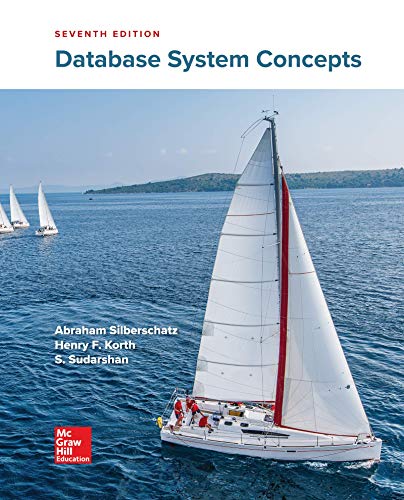
Concept explainers
How do I fix the errored outputs Java?
Code:
//import java.util.Arrays;
//Movie.java
public class Movie {
private String movieName;
private int numMinutes;
private boolean isKidFriendly;
private int numCastMembers;
private String[] castMembers;
public Movie() {
movieName = "Flick";
numMinutes = 0;
isKidFriendly = false;
numCastMembers = 0;
castMembers = new String[10];
}
public Movie(String movieName, int numMinutes, boolean isKidFriendly, String[] castMembers) {
this.movieName = movieName;
this.numMinutes = numMinutes;
this.isKidFriendly = isKidFriendly;
this.numCastMembers = castMembers.length;
this.castMembers = castMembers;
}
public String getMovieName() {
return this.movieName;
}
public int getNumMinutes() {
return this.numMinutes;
}
public boolean getIsKidFriendly() {
return this.isKidFriendly;
}
public boolean isKidFriendly() {
return this.isKidFriendly;
}
public int getNumCastMembers() {
return this.numCastMembers;
}
public String[] getCastMembers() {
return this.castMembers.clone();
}
public void setMovieName(String movieName) {
this.movieName = movieName;
}
public void setNumMinutes(int numMinutes) {
this.numMinutes = numMinutes;
}
public void setIsKidFriendly(boolean isKidFriendly) {
this.isKidFriendly = isKidFriendly;
}
public boolean replaceCastMember(int index, String castMemberName) {
if (index < 0 || index > numCastMembers) {
return false;
}
castMembers[index] = castMemberName;
return true;
}
public boolean doArraysMatch(String[] arr1, String[] arr2) {
if (arr1 == null && arr2 == null) {
return true;
}
if (arr1.length == arr2.length) {
for (int i = 0; i < arr1.length; i++) {
if (arr1[i] != null && arr2[i] != null) {
if (! arr1[i].toLowerCase().equals(arr2[i].toLowerCase())) {
return false;
}
}
}
return true;
}
return false;
}
public String getCastMemberNamesAsString() {
if (numCastMembers == 0) {
return "none";
}
String names = castMembers[0];
for (int i = 1; i < numCastMembers; i++) {
names += ", " + castMembers[i];
}
return names;
}
public String toString() {
String friendly;
if (isKidFriendly()) {
friendly = "kid friendly";
} else {
friendly = "not kid freindly";
}
return "Movie:" + " [ Minutes " + getNumMinutes() + " " + "| Movie Name: " + getMovieName() + " " + "| "
+ friendly + " " + "| Number of Cast Members: " + getNumCastMembers() + " " + "| Cast Members: "
+ getCastMemberNamesAsString() + " " + "]";
}
public boolean equals(Object o) {
Movie m = (Movie) o;
return movieName.equals(m.getMovieName()) && numMinutes == m.getNumMinutes()
&& doArraysMatch(castMembers, m.getCastMembers());
}
public static void main(String[] args) {
Movie movie = new Movie("The Shawshank Redemption", 142, false,
new String[] { "Tim Robbins", "Morgan Freeman", "Bob Gunton" });
Movie movie1 = new Movie(" Aladin", 90, true,
new String[] { "Scott Weigner", "Robin Williams", "Linda Larkin", "Jonathan Freeman" });
String[] castMembers = new String[] { "Tim Robbins", "Morgan Freeman", "Bob Gunton" };
System.out.println(movie.toString());
System.out.println(movie1.toString());
System.out.println("\ncast members are same : "+movie.doArraysMatch(movie.castMembers, castMembers));
System.out.println("\nCast Members of movie 1 "+movie1.getCastMemberNamesAsString());
}
}
Errors:
1. Error ---------- Exception occurred while calling Movie.doArraysMatch(String[] arr1, String[] arr2)
2. Error ---------- Movie.toString() returns:
Movie: [ Minutes 142 | Movie Name: The Shawshank Redemption | not kid freindly | Number of Cast Members: 3 | Cast Members: Tim Robins, Morgan Freeman, Bob Guton ]
instead of:
Movie: [ Minutes 142 | Movie Name: The Shawshank Redemption | not kid friendly | Number of Cast Members: 3 | Cast Members: Tim Robins, Morgan Freeman, Bob Guton ]
3. Error ---------- Exception occurred while calling Movie.equals()
4. FAILED ---- numCastMembers expected: 0
FAILED Overloaded Constructor test
5. java.lang.ArrayIndexOutOfBoundsException: Index 10 out of bounds for length 10
