// Airline.cpp - This program determines if an airline passenger is // eligible for a 25% discount. #include #include using namespace std; int main() { string passengerFirstName = ""; // Passenger's first name string passengerLastName = ""; // Passenger's last name int passengerAge = 0; // Passenger's age // This is the work done in the housekeeping() function cout << "Enter passenger's first name: "; cin >> passengerFirstName; cout << "Enter passenger's last name: "; cin >> passengerLastName; cout << "Enter passenger's age: "; cin >> passengerAge; // This is the work done in the detailLoop() function // Test to see if this customer is eligible for a 25% discount // This is the work done in the endOfJob() function return 0; }
// Airline.cpp - This program determines if an airline passenger is // eligible for a 25% discount. #include #include using namespace std; int main() { string passengerFirstName = ""; // Passenger's first name string passengerLastName = ""; // Passenger's last name int passengerAge = 0; // Passenger's age // This is the work done in the housekeeping() function cout << "Enter passenger's first name: "; cin >> passengerFirstName; cout << "Enter passenger's last name: "; cin >> passengerLastName; cout << "Enter passenger's age: "; cin >> passengerAge; // This is the work done in the detailLoop() function // Test to see if this customer is eligible for a 25% discount // This is the work done in the endOfJob() function return 0; }
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter2: Basic Elements Of C++
Section: Chapter Questions
Problem 5PE
Related questions
Question
// Airline.cpp - This program determines if an airline passenger is
// eligible for a 25% discount.
#include <iostream>
#include <string>
using namespace std;
int main()
{
string passengerFirstName = ""; // Passenger's first name
string passengerLastName = ""; // Passenger's last name
int passengerAge = 0; // Passenger's age
