Exercise 9.2 A student has found a summer job in a painting company. He is to estimate the amount of paint that the company needs to paint rectangular fields of different sizes. On average, a) 0.2 kg (kilogram) of paint is needed to paint a one square meter area (0.2 kg/m2). b) 22 mg (milligram) of paint is needed to paint a one square centimetres area (22 mg/cm2). c) 19.6 T (Ton) of paint is needed to paint a one square km area (19.6 T/km2). Use the below program to estimate the amount of paint required to paint a rectangle with the length and width that the user will enter from the keyboard. You need to compute the amount of paint in three different units (kg, mg, and Ton). Use the below program to estimate the amount of paint required to paint a rectangle with the length and width that the user will enter from the keyboard. You need to compute the amount of paint in three different units (kg, mg, and Ton). // P92.cpp - This program illustrates the use of namespaces #include using namespace std; namespace cm { double area(double length, double width); } namespace meter { double area(double length, double width); } double area_km(double length, double width); int main ( ) { double length, width; // dimension of a rectangle double A; // area of a rectangle cout << "Enter the length and the width of the rectangle. \n"; cout << "Assuming unit is meter \n"; cin >> length >> width; { using namespace cm; A = area(length, width); cout << "Area is: " << A << endl; } { using namespace meter; A = area(length, width); cout << "Area is: " << A << endl; } A = area_km(length, width); cout << "Area is: " << A << endl; return 0; } namespace cm { double area(double length, double width) { cout << "From namespace cm, I am sending area in cm^2 back\n"; return (length*100)*(width*100); } } namespace meter { double area(double length, double width) { cout << "From namespace meter, I am sending area in m^2 back\n"; return length*width; } } double area_km(double length, double width) { cout << "From std namespace, I am sending area in km^2 back\n"; return (length/1000)*(width/1000); } In the above program we have asked the user to input the length and width of a rectangle in meters. Then, we have used different name spaces to compute the area of THE rectangle using three different units. As you have noticed, the program that computes the area in namespace cm and meter are both called area, but they perform different computations depending on their definitions in their respective namespace.
Exercise 9.2
A student has found a summer job in a painting company. He is to estimate the amount of paint that the company needs to paint rectangular fields of different sizes. On average, a) 0.2 kg (kilogram) of paint is needed to paint a one square meter area (0.2 kg/m2). b) 22 mg (milligram) of paint is needed to paint a one square centimetres area (22 mg/cm2). c) 19.6 T (Ton) of paint is needed to paint a one square km area (19.6 T/km2). Use the below program to estimate the amount of paint required to paint a rectangle with the length and width that the user will enter from the keyboard. You need to compute the amount of paint in three different units (kg, mg, and Ton).
Use the below program to estimate the amount of paint required to paint a rectangle with the length and width that the user will enter from the keyboard. You need to compute the amount of paint in three different units (kg, mg, and Ton).
// P92.cpp - This program illustrates the use of namespaces
#include<iostream>
using namespace std;
namespace cm
{
double area(double length, double width);
}
namespace meter
{
double area(double length, double width);
}
double area_km(double length, double width);
int main ( )
{
double length, width; // dimension of a rectangle
double A; // area of a rectangle
cout << "Enter the length and the width of the rectangle. \n";
cout << "Assuming unit is meter \n";
cin >> length >> width;
{
using namespace cm;
A = area(length, width);
cout << "Area is: " << A << endl;
}
{
using namespace meter;
A = area(length, width);
cout << "Area is: " << A << endl;
}
A = area_km(length, width);
cout << "Area is: " << A << endl;
return 0;
}
namespace cm
{
double area(double length, double width)
{
cout << "From namespace cm, I am sending area in cm^2 back\n"; return (length*100)*(width*100);
}
}
namespace meter
{
double area(double length, double width)
{
cout << "From namespace meter, I am sending area in m^2 back\n"; return length*width;
}
}
double area_km(double length, double width)
{
cout << "From std namespace, I am sending area in km^2 back\n"; return (length/1000)*(width/1000);
}
In the above program we have asked the user to input the length and width of a rectangle in meters. Then, we have used different name spaces to compute the area of THE rectangle using three different units. As you have noticed, the program that computes the area in namespace cm and meter are both called area, but they perform different computations depending on their definitions in their respective namespace.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

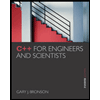
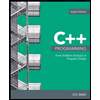
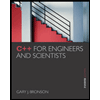
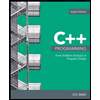