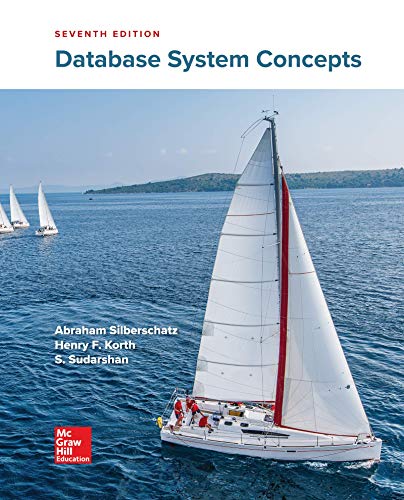
I have a program that asks for queries. It is based on a CSV file with three columns for a person's name, city, and height. The print statement for City_list gives it a blank. The problem I'm running into is that if I insert a city that isn't in the CSV file, it ends and doesn't transition to other cities. When I do put in the city that is in the list, it prints out the indexes from the file. How can this be revised to ignore off-list cities and work with in-list cities?
Example Output:
C:\Users\lucas\PycharmProjects\pythonProject3\venv\Scripts\python.exe C:\Users\lucas\Documents\cs1151\ch8\querydata.py
Welcome to my query program!
What would you like to do?
1) Count how many people in a city:
2) Find the tallest person/people in a city.
0) Quit
Option: 1
Which city?Ely
The population of Ely is 10
What would you like to do?
1) Count how many people in a city:
2) Find the tallest person/people in a city.
0) Quit
Option: 2
Which city?Ely
[1, 12, 13, 14, 20, 25, 40, 65, 84, 90]
# Lucas Conklin
# 5772707
import csv
def readCSVIntoDictionary(f_name):
dictionary = dict()
with open(f_name) as f:
f_reader = csv.reader(f)
headers = next(f_reader)
id = 1
for row in f_reader:
item_dictionary = dict()
for i in range(0, len(row)):
item_dictionary[headers[i]] = row[i]
dictionary[id] = item_dictionary
id += 1
f.close()
return dictionary
def printItem(item):
for k, v in item.items():
print(f' {k:20}: {v}')
def lookupIDs(mydict, key, value):
matching_keys = []
for i in mydict.keys():
if mydict[i][key] == value:
matching_keys.append(i)
return matching_keys
def people_counter(dict, city):
city_pop = lookupIDs(dict, "city", city)
return len(city_pop)
def find_tallest(dict, city):
city_dict = lookupIDs(dict, "city", city)
print(city_dict)
tall = 0
for i in city_dict:
height = int(dict[i]["height"])
if height > tall:
tall = height
tallest = []
for i in city_dict:
height = int(dict[i]["height"])
if tall == height:
name = dict[i]["name"]
tallest.append(name)
while True:
if city_dict == []:
continue
return tallest
cityDictionary = readCSVIntoDictionary("C:\\Users\\lucas\\Downloads\\sample-data.csv")
print("Welcome to my query program!")
while True:
print("What would you like to do?")
print()
print("1) Count how many people in a city: ")
print("2) Find the tallest person/people in a city.")
print("0) Quit")
option = int(input("Option: "))
if option == 0:
print("Goodbye!")
break
elif option == 1:
city = input("Which city?")
pop = people_counter(cityDictionary, city)
if pop == 0:
print(f'There are no people in {city}.')
else:
print(f'The population of {city} is {pop}')
elif option == 2:
city = input("Which city?")
people_list = find_tallest(cityDictionary, city)
if len(people_list) <= 1:
print(f'The tallest people/person in {city} is {people_list[0]}')
else:
print(f'The tallest people/person in {city} are ')
for people in people_list:
print(f' {people} ')

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- We want the program to use a DEQueue object to create a sorted list of names. The program gives a user the following three options to choose from – insert, delete, and quit. If the user selects ‘insert’, the program shouldaccept a name (String type) from the user and insert it into the deque in a sorted manner. If theuser selects ‘delete’, the program should accept a name (String type) from the user and deletethat name from the deque. Display the contents of deque after each insert/delete operation. Theprogram continues to prompt the user for options until the user chooses to quit. You have toimplement the insert and delete operationsarrow_forwardI have to create a program using python so when the user inputs a state, it gives them the info of the capital, state bird, and state rank. Here are the instructions: Your program will initialize lists to store information about the states. You should have one list for the names of the states, one list for the names of the capitals, one list for the names of the state birds per state, and one list for the order number the state joined the union. Alternatively, you can use a db list of lists as demonstrated in the exercises in the book to store the information in one structure or dictionaries. Next ask the user to enter a name of a state. Find the index of that name. Use that index to report back to the user the name of the capital, state bird, and the order the state joined the union.arrow_forwardThis is the question I am stuck on - Write an application that accepts up to 20 Strings, or fewer if the user enters the terminating value ZZZ. Store each String in one of two lists—one list for short Strings that are 10 characters or fewer and another list for long Strings that are 11 characters or more. After data entry is complete, prompt the user to enter which type of String to display, and then output the correct list. For this exercise, you can assume that if the user does not request the list of short strings, the user wants the list of long strings. If a requested list has no Strings, output The list is empty. Prompt the user continuously until a sentinel value, ZZZ, is entered. This is the code I have tried to start with but I am honestly just confusing myself more- import java.util.*; public class CategorizeStrings { public static void main (String[] args) { // your code here int count = 20; int x; short_string = [] long_string = []…arrow_forward
