Enhanced Test Scores application JavaScript Test Scores application Thanks for using the Array enhanced Test Scores application. © INF286 Modify the code above so that the application gets the highest score in the array and displays it below the average score in the alert dialog box: Declare a variable named highestScore at the start of the script that will be used to store the highest score. Its starting value should be zero. Add a second for loop right before the alert statement at the end of the script. This for loop should be executed once for each score in the array. Within the loop, an if statement should replace the value in the highestScore variable with the current score if that score is greater than the value in highestScore. That way, the highestScore variable will eventually store the highest score in the array. When you’ve got that working, comment out the second for loop. Then, modify the first for loop so it not only sums the scores but also puts the highest score in the highestScore variable. Modify the alert statement that follows the for loop so it displays both the average score and highest score. Problem 2: Converting Fahrenheit to Celsius Please create a JavaScript application, named as “convert_temps.html”, that converts Fahrenheit temperatures to Celsius temperatures: Use the prompt() method to ask user to input a Fahrenheit value. Convert Fahrenheit to Celsius, first subtract 32 from the Fahrenheit temperature. Then, multiply that result by 5/9. (That is c = (f-32) * 5/9). Add data validation to the application so it won’t do the conversion until the user enters a Fahrenheit temperature between -100 and 212. If the entry is invalid, an alert dialog box should be displayed. Add a loop to the code so the user can do a series of calculations without restarting the application. To end the application, the user must enter 999 as the temperature.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Enhanced Test Scores application</title>
<script>
var total = 0;
var entry;
var scores = [];
var show = "The test scores:\n";
var average;
// use a do-while loop to put the scores in an array
do {
entry = prompt("Enter test score\n" +
"Or enter 999 to end entries", 999);
entry = parseInt(entry);
if (entry >= 0 && entry <= 100) {
scores[scores.length] = entry;
}
else if (entry != 999){
alert("Entry must be a valid number from 0 " +
"through 100\nOr enter 999 to end entries");
}
}while (entry != 999);
// calculate the total of the scores and the average of the scores.
if (scores.length != 0) {
// use a for loop to process the scores
for (var i = 0; i < scores.length; i++) {
total = total + scores[i]; // both are numbers so adds
show = show + scores[i] + "\n"; // strings & number so concatenates
}
// calculate the average and display
average = parseInt(total/scores.length);
alert(show + "\nAverage score is " + average);
}
</script>
</head>
<body>
<header>
<h1>JavaScript Test Scores application</h1>
</header>
<main>
Thanks for using the Array enhanced Test Scores application.
</main>
<footer>
<p>© INF286 </p>
</footer>
</body>
</html>
Modify the code above so that the application gets the highest score in the array and displays it below the average score in the alert dialog box:
- Declare a variable named highestScore at the start of the script that will be used to store the highest score. Its starting value should be zero.
- Add a second for loop right before the alert statement at the end of the script. This for loop should be executed once for each score in the array. Within the loop, an if statement should replace the value in the highestScore variable with the current score if that score is greater than the value in highestScore. That way, the highestScore variable will eventually store the highest score in the array. When you’ve got that working, comment out the second for loop. Then, modify the first for loop so it not only sums the scores but also puts the highest score in the highestScore variable.
- Modify the alert statement that follows the for loop so it displays both the average score and highest score.
Problem 2: Converting Fahrenheit to Celsius
Please create a JavaScript application, named as “convert_temps.html”, that converts Fahrenheit temperatures to Celsius temperatures:
- Use the prompt() method to ask user to input a Fahrenheit value.
- Convert Fahrenheit to Celsius, first subtract 32 from the Fahrenheit temperature. Then, multiply that result by 5/9. (That is c = (f-32) * 5/9).
- Add data validation to the application so it won’t do the conversion until the user enters a Fahrenheit temperature between -100 and 212. If the entry is invalid, an alert dialog box should be displayed.
- Add a loop to the code so the user can do a series of calculations without restarting the application. To end the application, the user must enter 999 as the temperature.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

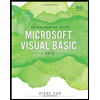
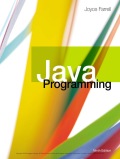
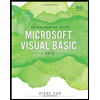
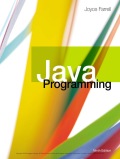