Write a MIPS program to convert the following C code to MIPS: int bigger (int, int); int smaller (int, int); int main () int x = 30; int y = 27; int z - biger (x, y): int w - smaller (x, y): return 0; }// end main int bigger (int numl, int num2) { if (num2 < numl) ( return numl; ) else { return num2; int smaller (int numl, int num2) { if (num2 < numl) ( return num2; } else { return numl; At the beginning of the .asm file, type the following li $s0, 30 li $s1, 27 As you write the MIPS code, be aware of the following: • You will need to give the $a0 and $a1 registers values before calling the two procedures. Use two add instructions with the $zero register to do this. The Bigger procedure, which will need its own label, must determine which of $a0 and $a1 has the bigger value. Use a set-less-than (slt) instructions, followed by a branch instruction (beq or bne, you decide) to do this. • Once the bigger value is found, its register must be added to the $v0 register (add instruction with $zero) so that the correct value is returned. • When the value is returned, use another add instruction with $zero to store the bigger value in $s2. • The Smaller procedure will be similar to the Bigger procedure except its result should be stored in $s3 when it is done. • After $s2 and $s3 gets their values, you will need to use a jump 6) instruction with a label (like Exit) that will jump below the two procedures and end the program. • Be sure to test your program with the bigger value in $s0 and with the bigger value in $1. • Use any temporary registers ($t0, $t1, etc.) as needed to make sure instructions work. • You might have to use different labels within each procedure. • Make sure your program assembles by clicking this button: • Execute the program by clicking this button: • tis highly recommended you use the single-step feature to check values for registers after each instruction executes. You can single-step by clicking this button:
Write a MIPS program to convert the following C code to MIPS: int bigger (int, int); int smaller (int, int); int main () int x = 30; int y = 27; int z - biger (x, y): int w - smaller (x, y): return 0; }// end main int bigger (int numl, int num2) { if (num2 < numl) ( return numl; ) else { return num2; int smaller (int numl, int num2) { if (num2 < numl) ( return num2; } else { return numl; At the beginning of the .asm file, type the following li $s0, 30 li $s1, 27 As you write the MIPS code, be aware of the following: • You will need to give the $a0 and $a1 registers values before calling the two procedures. Use two add instructions with the $zero register to do this. The Bigger procedure, which will need its own label, must determine which of $a0 and $a1 has the bigger value. Use a set-less-than (slt) instructions, followed by a branch instruction (beq or bne, you decide) to do this. • Once the bigger value is found, its register must be added to the $v0 register (add instruction with $zero) so that the correct value is returned. • When the value is returned, use another add instruction with $zero to store the bigger value in $s2. • The Smaller procedure will be similar to the Bigger procedure except its result should be stored in $s3 when it is done. • After $s2 and $s3 gets their values, you will need to use a jump 6) instruction with a label (like Exit) that will jump below the two procedures and end the program. • Be sure to test your program with the bigger value in $s0 and with the bigger value in $1. • Use any temporary registers ($t0, $t1, etc.) as needed to make sure instructions work. • You might have to use different labels within each procedure. • Make sure your program assembles by clicking this button: • Execute the program by clicking this button: • tis highly recommended you use the single-step feature to check values for registers after each instruction executes. You can single-step by clicking this button:
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
MIPS ONLY
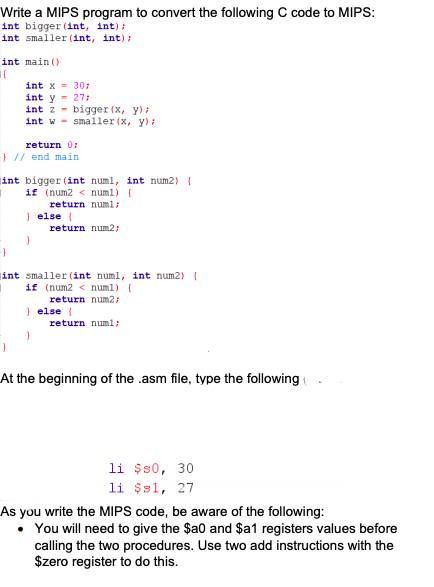
Transcribed Image Text:Write a MIPS program to convert the following C code to MIPS:
int bigger (int, int);
int smaller (int, int);
int main ()
int x = 30;
int y = 27;
int z - biger (x, y):
int w - smaller (x, y):
return 0;
}// end main
int bigger (int numl, int num2) {
if (num2 < numl) (
return numl;
) else {
return num2;
int smaller (int numl, int num2) {
if (num2 < numl) (
return num2;
} else {
return numl;
At the beginning of the .asm file, type the following
li $s0, 30
li $s1, 27
As you write the MIPS code, be aware of the following:
• You will need to give the $a0 and $a1 registers values before
calling the two procedures. Use two add instructions with the
$zero register to do this.
