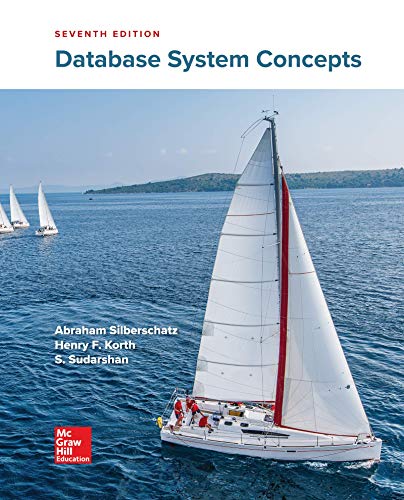
Write a loop that finds the smallest value. Print it and the index
code format:
#include <iostream>
using namespace std;
#include <cstdlib> // required for rand()
int main()
{
// Put Part2 code here
// Declare smallestFoundSoFar
// Q: what should be its initial value?
int indexOfSmallest = -1;// sign that it is not initialized
Part 2 code
#include <iostream>
using namespace std;
// part 1 code
#include <cstdlib>
int main()
{
srand(17);
const int ARRAYSIZE = 20; // size for the array
int RandArray[ARRAYSIZE]; // array declared
int i; // to iterate the loop
// this loop will store thei random number in the array
for (i = 0; i < ARRAYSIZE; i++)
RandArray[i] = rand() % 100;
// this loop will print the array
for (i = 0; i < ARRAYSIZE; i++)
cout <<"randArray["<< i <<"]=" << RandArray[i] << endl;
int largestFoundSoFar=-1; // anything is larger than this!
int indexOfLargest = -1; // sign that it is not initialized
for (i = 0; i < ARRAYSIZE; i++)
{
if (RandArray[i] > largestFoundSoFar)
{
largestFoundSoFar=RandArray[i];
indexOfLargest = i;
}
}
cout << "\nlargestFoundSoFar=" << largestFoundSoFar << " at index " << indexOfLargest;
return 0;
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- #include #include #include struct Point2d { double x; double y; void print() { // example: (2.5,3.64) std::cout << "(" << x << "," << y << ")" << std::endl; } double length() const { return std::sqrt(x * x + y * y); } // const Point2D & ==> function can't modify 'other' // the second const ==> function can't modify x, y Point2d add(const Point2d &other) const { Point2d result; result.x = x + other.x; result.y = y + other.y; return result; } }; // // do NOT modify above this line // Point2d discplacement(std::string commands) { // TODO: re-write this Point2d p = {0, 0}; return p; } // // do NOT modify below this line // void check(Point2d result, double x , double y) { if (result.x != x || result.y != y) { std::cout << "fail" << std::endl; } else { std::cout << "pass" << std::endl; } } int main() { Point2d result =…arrow_forward7bact2 Please help me answer this in python programming.arrow_forward[2, 4, 5, 8] input () while user_input != 'end': numbers = user_input try: = #Possible ValueError diviforint (user_input) if divisor > 20: #Possible NameError # compute() is not defined result = compute (result) elif divisor < 0: # Possible IndexError result = else: print ('OK') numbers [divisor] # Possible ZeroDivisionError result = 20 // divisor print (result, end-' ') except (ValueError, ZeroDivisionError): print ('r', end=' ') except (NameError, IndexError) : print ('s', end=' ') input () user input Type the program's output = # // truncates to an integer Input nine -10 0 2 -2 95 end Output 0arrow_forward
- // MichiganCities.cpp - This program prints a message for invalid cities in Michigan. // Input: Interactive // Output: Error message or nothing #include <iostream> #include <string> using namespace std; int main() { // Declare variables string inCity; // name of city to look up in array const int NUM_CITIES = 10; // Initialized array of cities string citiesInMichigan[] = {"Acme", "Albion", "Detroit", "Watervliet", "Coloma", "Saginaw", "Richland", "Glenn", "Midland", "Brooklyn"}; bool foundIt = false; // Flag variable int x; // Loop control variable // Get user input cout << "Enter name of city: "; cin >> inCity; // Write your loop here // Write your test statement here to see if there is // a match. Set the flag to true if city is found. // Test to see if city was not found to determine if // "Not a city in Michigan" message should be printed.…arrow_forward#include <iostream>#include <string.h>using namespace std;int index1(char *T,char *P){int t=0,p=0,i,j,r;t = strlen(T);p = strlen(P);i=0;int max = t - p + 1;while(i<max){for(j=0;j<p;j++){if(P[j]==T[i+j]){r = i;break;}else r = -1;}i++;}return r;} int main(){ int l;char c[100];char d[100];cout<<"Enter First String\n"; cin>>c;cout<<"Enter Second String\n";cin>>d;l = index1(c,d);cout<<l; return 0;} Note: Remove Funcationarrow_forwardC++arrow_forward
