Given base and n that are both 1 or more, compute recursively (no loops) the value of base to the n power, so powerN(3, 2) is 9 (3 squared). powerN(3, 1) → 3 powerN(3, 2) → 9 powerN(3, 3) → 27
- [Very Easy]
Given base and n that are both 1 or more, compute recursively (no loops) the value of base
to the n power, so powerN(3, 2) is 9 (3 squared).
powerN(3, 1) → 3
powerN(3, 2) → 9
powerN(3, 3) → 27
- [Easy]
class Trace:
def hMB(self,h):
if (h==0):
print("Stop: ",h)
return 0
elif(h==1):
print("Stop: ",h)
return h
else:
print("Continue: ",h)
return h + self.hMB(h-1)
#Tester
t = Trace()
print("Finally ",t.hMB(5))
- [Medium]
Suppose, you have been given a non-negative integer which is the height of a ‘house of
cards’. To build such a house you at-least require 8 cards. To increase the level (or height)
of that house, you would require four sides and a base for each level. Therefore, for the top
level, you would require 8 cards and for each of the rest of the levels below you would
require 5 extra cards. If you were asked to build level one only, you would require just 8
cards. Of course, the input can be zero; in that case, you do not build a house at all.
Complete the recursive method below to calculate the number of cards required to build a
‘house of cards’ of specific height given by the parameter.
public int hocBuilder (int height){
// TO DO
}
OR
def hocBuilder(height):
#TO DO
- Draw the recursive flow diagram/memory stack (draw each methods and their behavior in
the way they are being called and executed) of the code given below:
public class Surprise{
public static int mystery(int n) {
System.out.println("h(" + n + ")");
if (n == 0) {
System.out.println("value: 0"); return 0;
} else {
System.out.println("going down");
int temp = mystery (n - 1) + 1;
System.out.println("h(" + n + ") --> " + temp);
return temp;
}
}
public static void main(String [] args){
mystery(4);
}
OR
class Surprise:
def mystery(self,n):
print("h(" ,n,")")
if(n==0):
print("value: 0")
return 0
else:
print("going down")
temp = self.mystery(n-1)+1
print("h(",n,") --> ",temp)
return temp
#Tester
s = Surprise()
s.mystery(4)
- [Hard]
- Print the following pattern for the given input:
Input: 5
Output:
1
1 2
1 2 3
1 2 3 4
1 2 3 4 5
- Print the following pattern for the given input:
Input: 5
Output:
1
1 2
1 2 3
1 2 3 4
1 2 3 4 5
- [Very Hard]
Suppose, you are working in a company ‘X’ where your job is to calculate the profit based
on their investment.
If the company invests 100,000 USD or less, their profit will be based on 75,000 USD as
first 25,000 USD goes to set up the business in the first place. For the first 100,000 USD,
the profit margin is low: 4.5%. Therefore, for every 100 dollar they spend, they get a profit
of 4.5 dollar.
For an investment greater than 100,000 USD, for the first 100,000 USD (actually on 75,000
USD as 25,000 is the setup cost), the profit margin is 4.5% where for the rest, it goes up to
8%. For example, if they invest 250,000 USD, they will get an 8% profit for the 150,000
USD. In addition, from the rest 100,000 USD, 25,000 is the setup cost and there will be a
4.5% profit on the rest 75,000. Investment will always be greater or equal to 25,000 and
multiple of 100.
Complete the RECURSIVE methods below that take an array of integers (investments)
and an iterator (always sets to ZERO(‘0’) when the method is initially called) and prints
the profit for the corresponding investment. You must avoid loop and multiplication (‘*’)
operator.
public class FinalQ{
public static void print(int[]array,int idx){
if(idx<array.length){
double profit=calcProfit(array[idx]);
//TODO
}
}
public static double calcProfit(int investment){
//TODO
}
public static void main(String[]args){
int[]array={25000,100000,250000,350000};
print(array,0);
}
}
OR
class FinalQ:
def print(self,array,idx):
if(idx<len(array)):
profit = self.calcProfit(array[idx])
#TO DO
def calcProfit(self,investment):
#TO DO
#Tester
array=[25000,100000,250000,350000]
f = FinalQ()
f.print(array,0)
-------------------------------------------------
Output:
- Investment: 25000; Profit: 0.0
- Investment: 100000; Profit: 3375.0
- Investment: 250000; Profit: 15375.0
- Investment: 350000; Profit: 23375.0

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 4 images

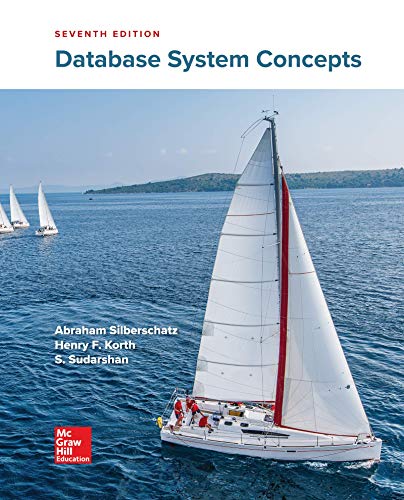
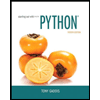
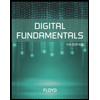
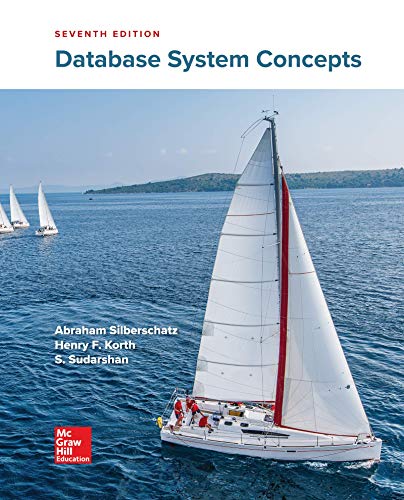
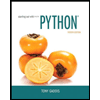
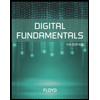
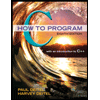
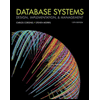
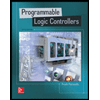