Hi, I am not sure what's wrong with my code. Can you please check why it is giving me an error? In the starter file is a partial implementation of a doubly-linked list in DoublyLinkedList.java. We will write three new methods in this class to provide additional functionality. Write a method addFirst that adds a new element at the beginning of a DoublyLinkedList. Write a method addLast that adds a new element at the end of a DoublyLinkedList. Write a method removeFirst that removes and returns the first element of a DoublyLinkedList. Try to keep your implementations as simple as possible. For example, recall this definition of addFirst in the (Singly) LinkedList class: public void addFirst(E value) { head = new Node(value, head); } In the DoublyLinkedList class, you will need to keep the three instance variables head, tail, and count updated in all methods. Note that addFirst and addLast will be symmetric to each other, as will removeFirst and removeLast (provided in the starter code).
Hi, I am not sure what's wrong with my code. Can you please check why it is giving me an error?
In the starter file is a partial implementation of a doubly-linked list in DoublyLinkedList.java. We will write three new methods in this class to provide additional functionality.
-
Write a method addFirst that adds a new element at the beginning of a DoublyLinkedList.
-
Write a method addLast that adds a new element at the end of a DoublyLinkedList.
-
Write a method removeFirst that removes and returns the first element of a DoublyLinkedList.
Try to keep your implementations as simple as possible. For example, recall this definition of addFirst in the (Singly) LinkedList class:
