// Airline.cpp - This program determines if an airline passenger is // eligible for a 25% discount. #include #include using namespace std; int main() { string passengerFirstName = ""; // Passenger's first name string passengerLastName = ""; // Passenger's last name int passengerAge = 0; // Passenger's age // This is the work done in the housekeeping() function cout << "Enter passenger's first name: "; cin >> passengerFirstName; cout << "Enter passenger's last name: "; cin >> passengerLastName; cout << "Enter passenger's age: "; cin >> passengerAge; // This is the work done in the detailLoop() function // Test to see if this customer is eligible for a 25% discount // This is the work done in the endOfJob() function return 0;
// Airline.cpp - This program determines if an airline passenger is // eligible for a 25% discount. #include #include using namespace std; int main() { string passengerFirstName = ""; // Passenger's first name string passengerLastName = ""; // Passenger's last name int passengerAge = 0; // Passenger's age // This is the work done in the housekeeping() function cout << "Enter passenger's first name: "; cin >> passengerFirstName; cout << "Enter passenger's last name: "; cin >> passengerLastName; cout << "Enter passenger's age: "; cin >> passengerAge; // This is the work done in the detailLoop() function // Test to see if this customer is eligible for a 25% discount // This is the work done in the endOfJob() function return 0;
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter2: Basic Elements Of C++
Section: Chapter Questions
Problem 5PE
Related questions
Question
// Airline.cpp - This program determines if an airline passenger is
// eligible for a 25% discount.
#include <iostream>
#include <string>
using namespace std;
int main()
{
