Please complete the following function getMax, which accepts three integer parameters (int p, int q, int r), and must return the maximum of those 3 integers received to the caller. THIS IS ALL I HAVE SO FAR. AREAS MARKED WITH ":::::::" is where I need help. Thank you! int getMax(int p, int q, int r) // returns the max of 3 integers { // begin getMax() :::::::: NEED HELP HERE :::::::: } // end getMax() void main( ) // To test function getMax( ) { cout << "Data: 9 18 4 , Max = " << getMax(9, 18, 4) << endl; cout << "Data: 99 18 4 , Max = " << getMax(99, 18, 4) << endl; cout << "Data: 9 18 49 , Max = " << getMax(9, 18, 49) << endl; } The output of the above program should be as follows: Data: 9 18 4 , Max = 18 Data: 99 18 4 , Max = 99 Data: 9 18 49 , Max = 49
Please complete the following function getMax, which accepts three integer parameters (int p, int q, int r), and must return the maximum of those 3 integers received to the caller.
THIS IS ALL I HAVE SO FAR. AREAS MARKED WITH ":::::::" is where I need help. Thank you!
int getMax(int p, int q, int r) // returns the max of 3 integers
{ // begin getMax()
:::::::: NEED HELP HERE ::::::::
} // end getMax()
void main( ) // To test function getMax( )
{ cout << "Data: 9 18 4 , Max = " << getMax(9, 18, 4) << endl;
cout << "Data: 99 18 4 , Max = " << getMax(99, 18, 4) << endl;
cout << "Data: 9 18 49 , Max = " << getMax(9, 18, 49) << endl;
}
The output of the above program should be as follows:
Data: 9 18 4 , Max = 18
Data: 99 18 4 , Max = 99
Data: 9 18 49 , Max = 49

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

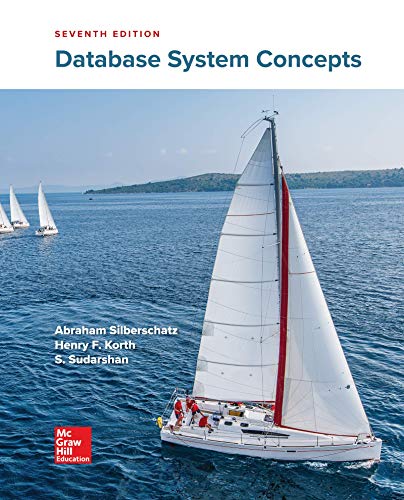
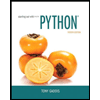
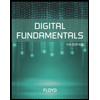
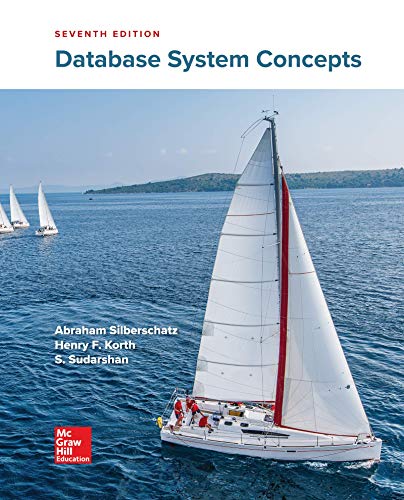
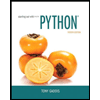
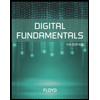
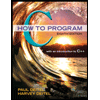
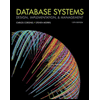
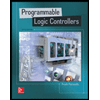