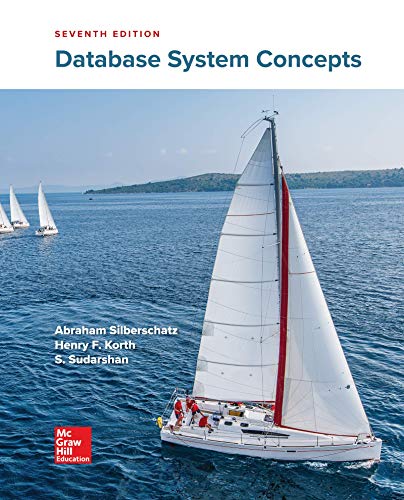
Code is complete, I just need an explanation on how this code functions.
#include <iostream>
using namespace std;
class node
{
public:
double data;
node* next;
};
node* head;
void addData(double val)
{
node *n = new node;
n->data=val;
n->next=NULL;
if(head==NULL)
{
head=n;
}
else
{
node *ptr=head;
while(ptr->next!=NULL)
{
ptr=ptr->next;
}
ptr->next=n;
}
}
void addBack(double x)
{
node *n = new node;
n->data=x;
n->next=NULL;
node *ptr=head;
while(ptr->next!=NULL)
{
ptr=ptr->next;
}
ptr->next=n;
}
void display()
{
node *ptr=head;
while(ptr!=NULL)
{
cout<<ptr->data<<", ";
ptr=ptr->next;
}
cout<<endl;
}
int main()
{
head=NULL;
addData(4.7);
addData(12.8);
addData(14.7);
addData(16.2);
cout<<"Linked List"<<endl;
display();
addBack(56.9);
cout<<"After adding node with data 56.9 to the back of linked list:";
display();
addBack(11.9);
cout<<"After adding node with data 11.9 to the back of linked list:";
display();
addBack(34.9);
cout<<"After adding node with data 34.9 to the back of linked list:";
display();
return 0;
}

Step by stepSolved in 2 steps

- member and non-member functions c++arrow_forward#include <iostream> using namespace std; class Player { private: int id; static int next_id; public: int getID() { return id; } Player() { id = next_id++; } }; int Player::next_id = 1; int main() { Player p1; Player p2; Player p3; cout << p1.getID() << " "; cout << p2.getID() << " "; cout << p3.getID(); return 0; } Run the program and give its output.arrow_forwardint machineCompare1(struct machine* mac1, struct machine* mac2){ struct mac1; struct mac2; char make1[21], make2[21]; char model1[51], model2[21]; if((lap1 -> make1 == lap2 -> make2) && (lap1 -> model1 == lap2 -> model2)) return 0; else return 1;} have to create function to compare make and model of 2 machines - it's using structures - not sure if this is correct/ on the right track?arrow_forward
- In C, using malloc to allocate memory for a linked list uses which memory allocation scheme? Heap allocation Static allocationarrow_forwardC++ Program #include <iostream>#include <cstdlib>#include <ctime>using namespace std; int getData() { return (rand() % 100);} class Node {public: int data; Node* next;}; class LinkedList{public: LinkedList() { // constructor head = NULL; } ~LinkedList() {}; // destructor void addNode(int val); void addNodeSorted(int val); void displayWithCount(); int size(); void deleteAllNodes(); bool exists(int val);private: Node* head;}; // function to check data exist in a listbool LinkedList::exists(int val){ if (head == NULL) { return false; } else { Node* temp = head; while (temp != NULL) { if(temp->data == val){ return true; } temp = temp->next; } } return false;} // function to delete all data in a listvoid LinkedList::deleteAllNodes(){ if (head == NULL) { cout << "List is empty, No need to delete…arrow_forward#include <iostream>using namespace std;class Player{private:int id;static int next_id;public:int getID() { return id; }Player() { id = next_id++; }};int Player::next_id = 1;int main(){Player p1;Player p2;Player p3;cout << p1.getID() << " ";cout << p2.getID() << " ";cout << p3.getID();return 0;} Run the program and give its output.arrow_forward
