Below for each class you find a UML and description of the public interface. Implementing the public interface as described is madatory. There's freedom on how to implement these classes.The private properties and private methods are under your control.. There are multiple ways of implementing all these classes. Feel free to add private properties and methods. For each object, it's mandatory to create a header file (.h), implementation file (.cpp) and a driver. Blank files are included. The header should have the class definition in it. The implementation file should contain the implementations of the methods laid out in the header fine. And finally the Driver should test/demonstrate all the features of the class. It's best to develop the driver as the class is being written. Check each section to see if there are added additional requirements for the driver. Two test suites are included so that work can be checked. It's important to implement the drivers to test and demonstrate Classes functionality. RoadTest.cpp: #define CATCH_CONFIG_MAIN static const int TEST_WIDTH = 15; static const int TEST_LENGTH = 200; #include "../Road.h" #include TEST_CASE("Should be able to set and get the width") { Road road; road.setWidth(TEST_WIDTH); int width = road.getWidth(); REQUIRE(width == TEST_WIDTH); } TEST_CASE("Should be able to set and get the length") { Road road; road.setLength(TEST_LENGTH); int length = road.getLength(); REQUIRE(length == TEST_LENGTH); } TEST_CASE("Should be able to get the Asphalt") { Road road; road.setWidth(TEST_WIDTH); road.setLength(TEST_LENGTH); float asphalt = road.asphalt(6); REQUIRE(asphalt == 7920000); } TEST_CASE("Copy Constructor should copy the width and Length"){ Road road; road.setWidth(TEST_WIDTH); road.setLength(TEST_LENGTH); Road copy = road; int width = copy.getWidth(); REQUIRE(width == TEST_WIDTH); int length = copy.getLength(); REQUIRE(length == TEST_LENGTH); } TEST_CASE("Copy Assignment Operator should copy the width and length") { Road road; road.setWidth(TEST_WIDTH); road.setLength(TEST_LENGTH); Road copy; copy = road; int width = copy.getWidth(); assert(width == TEST_WIDTH); int length = copy.getLength(); assert(length == TEST_LENGTH); } RoadDriver.cpp: #include using namespace std; #include "Road.h" int main() { std::cout << "Hello, Road Driver!" << std::endl; return 0; } Road.cpp: #include "Road.h" Road.h : #ifndef Road_h #define Road_h #endif
Below for each class you find a UML and description of the public interface. Implementing the public interface as described is madatory. There's freedom on how to implement these classes.The private properties and private methods are under your control.. There are multiple ways of implementing all these classes. Feel free to add private properties and methods.
For each object, it's mandatory to create a header file (.h), implementation file (.cpp) and a driver. Blank files are included. The header should have the class definition in it. The implementation file should contain the implementations of the methods laid out in the header fine. And finally the Driver should test/demonstrate all the features of the class. It's best to develop the driver as the class is being written. Check each section to see if there are added additional requirements for the driver. Two test suites are included so that work can be checked. It's important to implement the drivers to test and demonstrate Classes functionality.
RoadTest.cpp:
#define CATCH_CONFIG_MAIN
static const int TEST_WIDTH = 15;
static const int TEST_LENGTH = 200;
#include "../Road.h"
#include <catch2/catch_test_macros.hpp>
TEST_CASE("Should be able to set and get the width") {
Road road;
road.setWidth(TEST_WIDTH);
int width = road.getWidth();
REQUIRE(width == TEST_WIDTH);
}
TEST_CASE("Should be able to set and get the length") {
Road road;
road.setLength(TEST_LENGTH);
int length = road.getLength();
REQUIRE(length == TEST_LENGTH);
}
TEST_CASE("Should be able to get the Asphalt") {
Road road;
road.setWidth(TEST_WIDTH);
road.setLength(TEST_LENGTH);
float asphalt = road.asphalt(6);
REQUIRE(asphalt == 7920000);
}
TEST_CASE("Copy Constructor should copy the width and Length"){
Road road;
road.setWidth(TEST_WIDTH);
road.setLength(TEST_LENGTH);
Road copy = road;
int width = copy.getWidth();
REQUIRE(width == TEST_WIDTH);
int length = copy.getLength();
REQUIRE(length == TEST_LENGTH);
}
TEST_CASE("Copy Assignment Operator should copy the width and length") {
Road road;
road.setWidth(TEST_WIDTH);
road.setLength(TEST_LENGTH);
Road copy;
copy = road;
int width = copy.getWidth();
assert(width == TEST_WIDTH);
int length = copy.getLength();
assert(length == TEST_LENGTH);
}
RoadDriver.cpp:
#include <iostream>
using namespace std;
#include "Road.h"
int main() {
std::cout << "Hello, Road Driver!" << std::endl;
return 0;
}
Road.cpp:
#include "Road.h"
Road.h :
#ifndef Road_h
#define Road_h
#endif
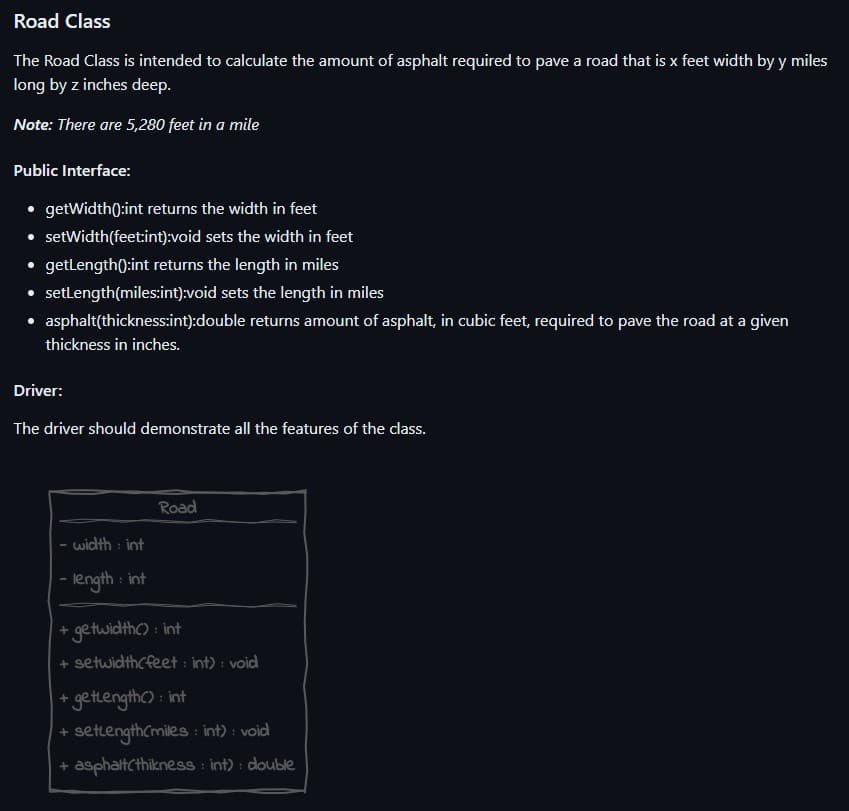

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

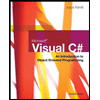
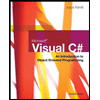