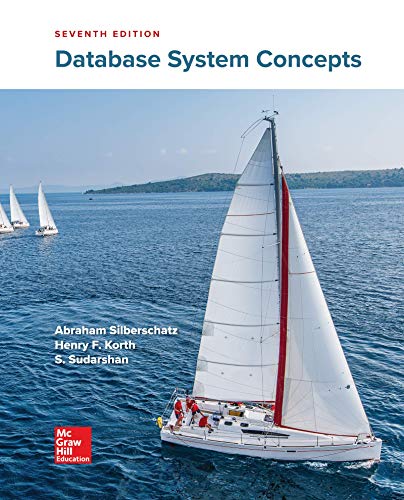
Concept explainers
Create a new project in IntelliJ called Recursion. Add a new Java class called Recursion with a public static main function. You will add several functions to your Recursion class as follows:
- 1 Write a recursive function int sum(int n) that adds up the numbers from n to 1 and print your result. For example from main do the following:
Recursion recursion = new Recursion();
System.out.println(recursion.sum(6));
1.2 Write a recursive function factorial(int n) that calculates the factorial of n. Print out the results. Add your function to your Recursion class.
1.3. Write a recursive function powerOf10(int n) that calculates 10n.
1.4. Write a more general recursive function powerOfN(int x, int p) that calculates the power of xp. Do not use the Math.pow function.
1.5. Write a function int bunnyEars(int n) that calculates the number of bunny ears for n bunnies. Bunnies have two ears.

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- Write a java method that receives a reference to an array of integers and then sorts only the non-perfect numbers. if a number is perfect then it must remain in its current location in the array. Notes: 1) You are not allowed to use the Arrays.sort() method or any other ready-made sorting method. 2) You can not define new arrays. Your sorting must be in-place. 3) A number if perfect if the some of its divisors is equal to the number itself. For example the number 6 is perfect because the sum of its divisors is 1+2+3 = 6 while 8 is not perfect because the sum of its divisors is 1+2+4 = 7 which is not equal to 8.arrow_forwardIn Java Modify Searching class: – Count how many comparisons are performed during each search – Print the number for each search along the results of the search Searching class.java: //********************************************************************// Searching.java Author: Lewis/Loftus//// Demonstrates the linear search and binary search algorithms.//******************************************************************** public class Searching<T>{ //----------------------------------------------------------------- // Searches the specified array of objects for the target using // a linear search. Returns a reference to the target object from // the array if found, and null otherwise. //----------------------------------------------------------------- public T linearSearch(T[] list, T target) { int index = 0; boolean found = false; while (!found && index < list.length) { if…arrow_forwardWrite code in Java: -Must be recursive import java.util.*; import java.lang.*; import java.io.*; //*Analysis goes here* //*Design goes here* class AllPermutation { public static void displayPermutation(String s) { //*Code goes here* } public static void displayPermutation(String s1, String s2) { //*Code goes here* } } //*Driver class should not be changed* class DriverMain { public static void main(String args[]) { Scanner input = new Scanner(System.in); AllPermutation.displayPermutation(input.nextLine()); } }arrow_forward
- A binary operator is an operation that is performed on two operands. For example, addition is a binary operator that adds two operands (e.g., 2 + 4). In the provided UML diagram, all binary operators have two operands and an execute method that performs the appropriate operation on the operands. The AddOperation class should add the two operands. The SubtractOperation class should subtract the two operands. Here is an example of how a binary operator could be used: IBinaryOperator operator = new AddOperator(8, 12);System.out.println(operator.execute()); // should print 20operator.setLeftOperand(18);System.out.println(operation.execute()); // should print 30 crate a Main.java to test your codearrow_forwardsolve the followingarrow_forwardUse java and correctly indent code.arrow_forward
- Using the code in the image provided: Implement a method called summation which adds two integers and returns their sum. INPUT: The first line of input contains an integer a. The Second Line of input containes and integer b. OUTPUT: Print the result which is the sum of a and b.arrow_forwardWrite a static recursive method in Java called mRecursion that displays all of the permutations of the charactersin a string passed to the method as its argument. For example, the character sequence abc has thefollowing permutations: acb, bac, bca, cab, cba. Then Write a static method called getInput that get aninput string from the user and passed it to the mRecursion method written above in a method call.Please does so using what I already had //Get input from in to call recursive method //to display those char permutations public static String getInput ( ) { Scanner in = new Scanner(System.in); String combination = in.nextLine(); System.out.println("Enter string:); return stringComb; //Method to show permutations of a desired string// This only return 3 string combination for some reason static void myRecursion(String aString) { //isEmpty check if ( aString.length() == 0){…arrow_forwardPlease help me with the below using java. Please also comment the code (explai. What each li e is doing). Please make sure both codes are completed using recursionarrow_forward
- Design and implement a program that implements Euclid’s algorithm for finding the greatest common divisor of two positive integers. The greatest common divisor is the largest integer that divides both values without producing a remainder. In a class called DivisorCalc, define a static method called gcd that accepts two integers, num1 and num2. Create a driver to test your implementation. The recursive algorithm is defined as follows:gcd (num1, num2) is num2 if num2 <= num1 and num2evenly divides num1gcd (num1, num2) is gcd(num2, num1) if num1 < num2gcd (num1, num2) is gcd(num2, num1%num2) otherwisearrow_forward19- java. Which of the following is a drawback of using recursion? It may be less efficient than an iterative version. It may have fewer lines of code than an iterative version. It uses lesser memory stack than an iterative version. It runs faster than an iterative version.arrow_forwardPlease answer in C++ and give explanation LAB 17.1-B Linked List Read the internal documentation for class ItemList carefully. Fill in the missing code in the implementation file, being careful to adhere to the preconditions and postconditions. Compile your program. ------------------------------------------------------------------------------------------------------------------------------- class ItemList { private: struct ListNode { int value; ListNode * next; }; ListNode * head; public: ItemList(); // Post: List is the empty list. bool IsThere(int item) const; // Post: If item is in the list IsThere is // True; False, otherwise. void Insert(int item); // Pre: item is not already in the list. // Post: item is in the list. void Delete(int item); // Pre: item is in the list. // Post: item is no…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
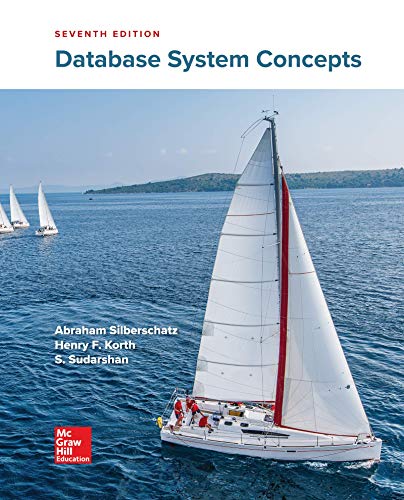
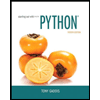
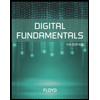
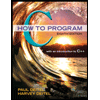
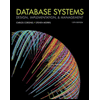
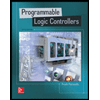