How do I fix the Java code errors? Code: //import java.util.Arrays; //Movie.java package movie; public class Movie { private String movieName; private int numMinutes; private boolean isKidFriendly; private int numCastMembers; private String[] castMembers; public Movie() { movieName = "Flick"; numMinutes = 0; isKidFriendly = false; numCastMembers = 0; castMembers = new String[10]; } public Movie(String movieName, int numMinutes, boolean isKidFriendly, String[] castMembers) { this.movieName = movieName; this.numMinutes = numMinutes; this.isKidFriendly = isKidFriendly; this.numCastMembers = castMembers.length; this.castMembers = castMembers; } public String getMovieName() { return this.movieName; } public int getNumMinutes() { return this.numMinutes; } public boolean getIsKidFriendly() { return this.isKidFriendly; } public boolean isKidFriendly() { return this.isKidFriendly; } public int getNumCastMembers() { return this.numCastMembers; } public String[] getCastMembers() { return this.castMembers.clone(); } public void setMovieName(String movieName) { this.movieName = movieName; } public void setNumMinutes(int numMinutes) { this.numMinutes = numMinutes; } public void setIsKidFriendly(boolean isKidFriendly) { this.isKidFriendly = isKidFriendly; } public boolean replaceCastMember(int index, String castMemberName) { if (index < 0 || index > numCastMembers) { return false; } castMembers[index] = castMemberName; return true; } public boolean doArraysMatch(String[] arr1, String[] arr2) { if (arr1 == null && arr2 == null) { return true; } if (arr1.length == arr2.length) { for (int i = 0; i < arr1.length; i++) { if (arr1[i] != null && arr2[i] != null) { if (! arr1[i].toLowerCase().equals(arr2[i].toLowerCase())) { return false; } } } return true; } return false; } public String getCastMemberNamesAsString() { if (numCastMembers == 0) { return "none"; } String names = castMembers[0]; for (int i = 1; i < numCastMembers; i++) { names += ", " + castMembers[i]; } return names; } public String toString() { String friendly; if (isKidFriendly()) { friendly = "kid friendly"; } else { friendly = "not kid freindly"; } return "Movie:" + " [ Minutes " + getNumMinutes() + " " + "| Movie Name: " + getMovieName() + " " + "| " + friendly + " " + "| Number of Cast Members: " + getNumCastMembers() + " " + "| Cast Members: " + getCastMemberNamesAsString() + " " + "]"; } public boolean equals(Object o) { Movie m = (Movie) o; return movieName.equals(m.getMovieName()) && numMinutes == m.getNumMinutes() && doArraysMatch(castMembers, m.getCastMembers()); } public static void main(String[] args) { Movie movie = new Movie("The Shawshank Redemption", 142, false, new String[] { "Tim Robbins", "Morgan Freeman", "Bob Gunton" }); Movie movie1 = new Movie(" Aladin", 90, true, new String[] { "Scott Weigner", "Robin Williams", "Linda Larkin", "Jonathan Freeman" }); String[] castMembers = new String[] { "Tim Robbins", "Morgan Freeman", "Bob Gunton" }; System.out.println(movie.toString()); System.out.println(movie1.toString()); System.out.println("\ncast members are same : "+movie.doArraysMatch(movie.castMembers, castMembers)); System.out.println("\nCast Members of movie 1 "+movie1.getCastMemberNamesAsString()); } } Errors: -java:17: error: cannot access Movie Movie m = null; -java:15: error: cannot access Movie Movie m = null; -java:29: error: cannot access Movie Movie m1 = null; -java:26: error: cannot access Movie Movie m1 = null; -java:16: error: cannot access Movie field = Movie.class.getDeclaredField(str); - java:13: error: cannot access Movie constructor = Movie.class.getConstructor(); -java:13: error: cannot access Movie constructor = Movie.class.getConstructor(String.class, int.class, boolean.class, String[].class); - java:12: error: cannot access Movie Method methodGetNumMinutes = Movie.class.getMethod("getNumMinutes"); -java:12: error: cannot access Movie Method methodSetNumMinutes = Movie.class.getMethod("setNumMinutes", int.class); - java:12: error: cannot access Movie Method replaceCastMember = Movie.class.getMethod("replaceCastMember", int.class, String.class);
How do I fix the Java code errors?
