Programming Fundamentals II JAVA Lab #7-Inheritance NAME Problem: Develop the 'Shape' application such that: 'Rectangle', 'Ellipse', and 'Triangle' classes inherit from the 'Shape' class Develop the 'Square' and 'Circle' class where 'Square' inherits from 'Rectangle' and Circle' inherits from Ellipse'. 'Triangle' has no derived class. For each class, implement the overridden methods 'draw', 'move', and 'erase'. Each method should only have an output statement such as "Rectangle draw method" that will be displayed when the method is invoked Implement the default constructors for each class with a corresponding message to be displayed when invoked. No initializations are required; that is, the output message will be the only executable statement in the constructors Do not implement any other methods for these classes ( i.e., 'toString', 'equals', getters and setters ) Implement a 'ShapeTest' class which will instantiate an object of each class . Exercise each of the 'draw', 'move', and 'erase' methods of each class Remember to make sure that there is only a single class per file.
Programming Fundamentals II JAVA Lab #7-Inheritance NAME Problem: Develop the 'Shape' application such that: 'Rectangle', 'Ellipse', and 'Triangle' classes inherit from the 'Shape' class Develop the 'Square' and 'Circle' class where 'Square' inherits from 'Rectangle' and Circle' inherits from Ellipse'. 'Triangle' has no derived class. For each class, implement the overridden methods 'draw', 'move', and 'erase'. Each method should only have an output statement such as "Rectangle draw method" that will be displayed when the method is invoked Implement the default constructors for each class with a corresponding message to be displayed when invoked. No initializations are required; that is, the output message will be the only executable statement in the constructors Do not implement any other methods for these classes ( i.e., 'toString', 'equals', getters and setters ) Implement a 'ShapeTest' class which will instantiate an object of each class . Exercise each of the 'draw', 'move', and 'erase' methods of each class Remember to make sure that there is only a single class per file.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Develop the ‘Shape’ application such that:
Implement an array of objects of various types (all SIX classes), in any order.
In some type of a looping structure, demonstrate polymorphism by calling all three
of the methods, draw, move, and erase. That is, within the curly braces, there will
be only three method calls.
Verify that the output messages come from all three methods, from all seven
classes.
The only class that you should have to develop for this class will be the test
application. The six shape classes from Lab-7 should remain unchanged.

Transcribed Image Text:Programming Fundamentals II JAVA
Lab #7-Inheritance
NAME
Problem: Develop the 'Shape' application such that:
'Rectangle', 'Ellipse', and 'Triangle' classes inherit from the 'Shape' class
Develop the 'Square' and 'Circle' class where 'Square' inherits from 'Rectangle' and
Circle' inherits from Ellipse'. 'Triangle' has no derived class.
For each class, implement the overridden methods 'draw', 'move', and 'erase'. Each
method should only have an output statement such as "Rectangle draw method"
that will be displayed when the method is invoked
Implement the default constructors for each class with a corresponding message to
be displayed when invoked. No initializations are required; that is, the output
message will be the only executable statement in the constructors
Do not implement any other methods for these classes ( i.e., 'toString', 'equals',
getters and setters )
Implement a 'ShapeTest' class which will instantiate an object of each class
. Exercise each of the 'draw', 'move', and 'erase' methods of each class
Remember to make sure that there is only a single class per file.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
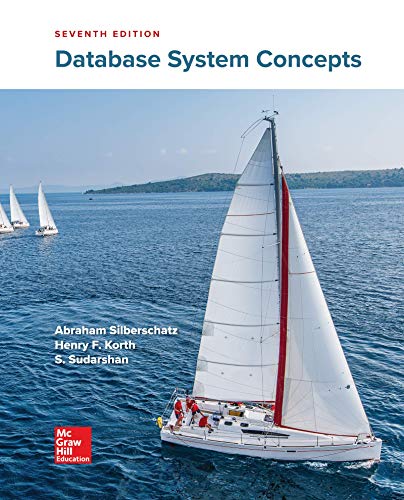
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
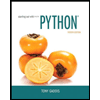
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
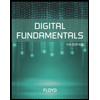
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
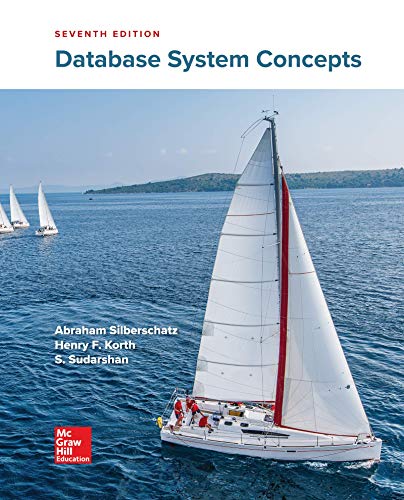
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
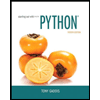
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
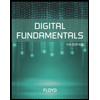
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
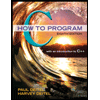
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
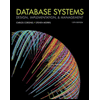
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
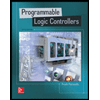
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education