- #ifndef LLCP_INT_H#define LLCP_INT_H #include <iostream> struct Node{ int data; Node *link;};void DelOddCopEven(Node*& headPtr);int FindListLength(Node* headPtr);bool IsSortedUp(Node* headPtr);void InsertAsHead(Node*& headPtr, int value);void InsertAsTail(Node*& headPtr, int value);void InsertSortedUp(Node*& headPtr, int value);bool DelFirstTargetNode(Node*& headPtr, int target);bool DelNodeBefore1stMatch(Node*& headPtr, int target);void ShowAll(std::ostream& outs, Node* headPtr);void FindMinMax(Node* headPtr, int& minValue, int& maxValue);double FindAverage(Node* headPtr);void ListClear(Node*& headPtr, int noMsg = 0); // prototype of DelOddCopEven of Assignment 5 Part 1 #endifarrow_forwardI need to convert this code using C++, that currently works with structs into classes, with the main class being called myGraph and a node class (if necessary) using composition. #include <stdio.h>#include <stdlib.h>#include <iostream>using namespace std; struct AdjListNode //node class{ int dest; struct AdjListNode* next;}; struct AdjList //node class{ struct AdjListNode *head; }; struct Graph //myGraph class{ int V; struct AdjList* array;}; struct AdjListNode * newAdjListNode(int dest){ struct AdjListNode * newNode = new AdjListNode; newNode->dest = dest; newNode->next = NULL; return newNode;} struct Graph* createGraph(int V) //constructor{ struct Graph* graph = new Graph; graph->V = V; graph->array = new AdjList; int i; for (i = 0; i < V; ++i) graph->array[i].head = NULL; return graph;} void addEdge(struct Graph* graph, int src, int dest){ struct AdjListNode* newNode =…arrow_forwardC++ Data Structure:Create an AVL Tree C++ class that works similarly to std::map, but does NOT use std::map. In the PRIVATE section, any members or methods may be modified in any way. Standard pointers or unique pointers may be used.** MUST use given Template below: #ifndef avltree_h#define avltree_h #include <memory> template <typename Key, typename Value=Key> class AVL_Tree { public: classNode { private: Key k; Value v; int bf; //balace factor std::unique_ptr<Node> left_, right_; Node(const Key& key) : k(key), bf(0) {} Node(const Key& key, const Value& value) : k(key), v(value), bf(0) {} public: Node *left() { return left_.get(); } Node *right() { return right_.get(); } const Key& key() const { return k; } const Value& value() const { return v; } const int balance_factor() const {…arrow_forward
- #include <iostream>using namespace std;class Player{private:int id;static int next_id;public:int getID() { return id; }Player() { id = next_id++; }};int Player::next_id = 1;int main(){Player p1;Player p2;Player p3;cout << p1.getID() << " ";cout << p2.getID() << " ";cout << p3.getID();return 0;} Run the program and give its output.arrow_forward#include <iostream>using namespace std;class Player{private:int id;static int next_id;public:int getID() { return id; }Player() { id = next_id++; }};int Player::next_id = 1;int main(){Player p1;Player p2;Player p3;cout << p1.getID() << " ";cout << p2.getID() << " ";cout << p3.getID();return 0;} Run the program and give its output.arrow_forwardC++ Given code #include <iostream>using namespace std; class Node {public:int data;Node *pNext;}; void displayNumberValues( Node *pHead){while( pHead != NULL) {cout << pHead->data << " ";pHead = pHead->pNext;}cout << endl;} //Option 1: Search the list// TODO: complete the function below to search for a given value in linked lsit// return true if value exists in the list, return false otherwise. ?? linkedlistSearch( ???){ } //Option 2: get sum of all values// TODO: complete the function below to return the sum of all elements in the linked list. ??? getSumOfAllNumbers( ???){ } int main(){int userInput;Node *pHead = NULL;Node *pTemp;cout<<"Enter list numbers separated by space, followed by -1: "; cin >> userInput;// Keep looping until end of input flag of -1 is givenwhile( userInput != -1) {// Store this number on the listpTemp = new Node;pTemp->data = userInput;pTemp->pNext = pHead;pHead = pTemp;cin >> userInput;}cout <<"…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
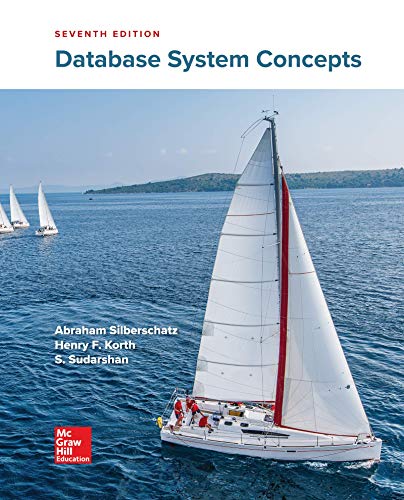
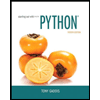
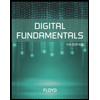
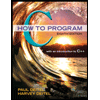
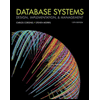
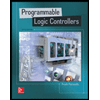