string passengerFirstName = ""; // Passenger's first name
string passengerLastName = ""; // Passenger's last name
int passengerAge = 0; // Passenger's age
// This is the work done in the housekeeping() function
cout << "Enter passenger's first name: ";
cin >> passengerFirstName;
cout << "Enter passenger's last name: ";
cin >> passengerLastName;
cout << "Enter passenger's age: ";
cin >> passengerAge;
// This is the work done in the detailLoop() function
// Test to see if this customer is eligible for a 25% discount
// This is the work done in the endOfJob() function
return 0;
}
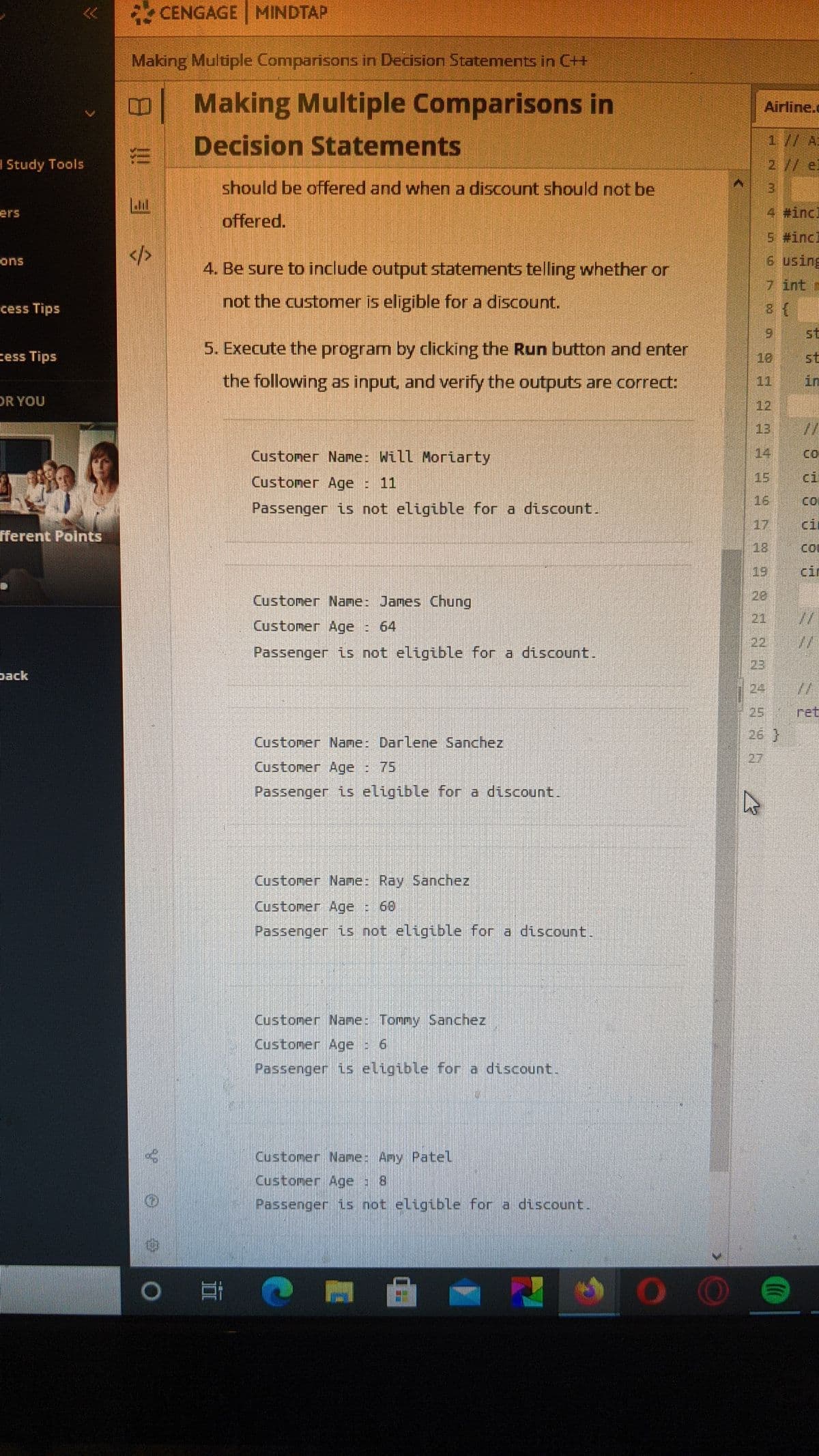
Transcribed Image Text:2 CENGAGE MINDTAP
Making Multiple Comparisons in Decision Statements in C++
Making Multiple Comparisons in
Airline.c
Decision Statements
1// Ai
2// el
Study Tools
should be offered and when a discount should not be
4 #incl
ers
offered.
s #incl
6 using
ons
4. Be sure to include output statements telling whether or
7 int =
cess Tips
not the customer is eligible for a discount.
9 st
5. Execute the program by clicking the Run button and enter
cess Tips
st
in
10
the following as input, and verify the outputs are correct:
11
DR YOU
12
13
Customer Name: Will Moriarty
14
Custoner Age : 11
15
ci
Passenger is not eligible for a discount.
16
co
17
ci
fferent Points
18
coD
19
cir
Customer Name: James Chung
20
21
Custoner Age : 64
22
Passenger is not eligible for a discount.
23
pack
24
//
ret
26)
25
Custoner Nane: Darlene Sanchez
27
Customer Age : 75
Passenger is eligible for a discount.
Customer Nane: Ray Sanchez
Customer Age : 60
Passenger is not eligible for a discount
Custoner Name: Tommy Sanchez
Customer Age 6
Passenger is eligible for a discount.
Customer Nane: Amy Patel
Custoner Age 8
Passenger is not eligible for a discount.
