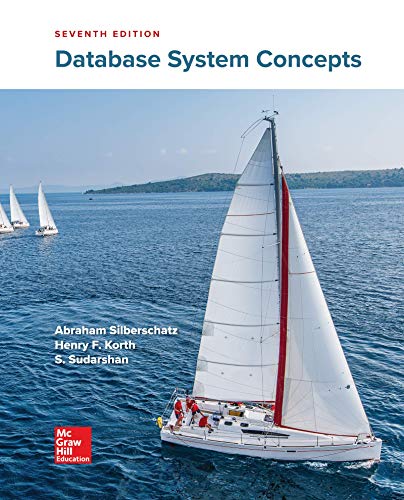
Getting more errors please free.
import java.util.HashMap;
import java.util.Set;
public class MapUse{
//main driver code
public static void main(String[] args) {
HashMap<String, Integer> map = new HashMap<>();
//insert
map.put("abc", 1);
map.put("def", 2);
map.put("abc1", 10);
map.put("def1", 20);
map.put("abc", 5); //updates abc from 1 to 5, every key is unique, no dupplicate keys
//check size
System.out.println("size "+ map.size());
//check Presence
if(map.containsKey("abc")){
System.out.println("has abc");
}
if(map.containsKey("abc1")){
System.out.println("has abc1");
}
//get Value
int v = 0;
if(map.containsKey("abc1")){ //checks whether the key is actually present
v = map.get("abc1"); //to protect from null pointer exception
}
System.out.println(v);
//remove
int s = map.remove("abc"); //removes the value and also returns the value
System.out.println(s);
//iterate
Set<String> keys = map.keySet();
//gives a set of all keys as a Set
for(String str: keys){
System.out.println(str);
}
}.

Step by stepSolved in 3 steps with 1 images

- Complete public class Solution { public static LinkedListNode<Integer> findPrevNode(LinkedListNode<Integer> head, int count) { for (int i=0;i<count-1;i++) { head=head.next; } return head; } public static LinkedListNode<Integer> swapNodes(LinkedListNode<Integer> head, int i, int j) { //Your code goes here if (head==null) { return head; } else if (j==0 || (i-j==-1)) { int temp=i; i=j; j=temp; } LinkedListNode<Integer> swap1=null,swap2=null,p1=null,n1=null,p2=null,n2=null; if (i==0 && i-j==1) { swap1=head; swap2=head.next; swap1=swap1.next; head=swap2; swap2.next=swap1; } else if(i-j==1) { p1=findPrevNode(head,j); swap1=p1.next; swap2=swap1.next; n2=swap2.next; //System.out.println(p1.data); //System.out.println(swap1.data);…arrow_forwardHow do you do this? Javaarrow_forwardJAVA please Given main() in the ShoppingList class, define an insertAtEnd() method in the ItemNode class that adds an element to the end of a linked list. DO NOT print the dummy head node. Ex. if the input is: 4 Kale Lettuce Carrots Peanuts where 4 is the number of items to be inserted; Kale, Lettuce, Carrots, Peanuts are the names of the items to be added at the end of the list. The output is: Kale Lettuce Carrots Peanuts Code provided in the assignment ItemNode.java:arrow_forward
- Consider the following class map, class map { public: map(ifstream &fin); void print(int,int,int,int); bool isLegal(int i, int j); void setMap(int i, int j, int n); int getMap(int i, int j) const; int getReverseMapI(int n) const; int getReverseMapJ(int n) const; void mapToGraph(graph &g); bool findPathRecursive(graph &g, stack<int> &moves); bool findPathNonRecursive1(graph &g, stack<int> &moves); bool findPathNonRecursive2(graph &g, queue<int> &moves); bool findShortestPath1(graph &g, stack<int> &bestMoves); bool findShortestPath2(graph &, vector<int> &bestMoves); void map::printPath(stack<int> &s); int numRows(){return rows;}; int numCols(){return cols;}; private: int rows; // number of latitudes/rows in the map int cols; // number of longitudes/columns in the map matrix<bool> value; matrix<int> mapping; // Mapping from latitude and longitude co-ordinates (i,j) values to node index…arrow_forward25. Show the output of the following code: public class Test { public static void main(String[] args) { Map map = new LinkedHashMap(); map.put("123", "John Smith"); map.put("111", "George Smith"); map.put("123", "Steve Yao"); map.put("222", "Steve Yao"); System.out.printin("(1) " + map): System.out.printin("(2) "+ new TreeMap(map)): } }arrow_forwardimport java.util.*;import java.io.*; public class HuffmanCode { private Queue<HuffmanNode> queue; private HuffmanNode overallRoot; public HuffmanCode(int[] frequencies) { queue = new PriorityQueue<HuffmanNode>(); for (int i = 0; i < frequencies.length; i++) { if (frequencies[i] > 0) { HuffmanNode node = new HuffmanNode(frequencies[i]); node.ascii = i; queue.add(node); } } overallRoot = buildTree(); } public HuffmanCode(Scanner input) { overallRoot = new HuffmanNode(-1); while (input.hasNext()) { int asciiValue = Integer.parseInt(input.nextLine()); String code = input.nextLine(); overallRoot =…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
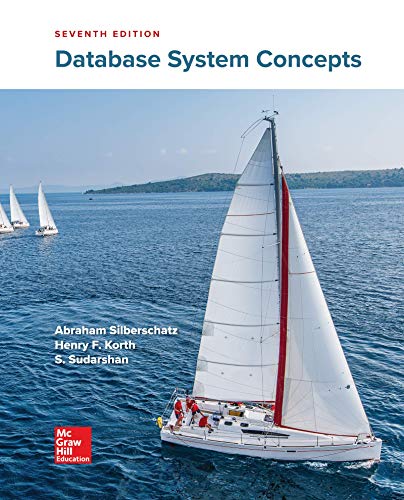
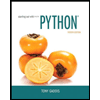
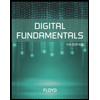
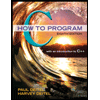
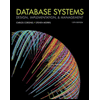
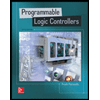