Design a program that will perform critical calculations for a logistics firm. The logistics firm uses two different types of Vehicles; Truck and Ship. Purpose of the program is to display whether or not a transportation vehicle (Truck or Ship) will be able to perform the transportation task. In order to evaluate, your program will check the distance of the route to be taken, the weight of the cargo laden and the total amount of initial fuel the transportation vehicle is initially loaded. If the route, fuel and cargo parameters lead to the conclusion that the vehicle is able to do the trip with no problems, inform the user that the transportation phase does not require any refuels and it can be done in one trip. Otherwise, if the program detects that the vehicle is unable to perform the transportation with the amount of fuel it is loaded in one trip or none at all, inform the user that the vehicle cannot perform the transportation and requires a refuel. Information about the consumption rate of ship and truck are given below. Ship: 60.00 L Fuel used when traveling 100.00 KM Truck: 30.00 L Fuel used when traveling 100.00 KM Whenever a new cargo is added into a vehicle, it's fuel consumption is increased. Weight is entered in tons. Each 1 Ton of weight increases the fuel consumption for the vehicles as follows: Fuel consumption for the ship is increased by 0.50 Fuel consumption for the truck is increased by 1.00 In your program, you will have a base class called Transportation. Transportation class has data members to hold information about the weight of the cargo, distance of the route, initial fuels loaded, fuel consumption and required fuel for completing the route in one trip. The base class is an abstract class. The pure virtual function checkAvailability within the base class has void return type and it is used for checking the availability of the truck/ship to complete the trip or not. Base class also has a member input operator overloading function. This overloaded operator function will first ask the user to enter the weight of the cargo and the distance and assign the values to the variables of cargo weight and distance of the route. Base class (class Transportation) has two derived classes which are called Truck and Ship. These derived classes can reach into all accessible data fields of the base class. Constructor for the class Ship gives an output message “A cargo ship is on hold for current transportation” to the user after that display “Enter the amount for initial fuel: " and assign the value entered by the user to the variable fuel, also assign the default value 60 to the variable fuel consumption as given. Constructor for the class Truck gives an output message “A truck is on hold for current transportation” to the user after that display “Enter the amount for initial fuel: " and assign the value entered by the user to the variable fuel, also assign the default value 30 to the variable fuel consumption as given. After the constructors, override the virtual function checkAvailability in each derived class. This function checks the availability of ship/truck whether it is possible to complete the transportation with the existing amount of fuel according to the distance. It will first calculate the new fuel consumption by processing the laden weight of the cargo and the default fuel consumptions. You will also have to calculate the amount of required fuel within this function. If the amount of fuel is not enough use exception handling. In your try block, you will check if the required fuel is less than or equal to the fuel in the ship/truck. If the condition is satisfied, you will output a message “Sufficient amount of fuel in the ship.” or “Sufficient amount of fuel in truck.” depending on the class. Else (which means vehicle requires more fuel) thrown an exception as (requiredFuel –fuel) for the needed amount of fuel. In the catch block, depending on the class display a message “Transportation Truck/Ship needs to refuel at the station” and the amount of fuel needed.
Addition of Two Numbers
Adding two numbers in programming is essentially the same as adding two numbers in general arithmetic. A significant difference is that in programming, you need to pay attention to the data type of the variable that will hold the sum of two numbers.
C++
C++ is a general-purpose hybrid language, which supports both OOPs and procedural language designed and developed by Bjarne Stroustrup. It began in 1979 as “C with Classes” at Bell Labs and first appeared in the year 1985 as C++. It is the superset of C programming language, because it uses most of the C code syntax. Due to its hybrid functionality, it used to develop embedded systems, operating systems, web browser, GUI and video games.
Design a program that will perform critical calculations for a logistics firm. The
logistics firm uses two different types of Vehicles; Truck and Ship.
Purpose of the program is to display whether or not a transportation vehicle (Truck or
Ship) will be able to perform the transportation task.
In order to evaluate, your program will check the distance of the route to be taken, the weight of
the cargo laden and the total amount of initial fuel the transportation vehicle is initially loaded.
If the route, fuel and cargo parameters lead to the conclusion that the vehicle is able to do the trip
with no problems, inform the user that the transportation phase does not require any refuels and it
can be done in one trip. Otherwise, if the program detects that the vehicle is unable to perform the
transportation with the amount of fuel it is loaded in one trip or none at all, inform the user that
the vehicle cannot perform the transportation and requires a refuel.
Information about the consumption rate of ship and truck are given below.
Ship: 60.00 L Fuel used when traveling 100.00 KM
Truck: 30.00 L Fuel used when traveling 100.00 KM
Whenever a new cargo is added into a vehicle, it's fuel consumption is increased. Weight is
entered in tons. Each 1 Ton of weight increases the fuel consumption for the vehicles as follows:
Fuel consumption for the ship is increased by 0.50
Fuel consumption for the truck is increased by 1.00
In your program, you will have a base class called Transportation. Transportation class
has data members to hold information about the weight of the cargo, distance of the route, initial
fuels loaded, fuel consumption and required fuel for completing the route in one trip.
The base class is an abstract class. The pure virtual function checkAvailability within the base
class has void return type and it is used for checking the availability of the truck/ship to complete
the trip or not.
Base class also has a member input operator overloading function. This overloaded operator
function will first ask the user to enter the weight of the cargo and the distance and assign the
values to the variables of cargo weight and distance of the route.
Base class (class Transportation) has two derived classes which are called Truck and Ship. These
derived classes can reach into all accessible data fields of the base class.
Constructor for the class Ship gives an output message “A cargo ship is on hold for current
transportation” to the user after that display “Enter the amount for initial fuel: " and assign the
value entered by the user to the variable fuel, also assign the default value 60 to the variable fuel
consumption as given.
Constructor for the class Truck gives an output message “A truck is on hold for current
transportation” to the user after that display “Enter the amount for initial fuel: " and assign the
value entered by the user to the variable fuel, also assign the default value 30 to the variable fuel
consumption as given.
After the constructors, override the virtual function checkAvailability in each derived class. This
function checks the availability of ship/truck whether it is possible to complete the transportation
with the existing amount of fuel according to the distance. It will first calculate the new fuel
consumption by processing the laden weight of the cargo and the default fuel consumptions. You
will also have to calculate the amount of required fuel within this function.
If the amount of fuel is not enough use exception handling. In your try block, you will check if the
required fuel is less than or equal to the fuel in the ship/truck. If the condition is satisfied, you will
output a message “Sufficient amount of fuel in the ship.” or “Sufficient amount of fuel in truck.”
depending on the class.
Else (which means vehicle requires more fuel) thrown an exception as (requiredFuel –fuel) for the
needed amount of fuel. In the catch block, depending on the class display a message
“Transportation Truck/Ship needs to refuel at the station” and the amount of fuel needed.



Step by step
Solved in 3 steps with 3 images

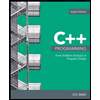
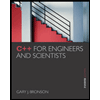
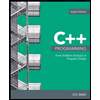
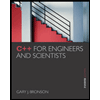