Guidelines are creating a cardlayout with 3 cards: login, fred and bob. import java.awt.event.*; import javax.swing.*; import java.awt.*; import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JPanel; import java.awt.BorderLayout; import java.awt.CardLayout; import java.awt.FlowLayout; import java.awt.Color; import java.awt.Container; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; public class SimpleCardWindow extends JFrame implements ActionListener { public static final int WIDTH = 300; public static final int HEIGHT = 300; private CardLayout theCardsLayoutManager; private JPanel theCardsPanel; private JTextField userTextField; private JTextField passwordTextField; private JButton loginButton; private JButton bobWordButton; private JTextField bobEntryTextField; private JTextArea bobDisplayTextArea; private JButton bobReturnButton; private JPanel fredCard; private JPanel fredButtonPanel; private JButton fredRedColorButton; private JButton fredGreenColorButton; private JButton fredReturnButton; public SimpleCardWindow() { super(); setSize(WIDTH, HEIGHT); setTitle("CardLayout Demonstration"); theCardsPanel = new JPanel(); theCardsLayoutManager = new CardLayout(); theCardsPanel.setLayout(theCardsLayoutManger); //login card// JPanel loginCard = new JPanel(); loginCard.setLayout(new GridLayout(3,1)); userTextField = new JTextField("Username:"); passwordTextField = new JTextField("Password:"); loginButton = new JButton("Login"); loginButton.addActionListener(this); loginCard.add(userTextField); loginCard.add(passwordTextField); loginCard.add(loginButton); theCardsPanel.add("loginCard",loginCard); //bob card// JPanel bobCard = new JPanel(); bobCard.setLayout(new BorderLayout()); bobWordButton = new JButton("Button 1"); bobWordButton.addActionListener(this); bobEntryTextField = new JTextField("Button 2"); bobDisplayTextArea = new JTextArea(5, 20); bobDisplayTextArea.setEditable(false); bobReturnButton = new JButton("Return"); bobReturnButton.addActionListener(this); JPanel bobEntryPanel = new JPanel(); bobEntryPanel.setLayout(new GridLayout(1,2)); bobEntryPanel.add(bobEntryTextField); bobEntryPanel.add(bobWordButton); bobCard.add(bobEntryPanel, BorderLayout.NORTH); bobCard.add(bobDisplayTextArea, BorderLayout.CENTER); bobCard.add(bobReturnButton, BorderLayout.SOUTH); theCardsPanel.add("bobCard", bobCard); //fred card// fredCard = new JPanel(); fredCard.setLayout(new BorderLayout()); fredRedColorButton = new JButton("Button 1"); fredRedColorButton.addActionListener(this); fredGreenColorButton = new JButton("Button 2"); fredGreenColorButton.addActionListener(this); fredReturnButton = new JButton("Button 3"); fredReturnButton.addActionListener(this); fredButtonPanel = new JPanel(); fredButtonPanel.add(fredGreenColorButton); fredButtonPanel.add(fredRedColorButton); fredCard.add(fredButtonPanel, BorderLayout.CENTER); fredCard.add(fredReturnButton, BorderLayout.SOUTH); theCardsPanel.add("fredCard", fredCard); getContentPane().add(theCardsPanel, BorderLayout.CENTER); //setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE); WindowDestroyer listener = new WindowDestroyer(); addWindowListener(listener); } public void actionPerformed(ActionEvent e) { if (e.getActionCommand().equals("Login")) { String id = userTextField.getText(); String pass = passwordTextField.getText(); if (id.equals("Bob") && pass.equals("mubby")) theCardsLayoutManager.show(theCardsPanel, "bobCard"); else if (id.equals("Fred") && pass.equals("goolag")) theCardsLayoutManager.show(theCardsPanel, "fredCard"); } else if (e.getActionCommand().equals("Return")) { theCardsLayoutManager.show(theCardsPanel, "loginCard"); } else if (e.getSource() == bobWordButton) { String add = bobEntryTextField.getText(); System.out.println("word is " + add); bobDisplayTextArea.append(add + "\n"); } else if (e.getSource() == fredGreenColorButton) { fredButtonPanel.setBackground(Color.GREEN); } else if (e.getSource() == fredRedColorButton) { fredButtonPanel.setBackground(Color.RED); } else System.out.println("Error in button interface."); } } I'm receiving this error code: 1) SimpleCardWindow.java:42: error: cannot find symbol theCardsPanel.setLayout(theCardsLayoutManger); ^ symbol: variable theCardsLayoutManger location: class SimpleCardWindow 2) SimpleCardWindow.java:95: error: cannot find symbol WindowDestroyer listener = new WindowDestroyer(); ^ symbol: class WindowDestroyer location: class SimpleCardWindow 3) SimpleCardWindow.java:95: error: cannot find symbol WindowDestroyer listener = new WindowDestroyer(); ^ symbol: class WindowDestroyer location: class SimpleCardWindow
Guidelines are creating a cardlayout with 3 cards: login, fred and bob.