Code:
//import java.util.Arrays;
//Movie.java
package movie;
public class Movie {
private String movieName;
private int numMinutes;
private boolean isKidFriendly;
private int numCastMembers;
private String[] castMembers;
public Movie() {
movieName = "Flick";
numMinutes = 0;
isKidFriendly = false;
numCastMembers = 0;
castMembers = new String[10];
}
public Movie(String movieName, int numMinutes, boolean isKidFriendly, String[] castMembers) {
this.movieName = movieName;
this.numMinutes = numMinutes;
this.isKidFriendly = isKidFriendly;
this.numCastMembers = castMembers.length;
this.castMembers = castMembers;
}
public String getMovieName() {
return this.movieName;
}
public int getNumMinutes() {
return this.numMinutes;
}
public boolean getIsKidFriendly() {
return this.isKidFriendly;
}
public boolean isKidFriendly() {
return this.isKidFriendly;
}
public int getNumCastMembers() {
return this.numCastMembers;
}
public String[] getCastMembers() {
return this.castMembers.clone();
}
public void setMovieName(String movieName) {
this.movieName = movieName;
}
public void setNumMinutes(int numMinutes) {
this.numMinutes = numMinutes;
}
public void setIsKidFriendly(boolean isKidFriendly) {
this.isKidFriendly = isKidFriendly;
}
public boolean replaceCastMember(int index, String castMemberName) {
if (index < 0 || index > numCastMembers) {
return false;
}
castMembers[index] = castMemberName;
return true;
}
public boolean doArraysMatch(String[] arr1, String[] arr2) {
if (arr1 == null && arr2 == null) {
return true;
}
if (arr1.length == arr2.length) {
for (int i = 0; i < arr1.length; i++) {
if (arr1[i] != null && arr2[i] != null) {
if (! arr1[i].toLowerCase().equals(arr2[i].toLowerCase())) {
return false;
}
}
}
return true;
}
return false;
}
public String getCastMemberNamesAsString() {
if (numCastMembers == 0) {
return "none";
}
String names = castMembers[0];
for (int i = 1; i < numCastMembers; i++) {
names += ", " + castMembers[i];
}
return names;
}
public String toString() {
String friendly;
if (isKidFriendly()) {
friendly = "kid friendly";
} else {
friendly = "not kid freindly";
}
return "Movie:" + " [ Minutes " + getNumMinutes() + " " + "| Movie Name: " + getMovieName() + " " + "| "
+ friendly + " " + "| Number of Cast Members: " + getNumCastMembers() + " " + "| Cast Members: "
+ getCastMemberNamesAsString() + " " + "]";
}
public boolean equals(Object o) {
Movie m = (Movie) o;
return movieName.equals(m.getMovieName()) && numMinutes == m.getNumMinutes()
&& doArraysMatch(castMembers, m.getCastMembers());
}
public static void main(String[] args) {
Movie movie = new Movie("The Shawshank Redemption", 142, false,
new String[] { "Tim Robbins", "Morgan Freeman", "Bob Gunton" });
Movie movie1 = new Movie(" Aladin", 90, true,
new String[] { "Scott Weigner", "Robin Williams", "Linda Larkin", "Jonathan Freeman" });
String[] castMembers = new String[] { "Tim Robbins", "Morgan Freeman", "Bob Gunton" };
System.out.println(movie.toString());
System.out.println(movie1.toString());
System.out.println("\ncast members are same : "+movie.doArraysMatch(movie.castMembers, castMembers));
System.out.println("\nCast Members of movie 1 "+movie1.getCastMemberNamesAsString());
}
}
Errors:
-java:17: error: cannot access Movie Movie m = null;
-java:15: error: cannot access Movie Movie m = null;
-java:29: error: cannot access Movie Movie m1 = null;
-java:26: error: cannot access Movie Movie m1 = null;
-java:16: error: cannot access Movie field = Movie.class.getDeclaredField(str);
- java:13: error: cannot access Movie constructor = Movie.class.getConstructor();
-java:13: error: cannot access Movie constructor = Movie.class.getConstructor(String.class, int.class, boolean.class,
String[].class);
- java:12: error: cannot access Movie Method methodGetNumMinutes = Movie.class.getMethod("getNumMinutes");
-java:12: error: cannot access Movie Method methodSetNumMinutes = Movie.class.getMethod("setNumMinutes", int.class);
- java:12: error: cannot access Movie Method replaceCastMember = Movie.class.getMethod("replaceCastMember", int.class, String.class);

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 9 images

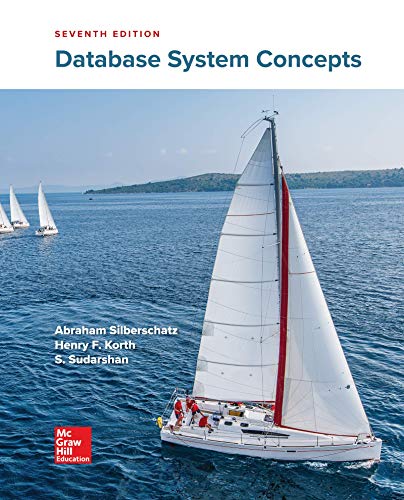
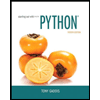
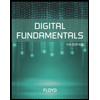
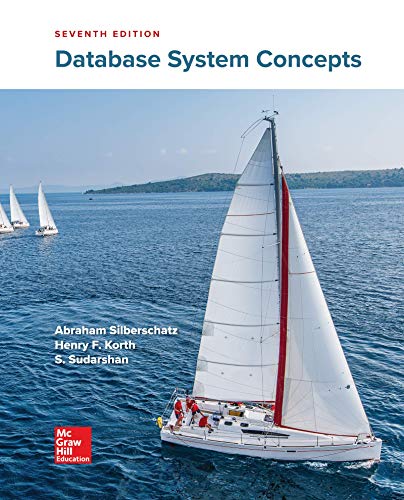
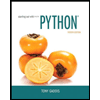
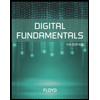
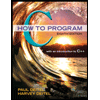
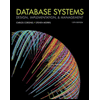
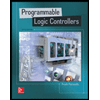