherwise. Dynamic Programming would be handy for this problem. Data: An array containing 0 or more coin values and an amount. Output: Return true or false. Sample Data ( 1, 2, 3, 12, 5 ), 3 ( 4, 15, 16, 17, 1 ), 21 ( 1 ), 5 ( 3 ), 7 Sample Output true true true falsearrow_forwardin javaarrow_forward1. Deck.java The Deck class represents a deck of cards. It starts off with 52 cards, but as cards are dealt from the deck. the number of cards becomes smaller. The class has one private instance variable that stores the Cards that are currently in the Deck: private Card] carde; Important Note: The first Card in the array will be considered the card that Is at the top of the deck. Whon cards aro removed from the deck they will be removed from the front of the array. You must implement the flollowing methods for the Deck class: • public Dock) This constructor initializes the Deck with 52 card objacts, representing the 52 cards that are in a standard deck. The cards must be ordered as in the diagram below: Important: The FIRST card in your array should be the Ace of Spades, and the LAST card should be the King of Diamonds. Note that in the picture below, the Ace of Spades is at the TOP of the deck, which is to the left. A234567890 J.0KA234567 25456 10KA2 S45 57|8|90U.0高 • public Deck(Deck…arrow_forward
- Please help with this java problemarrow_forwardJava - Sort an Arrayarrow_forwardIn C# Use a one-dimensional array to solve the following problem. Write a class called ScoreFinder. This class receives a single dimensional integer array representing passer ratings for NFL players as an argument for its constructor. The test class will provides this argument. The test class then calls upon a method from the ScoreFinder class to start the process of finding a specific rating, which will be provided by the user. The method will iterate through the array and find any matching values. For all the matches that are found, the method will note the index of the cell along with its value. At completion, the method will print a list of all the indices and the associated scores along with a count of all the matching values.arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
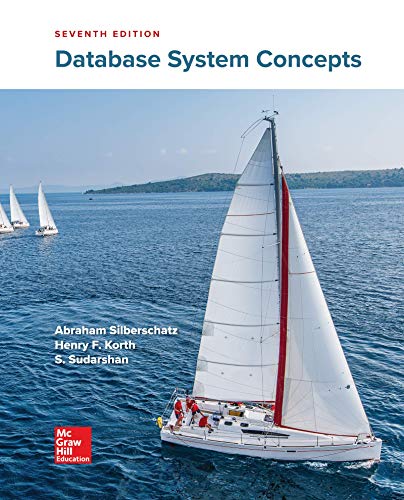
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
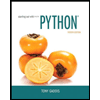
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
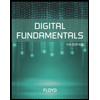
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
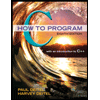
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
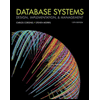
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
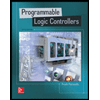
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education