import java.awt.event.*;
import javax.swing.*;
import java.awt.*;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import java.awt.BorderLayout;
import java.awt.CardLayout;
import java.awt.FlowLayout;
import java.awt.Color;
import java.awt.Container;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class SimpleCardWindow extends JFrame implements ActionListener {
public static final int WIDTH = 300;
public static final int HEIGHT = 300;
private CardLayout theCardsLayoutManager;
private JPanel theCardsPanel;
private JTextField userTextField;
private JTextField passwordTextField;
private JButton loginButton;
private JButton bobWordButton;
private JTextField bobEntryTextField;
private JTextArea bobDisplayTextArea;
private JButton bobReturnButton;
private JPanel fredCard;
private JPanel fredButtonPanel;
private JButton fredRedColorButton;
private JButton fredGreenColorButton;
private JButton fredReturnButton;
public SimpleCardWindow() {
super();
setSize(WIDTH, HEIGHT);
setTitle("CardLayout Demonstration");
theCardsPanel = new JPanel();
theCardsLayoutManager = new CardLayout();
theCardsPanel.setLayout(theCardsLayoutManger);
//login card//
JPanel loginCard = new JPanel();
loginCard.setLayout(new GridLayout(3,1));
userTextField = new JTextField("Username:");
passwordTextField = new JTextField("Password:");
loginButton = new JButton("Login");
loginButton.addActionListener(this);
loginCard.add(userTextField);
loginCard.add(passwordTextField);
loginCard.add(loginButton);
theCardsPanel.add("loginCard",loginCard);
//bob card//
JPanel bobCard = new JPanel();
bobCard.setLayout(new BorderLayout());
bobWordButton = new JButton("Button 1");
bobWordButton.addActionListener(this);
bobEntryTextField = new JTextField("Button 2");
bobDisplayTextArea = new JTextArea(5, 20);
bobDisplayTextArea.setEditable(false);
bobReturnButton = new JButton("Return");
bobReturnButton.addActionListener(this);
JPanel bobEntryPanel = new JPanel();
bobEntryPanel.setLayout(new GridLayout(1,2));
bobEntryPanel.add(bobEntryTextField);
bobEntryPanel.add(bobWordButton);
bobCard.add(bobEntryPanel, BorderLayout.NORTH);
bobCard.add(bobDisplayTextArea, BorderLayout.CENTER);
bobCard.add(bobReturnButton, BorderLayout.SOUTH);
theCardsPanel.add("bobCard", bobCard);
//fred card//
fredCard = new JPanel();
fredCard.setLayout(new BorderLayout());
fredRedColorButton = new JButton("Button 1");
fredRedColorButton.addActionListener(this);
fredGreenColorButton = new JButton("Button 2");
