Convert this to sorted array #include #include"Student.cpp" class StudentList { private: struct ListNode { Student astudent; ListNode *next; }; ListNode *head; public: StudentList(); ~StudentList(); int IsEmpty(); void Add(Student newstudent); void Remove(); void DisplayList(); }; StudentList::StudentList() { head=NULL; }; StudentList::~StudentList() { cout <<"\nDestructing the objects..\n"; while(IsEmpty()!=0) Remove(); if(IsEmpty()==0) cout <<"All students have been deleted from a list\n"; }; int StudentList::IsEmpty() { if(head==NULL) return 0; else return 1; }; void StudentList::Add(Student newstudent) { ListNode *newPtr=new ListNode; if(newPtr==NULL) cout <<"Cannot allocate memory"; else { newPtr->astudent=newstudent; newPtr->next=head; head=newPtr; } }; void StudentList::Remove() { if(IsEmpty()==0) cout <<"List empty on remove"; else { ListNode *temp=head; head=head->next; temp->next=NULL; delete temp; } }; void StudentList::DisplayList() { ListNode *cur=head; if(IsEmpty()==0) cout <<"List empty\n"; else {
Q: Convert this to sorted array
#include<iostream>
#include"Student.cpp"
class StudentList
{
private:
struct ListNode
{
Student astudent;
ListNode *next;
};
ListNode *head;
public:
StudentList();
~StudentList();
int IsEmpty();
void Add(Student newstudent); void Remove();
void DisplayList();
};
StudentList::StudentList() {
head=NULL;
};
StudentList::~StudentList()
{
cout <<"\nDestructing the objects..\n";
while(IsEmpty()!=0)
Remove();
if(IsEmpty()==0)
cout <<"All students have been deleted from a list\n"; };
int StudentList::IsEmpty()
{
if(head==NULL)
return 0;
else
return 1;
};
void StudentList::Add(Student newstudent) {
ListNode *newPtr=new ListNode;
if(newPtr==NULL)
cout <<"Cannot allocate memory";
else
{
newPtr->astudent=newstudent;
newPtr->next=head;
head=newPtr;
}
};
void StudentList::Remove() {
if(IsEmpty()==0)
cout <<"List empty on remove"; else
{
ListNode *temp=head;
head=head->next;
temp->next=NULL;
delete temp;
}
};
void StudentList::DisplayList() {
ListNode *cur=head;
if(IsEmpty()==0)
cout <<"List empty\n";
else
{
cout <<"Current List: \n"; while(cur!=NULL)
{
cur->astudent.GetData(); cur=cur->next;
}
cout <<"\n";
} //end if
};// method display list
-------------------------------------------------------------------------------
Student ccp:
#include <iostream>
using namespace std;
class Student
{
private:
struct Data
{
char Name[25];
char Course[30];
int Result;
}stdata;
public:
void SetData();
void GetData();
}; //class Student
void Student::SetData()
{
cout <<"Enter student name: ";
cin >>stdata.Name;
cout <<"Enter student course: ";
cin >>stdata.Course;
cout <<"Enter student Result:";
cin >>stdata.Result;
}; //method SetData
void Student::GetData()
{
cout <<"\nStudent name: "<<stdata.Name;
cout <<"\n";
cout <<"\nStudent course: "<<stdata.Course;
cout <<"\n";
cout <<"\nStudent result: "<<stdata.Result;
cout <<"\n";
}; //method GetData

Step by step
Solved in 2 steps

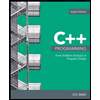
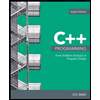