// This is the work done in the housekeeping() function
cout << "Enter passenger's first name: ";
cin >> passengerFirstName;
cout << "Enter passenger's last name: ";
cin >> passengerLastName;
cout << "Enter passenger's age: ";
cin >> passengerAge;
// This is the work done in the detailLoop() function
// Test to see if this customer is eligible for a 25% discount
// This is the work done in the endOfJob() function
return 0;
}
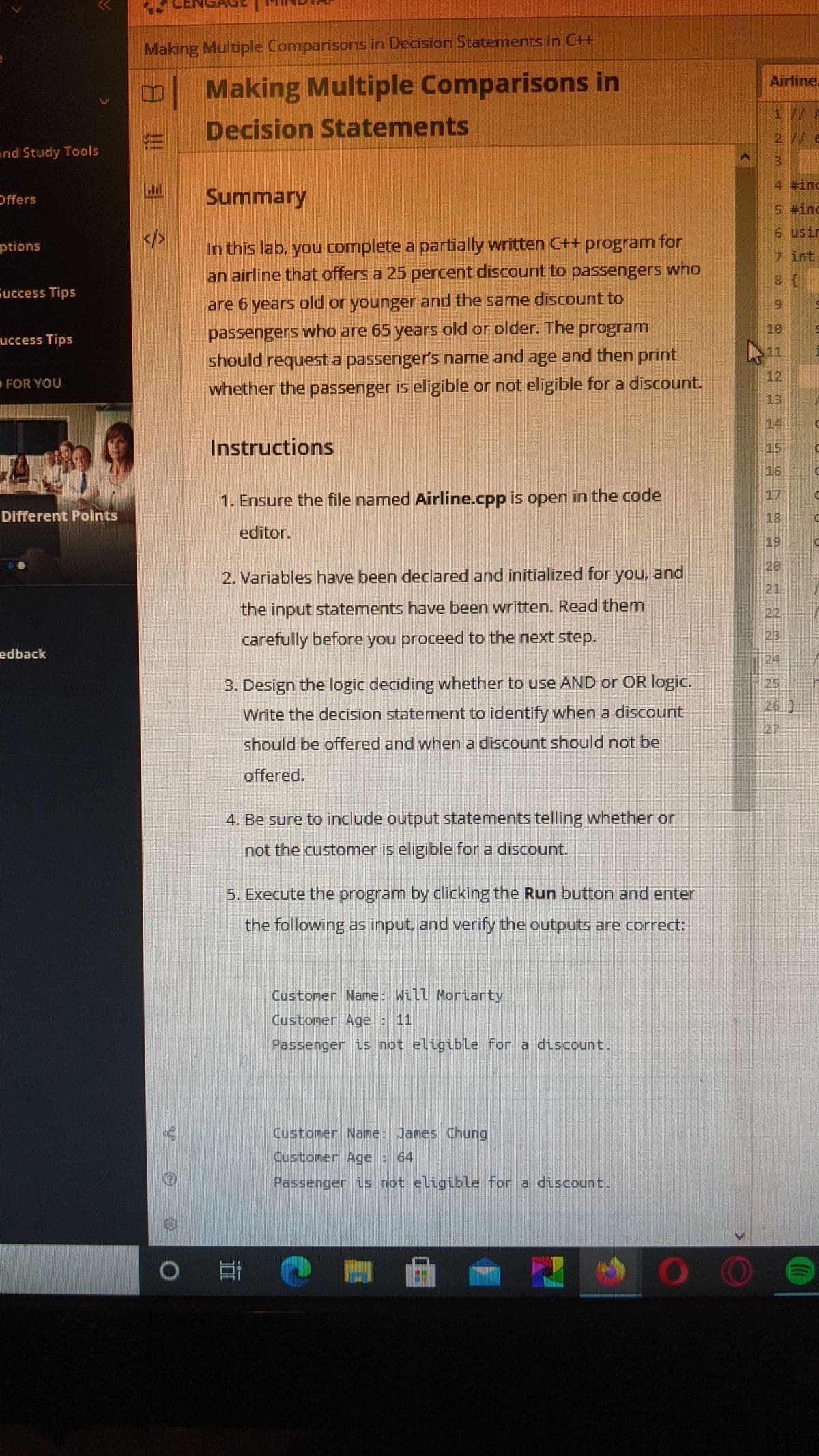
Transcribed Image Text:Making Multiple Comparisons in Decision Statements in C+
Making Multiple Comparisons in
Decision Statements
Airline.
1// A
2 // e
nd Study Tools
3.
4 #inc
Offers
Summary
5 #ind
6 usir
In this lab, you complete a partially written C++ program for
an airline that offers a 25 percent discount to passengers who
ptions
7 int
Success Tips
are 6 years old or younger and the same discount to
6.
passengers who are 65 years old or older. The program
18
uccess Tips
11
should request a passenger's name and age and then print
12
FOR YOU
whether the passenger is eligible or not eligible for a discount.
13
14
Instructions
15
16
17
1. Ensure the file named Airline.cpp is open in the code
Different Points
18
editor.
19
20
2. Variables have been declared and initialized for
you,
and
21
the input statements have been written. Read them
22
carefully before you proceed to the next step.
23
edback
24
3. Design the logic deciding whether to use AND or OR logic.
25
26 }
Write the decision statement to identify when a discount
27
should be offered and when a discount should not be
offered.
4. Be sure to include output statements telling whether or
not the customer is eligible for a discount.
5. Execute the program by clicking the Run button and enter
the following as input, and verify the outputs are correct:
Customer Name: Will Moriarty
Customer Age : 11
Passenger is not eligiblLe for a discount.
Customer Name: James Chung
Customer Age : 64
Passenger is not eligible for a discount.