fredGreenColorButton.addActionListener(this);
fredReturnButton = new JButton("Button 3");
fredReturnButton.addActionListener(this);
fredButtonPanel = new JPanel();
fredButtonPanel.add(fredGreenColorButton);
fredButtonPanel.add(fredRedColorButton);
fredCard.add(fredButtonPanel, BorderLayout.CENTER);
fredCard.add(fredReturnButton, BorderLayout.SOUTH);
theCardsPanel.add("fredCard", fredCard);
getContentPane().add(theCardsPanel, BorderLayout.CENTER);
//setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE);
WindowDestroyer listener = new WindowDestroyer();
addWindowListener(listener);
}
public void actionPerformed(ActionEvent e) {
if (e.getActionCommand().equals("Login")) {
String id = userTextField.getText();
String pass = passwordTextField.getText();
if (id.equals("Bob") && pass.equals("mubby"))
theCardsLayoutManager.show(theCardsPanel, "bobCard");
else if (id.equals("Fred") && pass.equals("goolag"))
theCardsLayoutManager.show(theCardsPanel, "fredCard");
}
else if (e.getActionCommand().equals("Return")) {
theCardsLayoutManager.show(theCardsPanel, "loginCard");
}
else if (e.getSource() == bobWordButton) {
String add = bobEntryTextField.getText();
System.out.println("word is " + add);
bobDisplayTextArea.append(add + "\n");
}
else if (e.getSource() == fredGreenColorButton) {
fredButtonPanel.setBackground(Color.GREEN);
}
else if (e.getSource() == fredRedColorButton) {
fredButtonPanel.setBackground(Color.RED);
}
else
System.out.println("Error in button interface.");
}
}
I'm receiving this error code:
1) SimpleCardWindow.java:42: error: cannot find symbol
theCardsPanel.setLayout(theCardsLayoutManger);
^
symbol: variable theCardsLayoutManger
location: class SimpleCardWindow
2) SimpleCardWindow.java:95: error: cannot find symbol
WindowDestroyer listener = new WindowDestroyer();
^
symbol: class WindowDestroyer
location: class SimpleCardWindow
3) SimpleCardWindow.java:95: error: cannot find symbol
WindowDestroyer listener = new WindowDestroyer();
^
symbol: class WindowDestroyer
location: class SimpleCardWindow

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

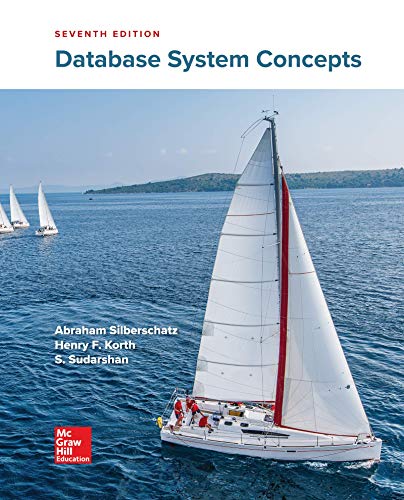
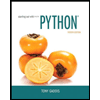
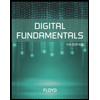
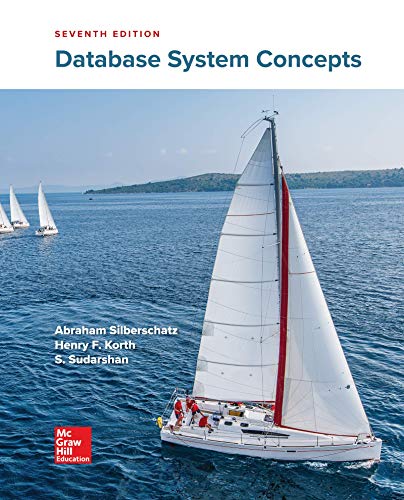
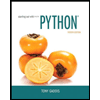
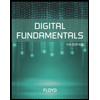
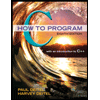
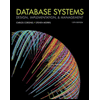
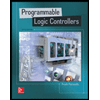