- Let's finish working on our booking system. The last part for our booking system will be to manage multiple flights. To do this, we'll modify our program's command list. The command "create [id] [cap]" will try to create a new empty flight with ID [id] and capacity [cap]. The command "delete [id]" will try to remove the flight with ID [id]. The command "add [id] [n]" will try to add n reservations to the flight with ID [id]. The command "cancel [id] [n]" will try to cancel n reservations from the flight with ID [id]. If an operation fails for any reason, the program will issue the message "Cannot perform this operation". You can add a more helpful message to identify why the operation failed. The command "quit" will stop the execution of the program. For the sake of simplicty, let's limit the maximum number of handled flights to ten. Use the code from your previous exercise as a starting point. Note that we need to add an access method to the flight id field. PLS ANSWER…arrow_forwardIn Java: When analyzing data sets, such as data for human heights or for human weights, a common step is to adjust the data. This can be done by normalizing to values between 0 and 1, or throwing away outliers. For this program, adjust the values by subtracting the smallest value from all the values. The input begins with an integer indicating the number of integers that follow. Assume that the list will always contain less than 20 integers. Ex: If the input is: 5 30 50 10 70 65 the output is: 20 40 0 60 55 For coding simplicity, follow every output value by a space, even the last one. Your program must define and call a method:public static int getMinimumInt(int[] listInts, int listSize) import java.util.Scanner; public class LabProgram {/* Define your method here */ public static void main(String[] args) {/* Type your code here. */}}arrow_forwardThe for construct is a kind of loop that iteratively processes a sequence of items. So long as there are things to process, it will continue to operate. In your opinion, how true is this statement?arrow_forward
- I need to write a Java ArrayList program called Search.java that counts the names of people that were interviewed from a standard input stream. The program reads people's names from the standard input stream and from time to time prints a sorted portion of the list of names. All the names in the input are given in the order in which they were found and interviewed. You can imagine a unique timestamp associated with each interview. The names are not necessarily unique (many of the people have the same name). Interspersed in the standard input stream are queries that begin with the question mark character. Queries ask for an alphabetical list of names to be printed to the standard output stream. The list depends only on the names up to that point in the input stream and does include any people's names that appear in the input stream after the query. The list does not contain all the names of the previous interviews, but only a selection of them. A query provides the names of two people,…arrow_forwardWrite a program in C++ that prints a sorted phone list from a database of names and phone numbers. The data is contained in two files named "phoneNames.txt" and "phoneNums.txt". The files may contain up to 2000 names and phone numbers. The files have one name or one phone number per line. To save paper, only print the first 50 lines of the output. Note: The first phone number in the phone number file corresponds to the first name in the name file. The second phone number in the phone number file corresponds to the second name in the name file. etc. You will find the test files in the Asn Five.ziparrow_forwardWritten in python with docstrings if applicable. Thanksarrow_forward
- Write a python program that creates a dictionary containing the U.S. states as keys, and their capitals as values. The program should then randomly quiz the user by displaying the name of a state and asking the user to enter that state's capital. The program should keep a count of the number of correct and incorrect responses. This program has really been giving me trouble. Any help is greatly appreciated. Thanks so much!arrow_forwardYou are going to write a program (In Python) called BankApp to simulate a banking application.The information needed for this project are stored in a text file. Those are:usernames, passwords, and balances.Your program should read username, passwords, and balances for each customer, andstore them into three lists.userName (string), passWord(string), balances(float)The txt file with information is provided as UserInformtion.txtExample: This will demonstrate if file only contains information of 3 customers. Youcould add more users into the file.userName passWord Balance========================Mike sorat1237# 350Jane para432@4 400Steve asora8731% 500 When a user runs your program, it should ask for the username and passwordfirst. Check if the user matches a customer in the bank with the informationprovided. Remember username and password should be case sensitive.After asking for the user name, and password display a menu with the…arrow_forwardPYTHON Complete the function below, which takes two arguments: data: a list of tweets search_words: a list of search phrases The function should, for each tweet in data, check whether that tweet uses any of the words in the list search_words. If it does, we keep the tweet. If it does not, we ignore the tweet. data = ['ZOOM earnings for Q1 are up 5%', 'Subscriptions at ZOOM have risen to all-time highs, boosting sales', "Got a new Mazda, ZOOM ZOOM Y'ALL!", 'I hate getting up at 8am FOR A STUPID ZOOM MEETING', 'ZOOM execs hint at a decline in earnings following a capital expansion program'] Hint: Consider the example_function below. It takes a list of numbers in numbers and keeps only those that appear in search_numbers. def example_function(numbers, search_numbers): keep = [] for number in numbers: if number in search_numbers(): keep.append(number) return keep def search_words(data, search_words):arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
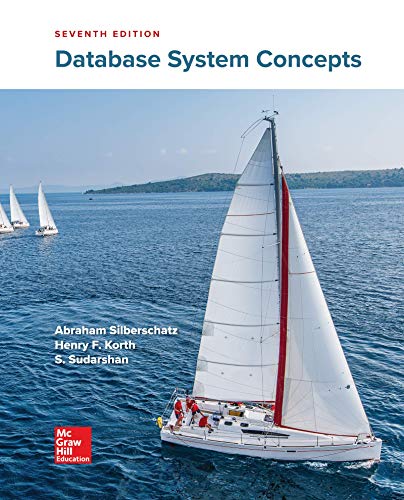
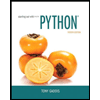
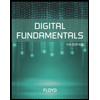
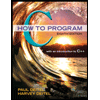
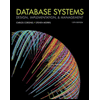
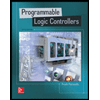