class Time { public: Time (int hours, int minutes); const Time operator-(const Time& rhs) const; // difference private: int m_hours; int m_minutes; // 0..59 }; // 0..23
class Time { public: Time (int hours, int minutes); const Time operator-(const Time& rhs) const; // difference private: int m_hours; int m_minutes; // 0..59 }; // 0..23
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
C++
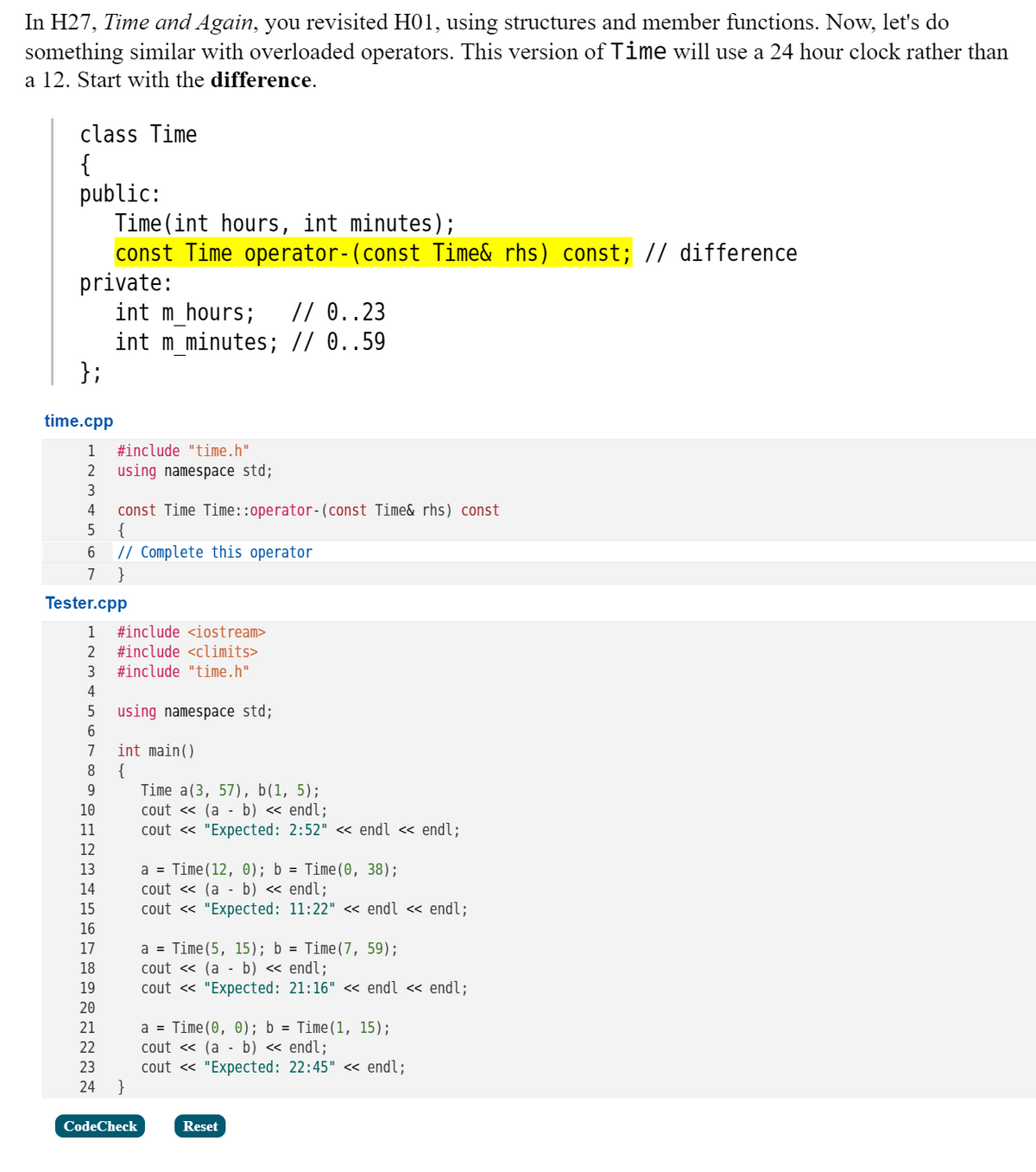
Transcribed Image Text:In H27, Time and Again, you revisited H01, using structures and member functions. Now, let's do
something similar with overloaded operators. This version of Time will use a 24 hour clock rather than
a 12. Start with the difference.
class Time
{
public:
Time (int hours, int minutes);
const Time operator-(const Time& rhs) const; // difference
private:
int m_hours;
int m_minutes; // 0..59
};
// 0..23
time.cpp
1
#include "time.h"
2
using namespace std;
3
const Time Time::operator-(const Time& rhs) const
{
// Complete this operator
4
7
}
Tester.cpp
1
#include <iostream>
2
#include <climits>
3
#include "time.h"
4
5
using namespace std;
int main()
{
Time a(3, 57), b(1, 5);
cout « (a - b) « endl;
cout « "Expected: 2:52" « endl « endl;
7
9
10
11
12
a = Time(12, 0); b = Time(0, 38);
cout « (a - b) « endl;
cout « "Expected: 11:22" << endl « endl;
13
14
15
16
a = Time(5, 15); b = Time(7, 59);
cout « (a - b) « endl;
cout « "Expected: 21:16" << endl « endl;
17
18
19
20
a = Time(0, 0); b = Time(1, 15);
cout « (a - b) « endl;
cout « "Expected: 22:45" << endl;
}
21
22
23
24
CodeCheck
Reset
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
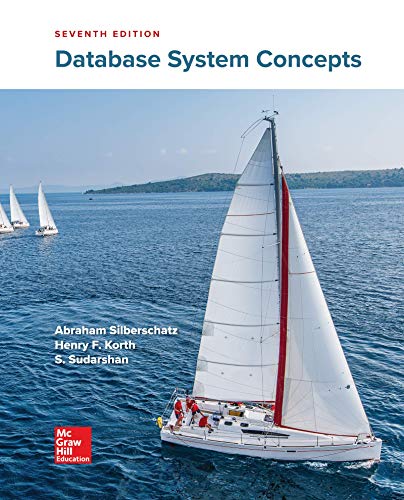
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
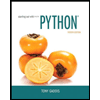
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
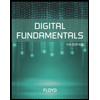
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
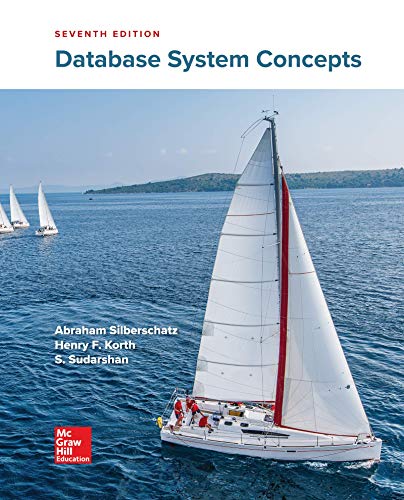
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
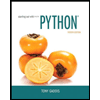
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
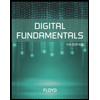
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
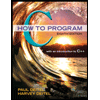
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
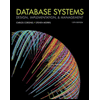
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
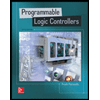
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education