- #include <stdio.h>#include <stdlib.h> typedef struct Number_struct { int num;} Number; void Swap(Number* numPtr1, Number* numPtr2) { /* Your code goes here */} int main(void) { Number* num1 = NULL; Number* num2 = NULL; num1 = (Number*)malloc(sizeof(Number)); num2 = (Number*)malloc(sizeof(Number)); int int1; int int2; scanf("%d", &int1); scanf("%d", &int2); Thank you so much but i forgot to put this how i would fit in into this. num1->num = int1; num2->num = int2; Swap(num1, num2); printf("num1 = %d, num2 = %d\n", num1->num, num2->num); return 0;}arrow_forwardstatic List<String> findWinners(String pattern, int minLength, boolean even, Stream<String> stream) This function will receive a stream of strings and it will return a sorted list of strings that meet all of the following criteria: They must contain the pattern The strings length must be equal to or greater than the min length number The strings length must be an even or odd number This Function must take no longer 10000 milliseconds to return. pattern - A pattern that all the strings in the list returned must containminLength - The length that all the strings in the list returned must be equal to or greater thaneven - True if even false if odd. Denotes a condition that all the strings in the list returned must have a length that is even or odd.stream - A stream of strings to be filteredreturn - A list of strings that meets the criteria outlined above sorted by their length from small to largearrow_forward/*Line 1:*/ doublevalues[6] = {10, 20, 30, 40, 50, 60}; /*Line 2:*/ double* yalptr= values; /*Line 3:*/ cout << 3*values[1] + yalptr[3] + *(valptr + 2); A. What is 3*values[1] equal to in Line 3? (Explain) B. What is yalptr[3] equal to in Line 3? (Explain)arrow_forward
- main.cc file #include <iostream>#include <memory> #include "customer.h" int main() { // Creates a line of customers with Adele at the front. // LinkedList diagram: // Adele -> Kehlani -> Giveon -> Drake -> Ruel std::shared_ptr<Customer> ruel = std::make_shared<Customer>("Ruel", 5, nullptr); std::shared_ptr<Customer> drake = std::make_shared<Customer>("Drake", 8, ruel); std::shared_ptr<Customer> giveon = std::make_shared<Customer>("Giveon", 2, drake); std::shared_ptr<Customer> kehlani = std::make_shared<Customer>("Kehlani", 15, giveon); std::shared_ptr<Customer> adele = std::make_shared<Customer>("Adele", 4, kehlani); std::cout << "Total customers waiting: "; // =================== YOUR CODE HERE =================== // 1. Print out the total number of customers waiting // in line by invoking TotalCustomersInLine. //…arrow_forward/* yahtC */ #include <stdio.h> #include <string.h> #include <stdlib.h> #include <time.h> void seed(int argc, char ** argv){ srand(time(NULL)); // Initialize random seed if (argc>1){ int i; if (1 == sscanf(argv[1],"%d",&i)){ srand(i); } } } void instructions(){ printf("\n\n\n\n" "\t**********************************************************************\n" "\t* Welcome to YahtC *\n" "\t**********************************************************************\n" "\tYahtC is a dice game (very) loosely modeled on Yahtzee\n" "\tBUT YahtC is not Yahtzee.\n\n" "\tRules:\n" "\t5 dice are rolled\n" "\tThe user selects which dice to roll again.\n" "\tThe user may choose to roll none or all 5 or any combination.\n" "\tAnd then the user selects which dice to roll, yet again.\n" "\tAfter this second reroll the turn is scored.\n\n" "\tScoring is as follows:\n" "\t\t*\t50 points \t5 of a kind scores 50 points.\n" "\t\t*\t45 points \tNo pairs (all unique) scores 45…arrow_forward3. int count(10); while(count >= 0) { count -= 2; std::cout << count << endl;arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
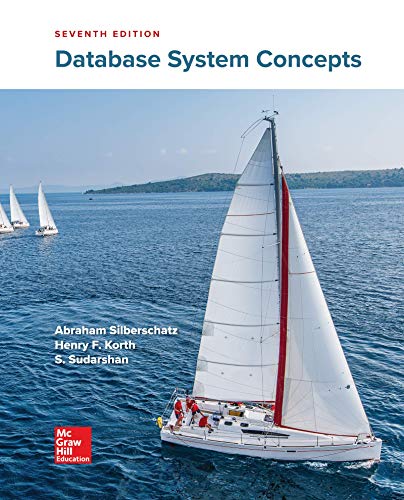
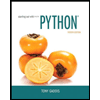
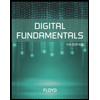
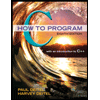
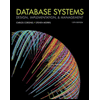
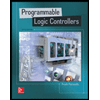