here is my c++ problem I'm working on. I have to use recursion to find all possible anagrams of a word. for example Please enter a string for an anagram: art Matching word art Matching word rat Matching word tar Please enter a string for an anagram: regardless No matches found the txt file consists of words from A to Z about 28,000 words need help finishing this problem. but may not use any of C++'s iteration constructs (do, while, for, and goto) or any STL algorithms #include #include #include #include using namespace std; const int MAXRESULTS = 20; // Max matches that can be found const int MAXDICTWORDS = 30000; // Max words that can be read in int loadWords(istream& dictfile, string dict[]); int recBlends(string word, const string dict[], int size, string results[]); void showResults(const string results[], int size); void printPermutations(string prefix, string rest); void loop(string prefix, string rest, int i, int max); int main() { string results[MAXRESULTS]; string dict[MAXDICTWORDS]; ifstream dictfile; // file containing the list of words int nwords; // number of words read from dictionary string word; dictfile.open("words.txt"); if (!dictfile) { cout <<"file not found!" << endl; return (1); } else cout << "file was open\n"; nwords = loadWords(dictfile, dict); cout << "Please enter a string for an anagram: "; cin >> word; int numMatches = recBlends(word, dict, nwords, results); if (!numMatches) cout << "No matches found" << endl; else showResults(results, numMatches); return 0; } int loadWords(istream& dictfile, string dict[]) { string data; if (dictfile.eof()) { // end of dictfile return 0; } dictfile >> data; // uses recursion to loop to the end of dictfile (dict starts with Z) int x = loadWords(dictfile, dict); if (x < MAXDICTWORDS) { dict[x] = data; x++; return x; } else return MAXDICTWORDS; //reached max capacity of array } void showResults(const string results[], int size) { if (size - 1 < 0) return; cout << "Matching word " << results[size - 1] << endl; showResults(results, size - 1); } int recBlends(string word, const string dict[], int size, string results[]) { int back = word.size() - 1; int matched = 0; //permute(word, 0, back, dict, size, matched, results); return matched; } void printPermutations(string prefix, string rest) { //if rest is empty, printing prefix if (rest.length() == 0) { cout << prefix << endl; } else { //otherwise passing prefix and rest to loop, loop from //0 to rest.length-1 loop(prefix, rest, 0, rest.length()); } } //loop method implementation, to loop for values between i and max-1 void loop(string prefix, string rest, int i, int max) { //if i exceeds or equals max, returning if (i >= max) { return; } //else, appending char at index i to prefix string updated_prefix = prefix + rest[i]; //taking out char at index i from rest to create a string without current char //(this is done by concatenating string before index i with string after index i) string updated_rest = rest.substr(0, i) + rest.substr(i + 1); //calling printPermutations, passing these updated values printPermutations(updated_prefix, updated_rest); //moving to the next iteration of the loop, passing i+1 as new value for i loop(prefix, rest, i
here is my c++ problem I'm working on. I have to use recursion to find all possible anagrams of a word. for example
Please enter a string for an anagram: art
Matching word art
Matching word rat
Matching word tar
Please enter a string for an anagram: regardless
No matches found
the txt file consists of words from A to Z about 28,000 words
need help finishing this problem. but may not use any of C++'s iteration constructs (do, while, for, and goto) or any STL
algorithms
#include <iostream>
#include <fstream>
#include <istream>
#include <cstring>
using namespace std;
const int MAXRESULTS = 20; // Max matches that can be found
const int MAXDICTWORDS = 30000; // Max words that can be read in
int loadWords(istream& dictfile, string dict[]);
int recBlends(string word, const string dict[], int size, string
results[]);
void showResults(const string results[], int size);
void printPermutations(string prefix, string rest);
void loop(string prefix, string rest, int i, int max);
int main()
{
string results[MAXRESULTS];
string dict[MAXDICTWORDS];
ifstream dictfile; // file containing the list of words
int nwords; // number of words read from dictionary
string word;
dictfile.open("words.txt");
if (!dictfile) {
cout <<"file not found!" << endl;
return (1);
}
else
cout << "file was open\n";
nwords = loadWords(dictfile, dict);
cout << "Please enter a string for an anagram: ";
cin >> word;
int numMatches = recBlends(word, dict, nwords, results);
if (!numMatches)
cout << "No matches found" << endl;
else
showResults(results, numMatches);
return 0;
}
int loadWords(istream& dictfile, string dict[]) {
string data;
if (dictfile.eof()) { // end of dictfile
return 0;
}
dictfile >> data;
// uses recursion to loop to the end of dictfile (dict starts with Z)
int x = loadWords(dictfile, dict);
if (x < MAXDICTWORDS) {
dict[x] = data;
x++;
return x;
}
else
return MAXDICTWORDS; //reached max capacity of array
}
void showResults(const string results[], int size) {
if (size - 1 < 0)
return;
cout << "Matching word " << results[size - 1] << endl;
showResults(results, size - 1);
}
int recBlends(string word,
const string dict[], int size, string results[])
{
int back = word.size() - 1;
int matched = 0;
//permute(word, 0, back, dict, size, matched, results);
return matched;
}
void printPermutations(string prefix, string rest) {
//if rest is empty, printing prefix
if (rest.length() == 0) {
cout << prefix << endl;
}
else {
//otherwise passing prefix and rest to loop, loop from
//0 to rest.length-1
loop(prefix, rest, 0, rest.length());
}
}
//loop method implementation, to loop for values between i and max-1
void loop(string prefix, string rest, int i, int max) {
//if i exceeds or equals max, returning
if (i >= max) {
return;
}
//else, appending char at index i to prefix
string updated_prefix = prefix + rest[i];
//taking out char at index i from rest to create a string without current char
//(this is done by concatenating string before index i with string after index i)
string updated_rest = rest.substr(0, i) + rest.substr(i + 1);
//calling printPermutations, passing these updated values
printPermutations(updated_prefix, updated_rest);
//moving to the next iteration of the loop, passing i+1 as new value for i
loop(prefix, rest, i + 1, max);
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

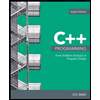
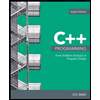