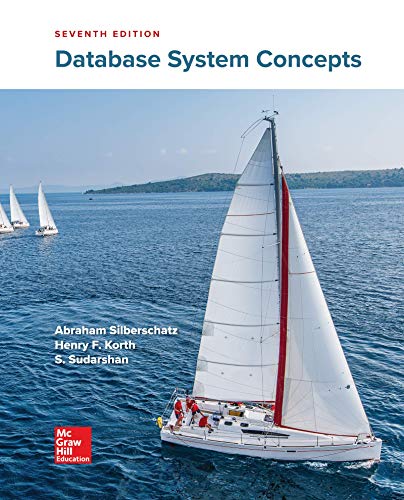
Most of it is done except that little area i put "NEED HELP IN". Thank you!
Please complete the following function getMax, which accepts three integer parameters (int p, int q, int r), and must return the maximum of those 3 integers received to the caller.
int getMax(int p, int q, int r) // returns the max of 3 integers
{ // begin getMax()
:::::: NEED HELP HERE ::::::
} // end getMax()
void main( ) // To test function getMax( )
{ cout << "Data: 9 18 4 , Max = " << getMax(9, 18, 4) << endl;
cout << "Data: 99 18 4 , Max = " << getMax(99, 18, 4) << endl;
cout << "Data: 9 18 49 , Max = " << getMax(9, 18, 49) << endl;
}
The output of the above program should be as follows:
Data: 9 18 4 , Max = 18
Data: 99 18 4 , Max = 99
Data: 9 18 49 , Max = 49

Step by stepSolved in 2 steps with 1 images

- Please complete the following function getGrade, which accepts only one parameter (int score), and must return its proper grade (i.e., char grade) to the caller. This function must determine the proper grade based on the score received as follows: THIS IS THE CODE I HAVE SO FAR. Need help with areas market "******". Thank you! grade = 'A' if score == 4 ; grade = 'B' if score == 3 ; grade = 'C' if score == 2 ; grade = 'D' if score == 1 ; grade = 'F' if score == 0 ; Otherwise, grade = '?' ; char getGrade( int score) // function returns a char to the caller { // parameter 1: int score (4 for example) // return: char grade (‘A’ for example) char grade = '?' ; // This is the only variable you can use ********* NEED HELP HERE ********* return grade ; } // end char getGrade( ) void main() // To test function getGrade( ) { cout << "score 4 -> grade = "…arrow_forwarddef double(num): num = float(num) return num * 2 double(5.0) I passed 5/6 cases. However, I don't know how to fix the Missing function_call error. Can you please help me?arrow_forwardCan someone help me to explain this? This is a pastime question. The answer is (C), which is 101, but I don't understand why. Question: Assume the following function has been implemented. def descript(num: int)-> str: """Return "High" if num is greater than 100, return "Low" if num is between 0 and 100 inclusive, and return "Neg" otherwise """ Also assume that the implementation has been tested on the inputs: 500, 100, 0, -1, -50. Which of the following inputs would be the least redundant for testing purposes. A. 0 B. 50 C. 101 D. 1000000000 E. They are all equally redundant.arrow_forward
- Python question please include all steps and screenshot of code. Also please provide a docstring, and comments through the code, and test the given examples below. Thanks. Write a function called qsolver() that solves a quadratic equation: ax2+bx+c=0. Yourfunction will take three input arguments, a, b, and c, first compute the discriminant d =b2 - 4*a*c. If d < 0, the equation has no real roots. If d == 0 then the equation has one realroot equal to -b / (2*a). If d > 0, the equation has two roots: (-b - math.sqrt(d)) / (2*a) and(-b+math.sqrt(d))/(2*a). Your program should solve the equation given by coefficients a,b, and c, and then return string "No roots", or value ____ , or tuple (____, ____).