OK --- method replaceCastMember exists, will test functionality now

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- How do I fix the Java code errors? Code: //import java.util.Arrays;//Movie.java package movie; public class Movie { private String movieName; private int numMinutes; private boolean isKidFriendly; private int numCastMembers; private String[] castMembers; public Movie() { movieName = "Flick"; numMinutes = 0; isKidFriendly = false; numCastMembers = 0; castMembers = new String[10]; } public Movie(String movieName, int numMinutes, boolean isKidFriendly, String[] castMembers) { this.movieName = movieName; this.numMinutes = numMinutes; this.isKidFriendly = isKidFriendly; this.numCastMembers = castMembers.length; this.castMembers = castMembers; } public String getMovieName() { return this.movieName; } public int getNumMinutes() { return this.numMinutes; } public boolean getIsKidFriendly() { return this.isKidFriendly; } public boolean isKidFriendly() {…arrow_forwardimport java.util.Scanner;/** This program computes the time required to double an investment with an annual contribution.*/public class DoubleInvestment{ public static void main(String[] args) { final double RATE = 5; final double INITIAL_BALANCE = 10000; final double TARGET = 2 * INITIAL_BALANCE; Scanner in = new Scanner(System.in); System.out.print("Annual contribution: "); double contribution = in.nextDouble(); double balance = INITIAL_BALANCE; int year = 0; // TODO: Add annual contribution, but not in year 0 do { year++; double interest = balance*(RATE/100); balance = (balance+contribution+interest); } while(balance< TARGET); System.out.println("Year: " + year); System.out.printf("Balance: %.2f%n", balance); }}arrow_forwardImage attachedarrow_forward
- Fix all the errors and send the code please // Application looks up home price // for different floor plans // allows upper or lowercase data entry import java.util.*; public class DebugEight3 { public static void main(String[] args) { Scanner input = new Scanner(System.in); String entry; char[] floorPlans = {'A','B','C','a','b','c'} int[] pricesInThousands = {145, 190, 235}; char plan; int x, fp = 99; String prompt = "Please select a floor plan\n" + "Our floorPlanss are:\n" + "A - Augusta, a ranch\n" + "B - Brittany, a split level\n" + "C - Colonial, a two-story\n" + "Enter floorPlans letter"; System.out.println(prompt); entry = input.next(); plan = entry.charAt(1); for(x = 0; x < floorPlans.length; ++x) if(plan == floorPlans[x]) x = fp; if(fp = 99) System.out.println("Invalid floor plan code entered")); else { if(fp…arrow_forward1. Insert the missing part of the code below to output the string. public class MyClass { public static void main(String[] args) { ("Hello, I am a Computer Engineer");arrow_forwardHow do I make the Java code output? //import java.util.Arrays;public class Movie {private String movieName;private int numMinutes;private boolean isKidFriendly;private int numCastMembers;private String[] castMembers;// default constructorpublic Movie() {this.movieName = "Flick";this.numMinutes = 0;this.isKidFriendly = false;this.numCastMembers = 0;this.castMembers = new String[10]; }// overloaded parameterized constructorpublic Movie(String movieName, int numMinutes, boolean isKidFriendly, String[] castMembers) {this.movieName = movieName;this.numMinutes = numMinutes;this.isKidFriendly = isKidFriendly;//numCastMember is set to array lengththis.numCastMembers = castMembers.length;this.castMembers = castMembers; }public String getMovieName() {return this.movieName; }public int getNumMinutes() {return this.numMinutes; }public boolean getIsKidFriendly() {return this.isKidFriendly; }public boolean isKidFriendly() {return this.isKidFriendly; }public int getNumCastMembers() {return…arrow_forward