0耳
ヨ令
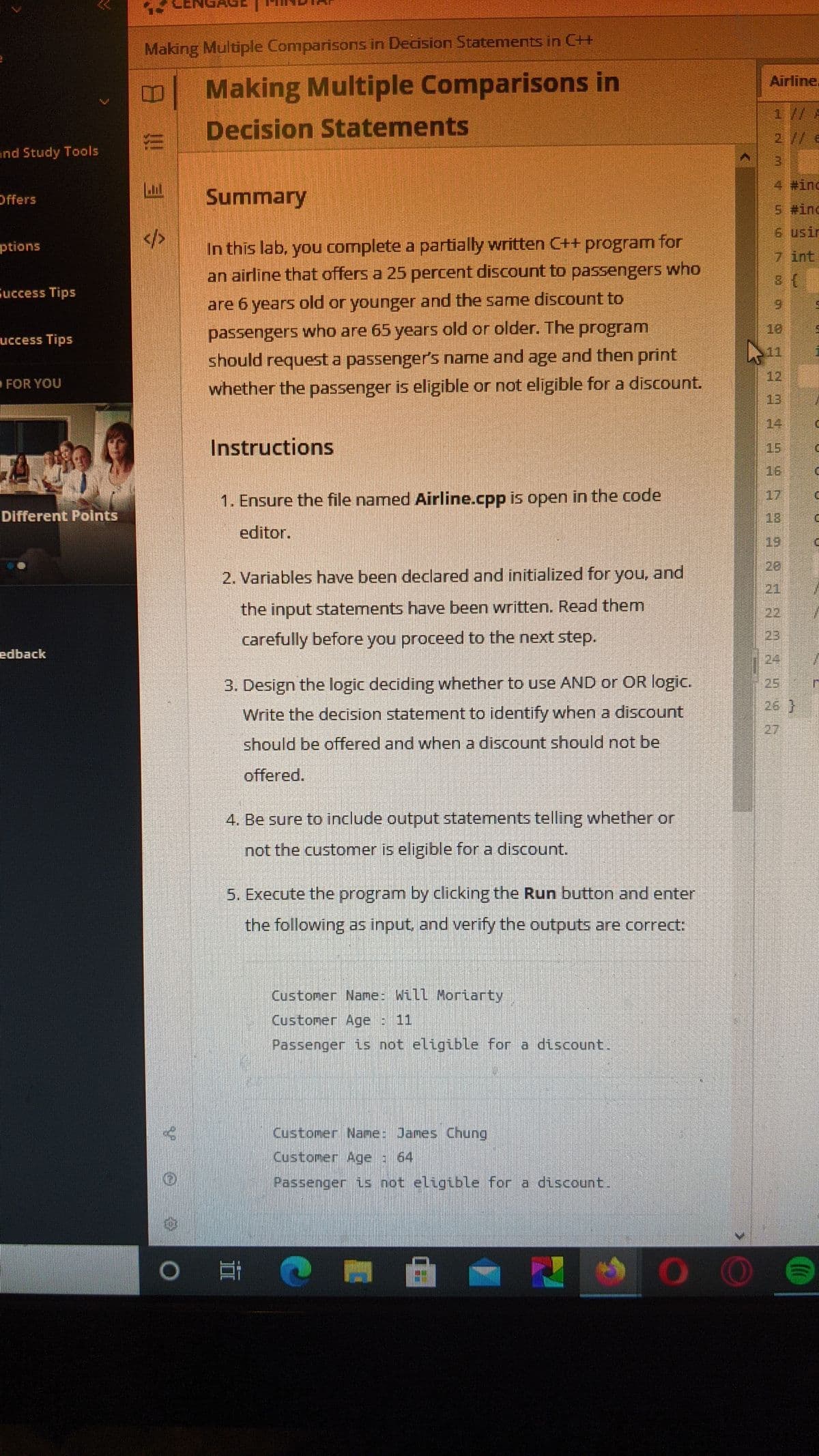
Transcribed Image Text:Making Multiple Comparisons in Decision Statements in C++
Airline,
Making Multiple Comparisons in
1// F
Decision Statements
2//e
and Study Tools
4 #inc
Summary
Offers
5 #inc
6 usir
In this lab, you complete a partially written C++ program for
an airline that offers a 25 percent discount to passengers who
ptions
7 int
uccess Tips
are 6 years old or younger and the same discount to
who are 65 years old or older. The program
passengers
18
uccess Tips
should request a passenger's name and age and then print
12
OFOR YOU
whether the passenger is eligible or not eligible for a discount.
13
14
Instructions
15
16
1. Ensure the file named Airline.cpp is open in the code
17
Different Points
18
editor.
19
20
2. Variables have been declared and initialized for you, and
21
the input statements have been written. Read them
[22
carefully before you proceed to the next step.
23,
edback
24
3. Design the logic deciding whether to use AND or OR logic.
25
26}
Write the decision statement to identify when a discount
27
should be offered and when a discount should not be
offered.
4. Be sure to include output statements telling whether or
not the customer is eligible for a discount.
5. Execute the program by clicking the Run button and enter
the following as input, and verify the outputs are correct:
Customer Nane: Will Moriarty
Custoner Age : 11
Passenger is not eligible for a discount.
Customer Nane: James Chung
Customer Age 64
Passenger is not eligible for a discount.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
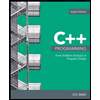
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
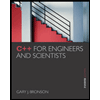
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
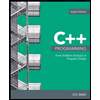
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
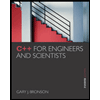
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr