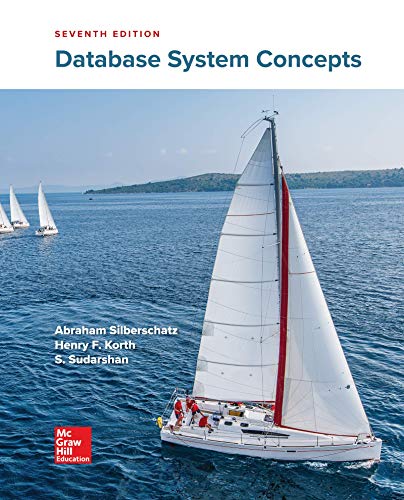
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
public class LinkedList3 {
private Node head = null;
public class Node {
char data;
Node next;
Node(char c) {
data = c;
}
}
build these 3 methods in java language
public int countOccurencesOf(char c) {
int count = 0;
return count;
}
public char getLast() {
}
public void deleteLast() {
}
Expert Solution

arrow_forward
Step 1
The functions have been programmed by me in the below steps.
Step by stepSolved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- add public method size() to the class Stack so the output will be pop is: 4 peek is: 8 size is: 2 false public class Stack<T> { private LinkedList<T> list; public Stack() { list = new LinkedList<>(); } public boolean isEmpty() { return list.isEmpty(); } public void push(T data) { list.prepend(data); } public T pop() { if (isEmpty()) { throw new EmptyStackException(); } return list.removeHead(); } public T peek() { if (isEmpty()) { throw new EmptyStackException(); } return list.getHead(); } } public class Main { public static void main(String[] args) { Stack<Integer> stack = new Stack<>(); stack.push(6); stack.push(8); stack.push(4); System.out.println("pop is: " + stack.pop()); System.out.println("peek is: " + stack.peek()); System.out.println("size is: " +…arrow_forwardimport java.util.HashSet; import java.util.Set; // Define a class named LinearSearchSet public class LinearSearchSet { // Define a method named linearSearch that takes in a Set and an integer target // as parameters public static boolean linearSearch(Set<Integer> set, int target) { // Iterate over all elements in the Set for () { // Check if the current value is equal to the target if () { // If so, return true } } // If the target was not found, return false } // Define the main method public static void main(String[] args) { // Create a HashSet of integers and populate integer values Set<Integer> numbers = new HashSet<>(); // Define the target to search for numbers.add(3); numbers.add(6); numbers.add(2); numbers.add(9); numbers.add(11); // Call the linearSearch method with the set…arrow_forwardimport java.util.*;public class MyLinkedListQueue{ class Node { public Object data; public Node next; private Node first; public MyLinkedListQueue() { first = null; } public Object peek() { if(first==null) { throw new NoSuchElementException(); } return first.data; } public void enqueue(Object element) { Node newNode = new newNode(); newNode.data = element; newNode.next = first; first = newNode; } public boolean isEmpty() { return(first==null); } public int size() { int count = 0; Node p = first; while(p ==! null) { count++; p = p.next; } return count; } } } Hello, I am getting an error message when I execute this program. This is what I get: MyLinkedListQueue.java:11: error: invalid method declaration; return type required public MyLinkedListQueue() ^1 error I also need to have a dequeue method in my program. It is very similar to the enqueue method. I'll…arrow_forward
- Complete public class Solution { public static LinkedListNode<Integer> findPrevNode(LinkedListNode<Integer> head, int count) { for (int i=0;i<count-1;i++) { head=head.next; } return head; } public static LinkedListNode<Integer> swapNodes(LinkedListNode<Integer> head, int i, int j) { //Your code goes here if (head==null) { return head; } else if (j==0 || (i-j==-1)) { int temp=i; i=j; j=temp; } LinkedListNode<Integer> swap1=null,swap2=null,p1=null,n1=null,p2=null,n2=null; if (i==0 && i-j==1) { swap1=head; swap2=head.next; swap1=swap1.next; head=swap2; swap2.next=swap1; } else if(i-j==1) { p1=findPrevNode(head,j); swap1=p1.next; swap2=swap1.next; n2=swap2.next; //System.out.println(p1.data); //System.out.println(swap1.data);…arrow_forwardclass BSTNode { int key; BSTNode left, right; public BSTNode(int item) { key = item; left = right = null; } } class BST_Tree { BSTNode root; BST_Tree() { // Constructor root = null; } boolean search(int key){ return (searchRec(root, key) != null); } public BSTNode searchRec(BSTNode root, int key) { if (root==null || root.key==key) return root; if (root.key > key) return searchRec(root.left, key); return searchRec (root.right, key); } void deleteKey(int key) { root = deleteRec(root, key); } /* A recursive function to insert a new key in BST */ BSTNode deleteRec(BSTNode root, int key) { /* Base Case: If the tree is empty */ if (root == null) return root; /* Otherwise, recur down the tree */ if (key < root.key)…arrow_forwardI need an explanation for this program. class Directory { Node head,tail; Directory() { head=null; tail=null; } public void insert(Node node) // method that insers node into list if phone number is new { Node temp=head; while(temp!=null && !temp.phone_number.equals(node.phone_number)) temp=temp.next; if(temp!=null) { System.out.println("Entered phone number already exists"); return; } if(head==null) { head=node; tail=node; } else { tail.next=node; tail=node; } System.out.println("Inserted Successfully"); } public void remove(String ph_no) // method that removes node with given phone number { Node prev=null; Node node=head; while(node!=null && !node.phone_number.equals(ph_no)) { prev=node;…arrow_forward
- import java.util.*; public class Main{ public static void main(String[] args) { Main m = new Main(); m.go(); } private void go() { List<Stadium> parks = new ArrayList<Stadium>(); parks.add(new Stadium("PNC Park", "Pittsburgh", 38362, true)); parks.add(new Stadium("Dodgers Stadium", "Los Angeles", 56000, true)); parks.add(new Stadium("Citizens Bank Park", "Philadelphia", 43035, false)); parks.add(new Stadium("Coors Field", "Denver", 50398, true)); parks.add(new Stadium("Yankee Stadium", "New York", 54251, false)); parks.add(new Stadium("AT&T Park", "San Francisco", 41915, true)); parks.add(new Stadium("Citi Field", "New York", 41922, false)); parks.add(new Stadium("Angels Stadium", "Los Angeles", 45050, true)); Collections.sort(parks, Stadium.ByKidZoneCityName.getInstance()); for (Stadium s : parks) System.out.println(s); }}…arrow_forwardi need it ASAP pleasearrow_forwardwrite this code below as algorithim to determine the leaf node reclusively ? public static void printLeafNodes(TreeNode node) { // base case if (node == null) { return; } if (node.left == null && node.right == null) { System.out.printf("%d ", node.value); } printLeafNodes(node.left); printLeafNodes(node.right); }arrow_forward
- class BSTNode { int key; BSTNode left, right; public BSTNode(int item) { key = item; left = right = null; } } class BST_Tree { BSTNode root; BST_Tree() { // Constructor root = null; } boolean search(int key){ return (searchRec(root, key) != null); } public BSTNode searchRec(BSTNode root, int key) { if (root==null || root.key==key) return root; if (root.key > key) return searchRec(root.left, key); return searchRec (root.right, key); } void deleteKey(int key) { root = deleteRec(root, key); } /* A recursive function to insert a new key in BST */ BSTNode deleteRec(BSTNode root, int key) { /* Base Case: If the tree is empty */ if (root == null) return root; /* Otherwise, recur down the tree */ if (key < root.key)…arrow_forwardRedesign LaptopList class from previous project public class LaptopList { private class LaptopNode //inner class { public String brand; public double price; public LaptopNode next; public LaptopNode(String brand, double price) { // add your code } public String toString() { // add your code } } private LaptopNode head; // head of the linked list public LaptopList(String fname) throws IOException { File file = new File(fname); Scanner scan = new Scanner(file); head = null; while(scan.hasNextLine()) { // scan data // create LaptopNode // call addToHead and addToTail alternatively } } private void addToHead(LaptopNode node) { // add your code } private void addToTail(LaptopNode node) { // add your code } private…arrow_forwardPLEASE HELP, PYTHON THANK YOU def add_vertex(self, vertex): if vertex not in self.__graph_dict: self.__graph_dict[vertex] = [] def add_edge(self, edge): edge = set(edge) (vertex1, vertex2) = tuple(edge) ################# # Problem 4: # Check to see if vertex1 is in the current graph dictionary ################## #DELETE AND PUT IN THE IF STATEMENTS self.__graph_dict[vertex1].append(vertex2) else: self.__graph_dict[vertex1] = [vertex2] def __generate_edges(self): edges = [] ################# # Problem 5: # Loop through all of the data in the graph dictionary and use the variable vertex for the loop'ed data ################## #DELETE AND PUT IN THE LOOP STATEMENTS for neighbour in self.__graph_dict[vertex]: if {neighbour, vertex} not in edges: edges.append({vertex, neighbour}) return edges…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
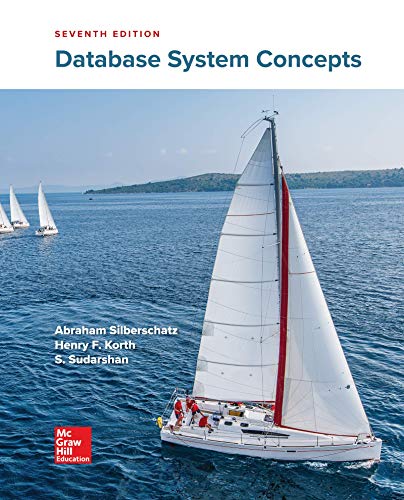
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
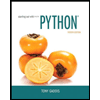
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
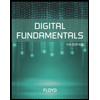
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
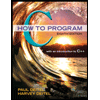
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
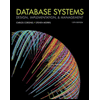
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
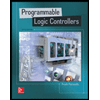
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education