public void addFirst(E value) { head = new Node(value, head); }
In the DoublyLinkedList class, you will need to keep the three instance variables head, tail, and count updated in all methods. Note that addFirst and addLast will be symmetric to each other, as will removeFirst and removeLast (provided in the starter code).
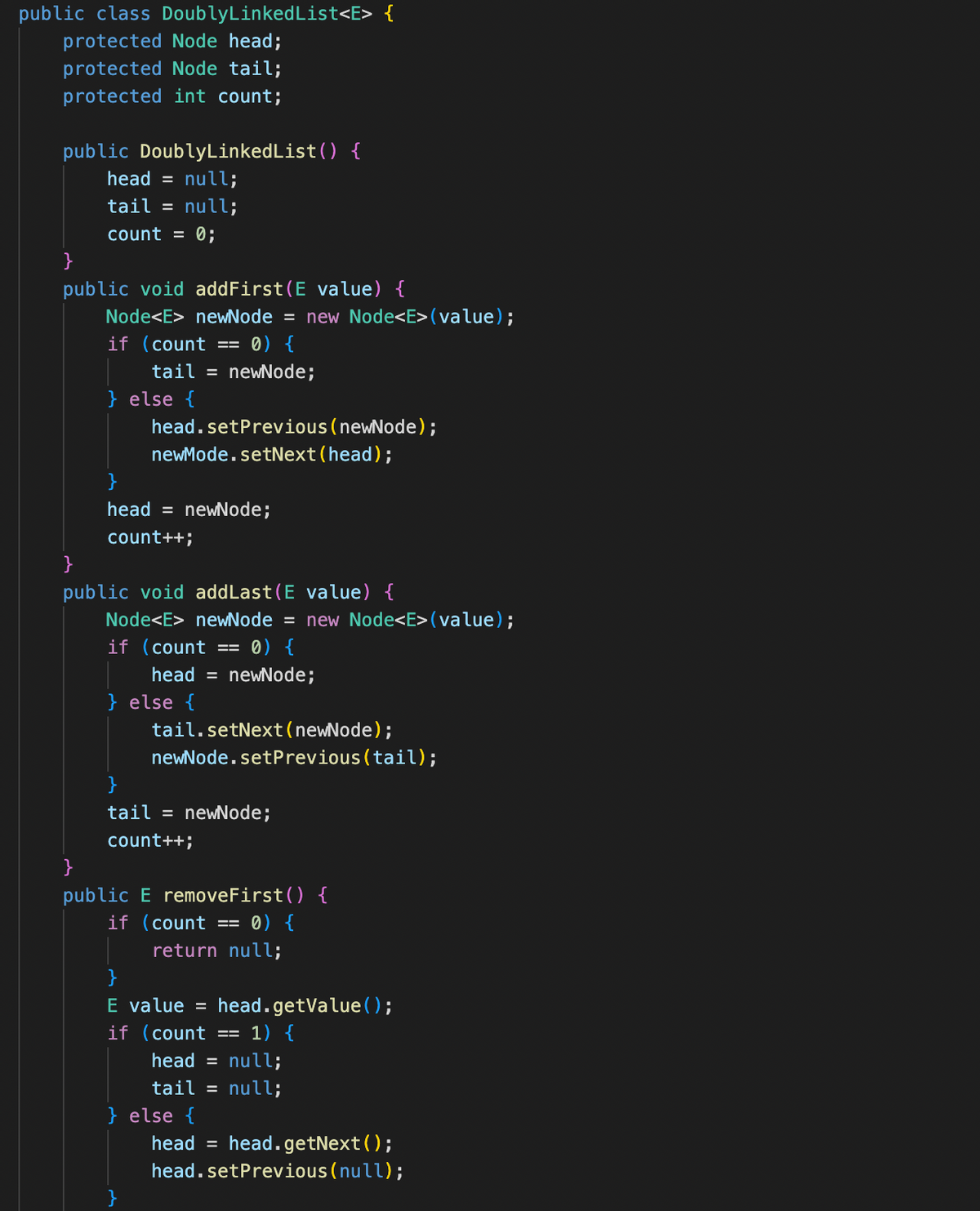
![}
public E removeLast() {
if (tail == null) {
return null;
count--;
return value ();
}
}
else {
E returnValue = tail.value();
tail = tail.prev();
if (tail == null) {
head = null;
}
else {
}
}
tail.setNext (next:null);
public String toString() {
Node finger = head;
String result = "[ ";
while (finger != null) {
count--;
return returnValue;
result + finger.toString() +
finger = finger.next();
}
result += "]";
return result;
/**
* Returns the number of elements in this list.
*
* @return the number of elements in this list
*/
public int size() {
return count;
Run | Debug
public static void main(String[] args) {](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F385eb8fb-6648-4df6-8047-4f830c46ad3b%2Faa46f49f-369f-4595-aec3-91a4f294b95d%2F32z1txf_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 7 steps with 7 images

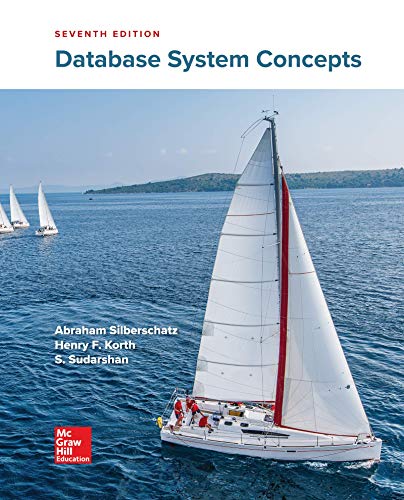
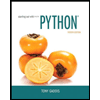
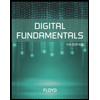
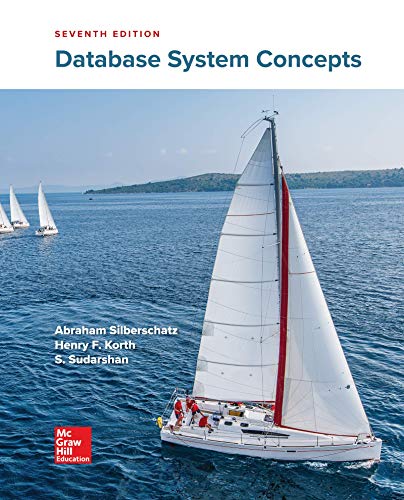
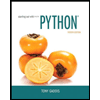
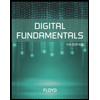
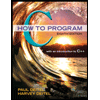
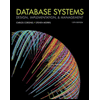
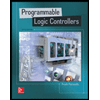