>>> qsolver(1,2,1)-1.0>>> qsolver(1,0,-1)(1.0, -1.0)>>> qsolver(1,0,1)'No roots'arrow_forwardHere is the main function in a program int main () { int x = 30; //more code goes here someFunction (x) ; cout « x; return 0; } Assume the heading for someFunction is written correctly with an integer parameter named x, and that this is the body of that function: { x = x * 20; //more code goes here } What will be the output? Just enter a number. If there would be an error, enter -999arrow_forward//Write a function that loops through and console.log's the numbers from 1 to 100, except multiples of three, log (without quotes) "VERY GOOD" instead of the number, for the multiples of five, log (without quotes) "SUPER AWESOME". For numbers which are multiples of both three and five, log (without quotes) "VERY GOOD SUPER AWESOME" Console log out: 12VERY GOOD4SUPER AWESOMEVERY GOOD78VERY GOODSUPER AWESOME11VERY GOOD1314VERY GOOD SUPER AWESOME1617VERY GOOD19SUPER AWESOMEVERY GOOD2223VERY GOODSUPER AWESOME26VERY GOOD2829VERY GOOD SUPER AWESOMEarrow_forward
- Create a function that determines whether each seat can "see" the front-stage. A number can "see" the front-stage if it is strictly greater than the number before it. Everyone can see the front-stage in the example below: # FRONT STAGE [[1, 2, 3, 2, 1, 1], [2, 4, 4, 3, 2, 2], [5, 5, 5, 5, 4, 4], [6, 6, 7, 6, 5, 5]] # Starting from the left, the 6 > 5 > 2 > 1, so all numbe # 6 > 5 > 4 > 2 - so all numbers can see, etc. Not everyone can see the front-stage in the example below: # FRONT STAGE [[1, 2, 3, 2, 1, 1], [2, 4, 4, 3, 2, 2], [5, 5, 5, 10, 4, 4], [6, 6, 7, 6, 5, 5]] # The 10 is directly in front of the 6 and blocking its varrow_forwardPlease tell me where I get wrong.arrow_forwardCan i please get help with this, thanksarrow_forward
- How do you do 7 and 8?arrow_forwardPlease complete the following function getMax, which accepts three integer parameters (int p, int q, int r), and must return the maximum of those 3 integers received to the caller. THIS IS ALL I HAVE SO FAR. AREAS MARKED WITH ":::::::" is where I need help. Thank you! int getMax(int p, int q, int r) // returns the max of 3 integers { // begin getMax() :::::::: NEED HELP HERE :::::::: } // end getMax() void main( ) // To test function getMax( ) { cout << "Data: 9 18 4 , Max = " << getMax(9, 18, 4) << endl; cout << "Data: 99 18 4 , Max = " << getMax(99, 18, 4) << endl; cout << "Data: 9 18 49 , Max = " << getMax(9, 18, 49) << endl; } The output of the above program should be as follows: Data: 9 18 4 , Max = 18 Data: 99 18 4 , Max = 99 Data: 9 18 49 , Max = 49arrow_forwardPython question please include all steps and screenshot of code. Also please provide a docstring, and comments throughout the code, and test the given examples below. Thanks. Develop function fingerprint() that takes text (as a string) as input and creates andreturns the text “fingerprint” obtained as follows: replace each word in the text by itslength (i.e., the number of letters) and concatenate these numbers.>>> fingerprint('This is a secret message')'42167'>>> fingerprint('This message has a different fingerprint')'4731911'>>> fingerprint('Very Short')'45'arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
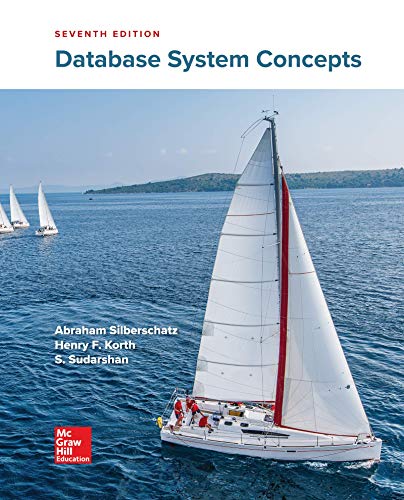
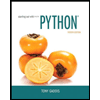
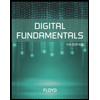
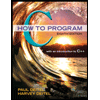
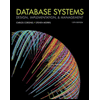
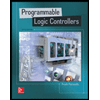