OO
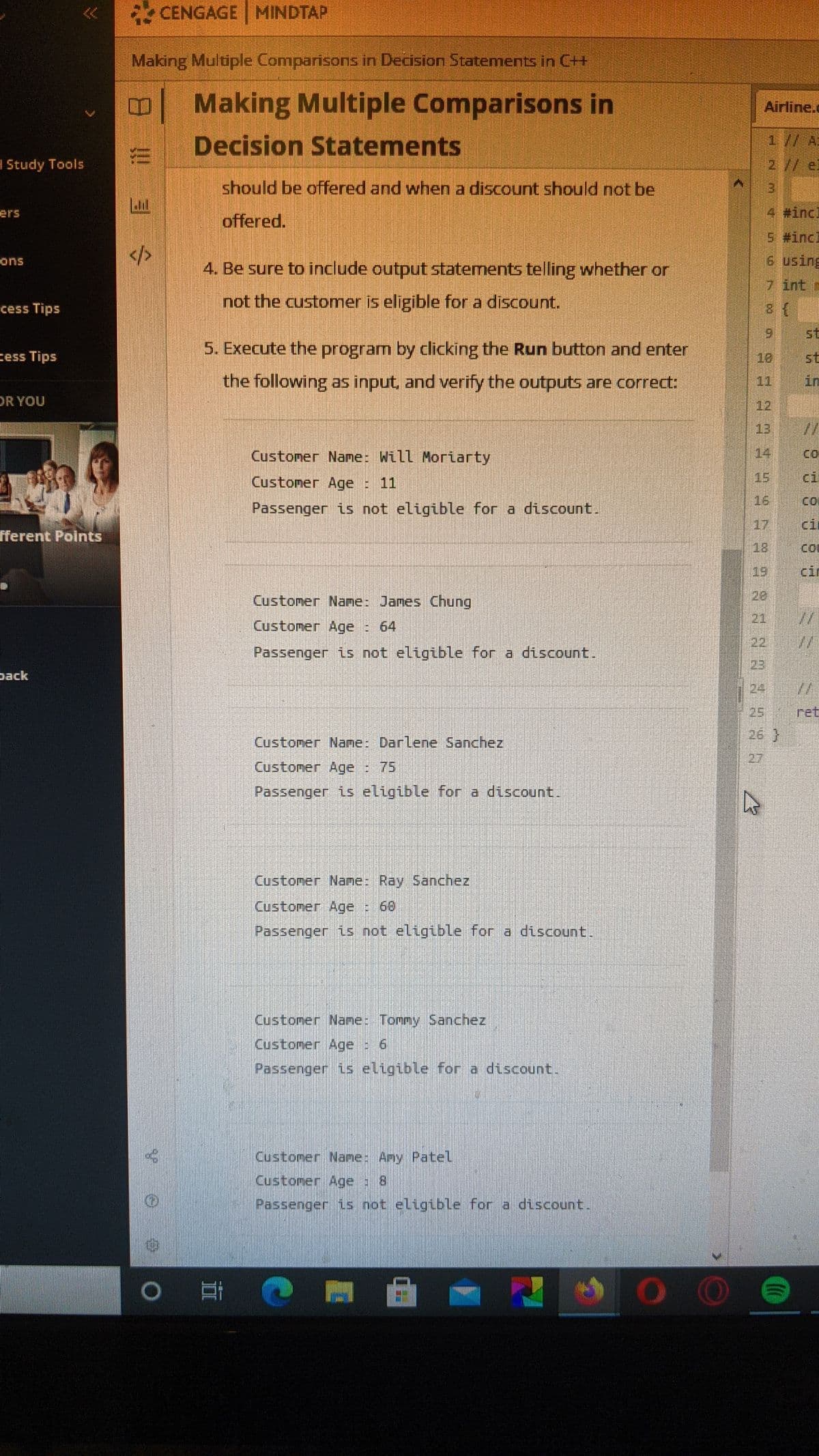
Transcribed Image Text:CENGAGE MINDTAP
Making Multiple Comparisons in Decision Statements in C++
Making Multiple Comparisons in
Airline.c
Decision Statements
1// A
Study Tools
2 // el
should be offered and when a discount should not be
3.
ers
4 #incl
offered.
5 #inc)
ons
4. Be sure to include output statements telling whether or
6 using
7 int
not the customer is eligible for a discount.
cess Tips
st
5. Execute the program by clicking the Run button and enter
=ess Tips
10
st
the following as input, and verify the outputs are correct:
11
in
OR YOU
12
13
//
Customer Name: Will Moriarty
14
co
Customer Age : 11
15
ci
16
co
Passenger is not eligible for a discount.
17
ci
fferent Points
18
COL
19
cir
Customer Name: James Chung
28
21
Customer Age : 64
22
Passenger is not eligible for a discount.
23
pack
24
25
ret
Customer Name: Darlene Sanchez
26 }
27
Customer Age : 75
Passenger is eligible for a discount.
Customer Name: Ray Sanchez
Customer Age : 60
Passenger is not eligible for a discount.
Customer Name: Tommy Sanchez
Customer Age : 6
Passenger is eligible for a discount.
Customer Nane: Amy Patel
Customer Age : 8
Passenger is not eligible for a discount.
日 !
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
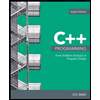
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
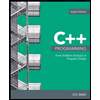
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning