Create three files: Payroll class.java -Base class definition PayrollOvertime.jave - Derived class definition PayrollOvertimeClient.java - contains main() method Implement the two user define classes with the following specifications: Payroll class (the base class) 5 data fields(protected) String name - Initialized in default constructor to "John Doe" int ID - Initialized in default constructor to 9999 doulbe payRate - Initialized in default constructor to 15.0 doulbe hrWorked - Initialized in default constructor to 40 static int data field named nOfObjs for tracking the number of employees, (initialized to 0 and updated nOfObjs++ in the constructors) 2 constructors (public) Default constructor (make sure to update the nOfObjs member) A constructor that accepts the employee’s name, ID, and pay rate as arguments. These values should be assigned to the object’s name, ID, and payRate fields, also update the nOfObjs member 8 public member methods (mutators, accessors, and other) getNOfObjts() getName() getID() setPayRate() & getPayRate() setHrWorked() & getHrWorked() A method named grossPay that returns employee’s gross pay (=hrWorked * payRate) PayrollOvertime class (the derived class) 2 private fields double overtimePayRate- Initialized in default constructor to 22.5 double overtimeHrWorked - Initialized in default constructor to 0 2 constructors (public) Default constructor (use super() to invoke the Payroll constructor ) A constructor that accepts the employee’s name, ID, and pay rate, over time pay rate as arguments. These values should be assigned to the object’s name, ID, payRate, and overtimePayRate fields. (use super() to invoke the Payroll constructor ) 5 public member methods (mutators, accessors, and other) getOvertimePayRate() & setOvertimeHrWorked (double) getOvertimeHrWorked() & setOvertimePayRate(double) Override the grossPay method of Payroll class, that returns employee’s gross pay (hrWorked * payRate+ overtimePayRate *overtimeHrWorked) ClientClass In main() method, create two PayrollOvertime objects (one use the info inputted by the user and the other use the default constructor); and print the info for each employee Ex: Enter the name, ID, pay rate, overtime pay rate, regular hours and overtime worked of an employee: Linda Smith 1234 20.5 30.25 35 10 Record 1 Name: Linda Smith ID: 1234 Regular pay rate: $ 20.5 Overtime pay rate: $ 30.25 Regular hours worked: 35.0 Overtime hours worked: 10.0 Gross pay: $1020.00 Record 2 Name: John Doe ID: 9999 Regular pay rate: $ 15.0 Overtime pay rate: $ 22.5 Regular hours worked: 40.0 Overtime hours worked: 0.0 Gross pay: $600.00
Create three files:
- Payroll class.java -Base class definition
- PayrollOvertime.jave - Derived class definition
- PayrollOvertimeClient.java - contains main() method
Implement the two user define classes with the following specifications:
Payroll class (the base class)
-
5 data fields(protected)
- String name - Initialized in default constructor to "John Doe"
- int ID - Initialized in default constructor to 9999
- doulbe payRate - Initialized in default constructor to 15.0
- doulbe hrWorked - Initialized in default constructor to 40
- static int data field named nOfObjs for tracking the number of employees, (initialized to 0 and updated nOfObjs++ in the constructors)
-
2 constructors (public)
- Default constructor (make sure to update the nOfObjs member)
- A constructor that accepts the employee’s name, ID, and pay rate as arguments. These values should be assigned to the object’s name, ID, and payRate fields, also update the nOfObjs member
-
8 public member methods (mutators, accessors, and other)
- getNOfObjts()
- getName()
- getID()
- setPayRate() & getPayRate()
- setHrWorked() & getHrWorked()
- A method named grossPay that returns employee’s gross pay (=hrWorked * payRate)
- getNOfObjts()
PayrollOvertime class (the derived class)
-
2 private fields
- double overtimePayRate- Initialized in default constructor to 22.5
- double overtimeHrWorked - Initialized in default constructor to 0
-
2 constructors (public)
- Default constructor (use super() to invoke the Payroll constructor )
- A constructor that accepts the employee’s name, ID, and pay rate, over time pay rate as arguments.
These values should be assigned to the object’s name, ID, payRate, and overtimePayRate fields. (use super() to invoke the Payroll constructor )
-
5 public member methods (mutators, accessors, and other)
- getOvertimePayRate() & setOvertimeHrWorked (double)
- getOvertimeHrWorked() & setOvertimePayRate(double)
- Override the grossPay method of Payroll class, that returns employee’s gross pay (hrWorked * payRate+ overtimePayRate *overtimeHrWorked)
ClientClass In main() method, create two PayrollOvertime objects (one use the info inputted by the user and the other use the default constructor); and print the info for each employee
Ex:
Enter the name, ID, pay rate, overtime pay rate, regular hours and overtime worked of an employee:
Linda Smith
1234
20.5
30.25
35
10
Record 1
Name: Linda Smith
ID: 1234
Regular pay rate: $ 20.5
Overtime pay rate: $ 30.25
Regular hours worked: 35.0
Overtime hours worked: 10.0
Gross pay: $1020.00
Record 2
Name: John Doe
ID: 9999
Regular pay rate: $ 15.0
Overtime pay rate: $ 22.5
Regular hours worked: 40.0
Overtime hours worked: 0.0
Gross pay: $600.00

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

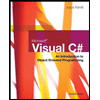
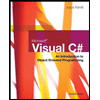