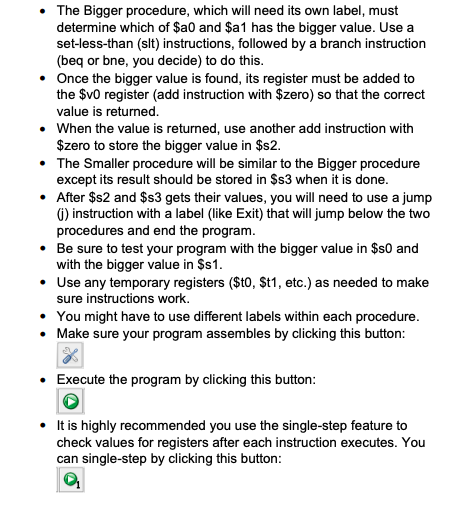
Transcribed Image Text:The Bigger procedure, which will need its own label, must
determine which of $a0 and $a1 has the bigger value. Use a
set-less-than (slt) instructions, followed by a branch instruction
(beq or bne, you decide) to do this.
• Once the bigger value is found, its register must be added to
the $v0 register (add instruction with $zero) so that the correct
value is returned.
• When the value is returned, use another add instruction with
$zero to store the bigger value in $s2.
• The Smaller procedure will be similar to the Bigger procedure
except its result should be stored in $s3 when it is done.
• After $s2 and $s3 gets their values, you will need to use a jump
6) instruction with a label (like Exit) that will jump below the two
procedures and end the program.
• Be sure to test your program with the bigger value in $s0 and
with the bigger value in $1.
• Use any temporary registers ($t0, $t1, etc.) as needed to make
sure instructions work.
• You might have to use different labels within each procedure.
• Make sure your program assembles by clicking this button:
• Execute the program by clicking this button:
• tis highly recommended you use the single-step feature to
check values for registers after each instruction executes. You
can single-step by clicking this button:
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
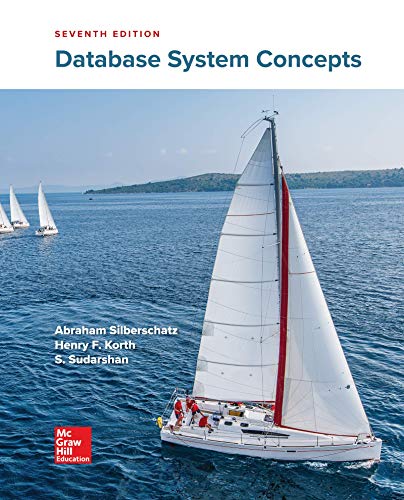
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
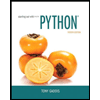
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
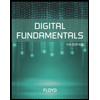
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
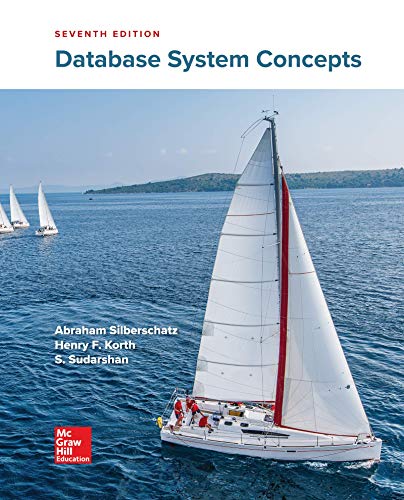
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
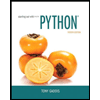
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
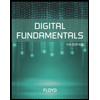
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
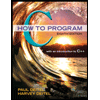
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
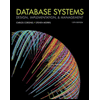
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
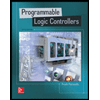
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education