- import java.util.*; class Money { private int dollar; private int cent; final static int MIN_CENT_VALUE = 0; final static int MAX_CENT_VALUE = 99; public Money() { this.dollar = 0; this.cent = 0; } public Money(int dollar, int cent) { this.dollar = dollar; if (cent > MIN_CENT_VALUE && cent <= MAX_CENT_VALUE) { this.cent = cent; } else { cent = 0; } } public int getDollar() { return this.dollar; } public void setDollar(int dol) { this.dollar = dol; } public int getCent() { return this.cent; } public void setCent(int c) { if (c >= MIN_CENT_VALUE && c <= MAX_CENT_VALUE) { this.cent = c; } } public Money add(Money otherMoney) { Money m = new Money(); m.dollar = this.dollar + otherMoney.dollar; m.cent = this.cent + otherMoney.cent; if (m.cent >= 100) {…arrow_forwardQuestion 37 public static void main(String[] args) { Dog[] dogs = { new Dog(), new Dog()}; for(int i = 0; i >>"+decision()); } class Counter { private static int count; public static void inc() { count++;} public static int getCount() {return count;} } class Dog extends Counter{ public Dog(){} public void wo(){inc();} } class Cat extends Counter{ public Cat(){} public void me(){inc();} } The Correct answer: Nothing is output O 2 woofs and 5 mews O 2 woofs and 3 mews O 5 woofs and 5 mews Oarrow_forwardHow do I fix the errors? Code: import java.util.Scanner;public class ReceiptMaker { public static final String SENTINEL = "checkout";public final int MAX_NUM_ITEMS;public final double TAX_RATE;private String[] itemNames;private double[] itemPrices;private int numItemsPurchased; public ReceiptMaker() {MAX_NUM_ITEMS = 10;TAX_RATE = 0.0875;itemNames = new String[MAX_NUM_ITEMS];itemPrices = new double[MAX_NUM_ITEMS];numItemsPurchased = 0;}public ReceiptMaker(int maxNumItems, double taxRate) {MAX_NUM_ITEMS = maxNumItems;TAX_RATE = taxRate;itemNames = new String[MAX_NUM_ITEMS];itemPrices = new double[MAX_NUM_ITEMS];numItemsPurchased = 0;} public void greetUser() {System.out.println("Welcome to the " + MAX_NUM_ITEMS + " items or less checkout line");}public void promptUserForProductEntry() {System.out.println("Enter item #" + (numItemsPurchased + 1) + "'s name and price separated by a space, or enter \"checkout\" to end transaction early");}public void addNextPurchaseItemFromUser(String…arrow_forward
- //Assignment 06 */public static void main[](String[] args) { String pass= "ICS 111"; System.out.printIn(valPassword(pass));} /* public static boolean valPassword(String password){ if(password.length() > 6) { if(checkPass(password) { return true; } else { return false; } }else System.out.print("Too small"); return false;} public static boolean checkPass (String password){ boolean hasNum=false; boolean hasCap = false; boolean hasLow = false; char c; for(int i = 0; i < password.length(); i++) { c = password.charAt(1); if(Character.isDigit(c)); { hasNum = true; } else if(Character.isUpperCase(c)) { hasCap = true; } else if(Character.isLowerCase(c)) { hasLow = true; } } return true; { return false; } }arrow_forwardcomplete the missing code. public class Exercise09_04Extra { public static void main(String[] args) { SimpleTime time = new SimpleTime(); time.hour = 2; time.minute = 3; time.second = 4; System.out.println("Hour: " + time.hour + " Minute: " + time.minute + " Second: " + time.second); } } class SimpleTime { // Complete the code to declare the data fields hour, // minute, and second of the int type }arrow_forwardclass TenNums {private: int *p; public: TenNums() { p = new int[10]; for (int i = 0; i < 10; i++) p[i] = i; } void display() { for (int i = 0; i < 10; i++) cout << p[i] << " "; }};int main() { TenNums a; a.display(); TenNums b = a; b.display(); return 0;} Continuing from Question 4, let's say I added the following overloaded operator method to the class. Which statement will invoke this method? TenNums TenNums::operator+(const TenNums& b) { TenNums o; for (int i = 0; i < 10; i++) o.p[i] = p[i] + b.p [i]; return o;} Group of answer choices TenNums a; TenNums b = a; TenNums c = a + b; TenNums c = a.add(b);arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
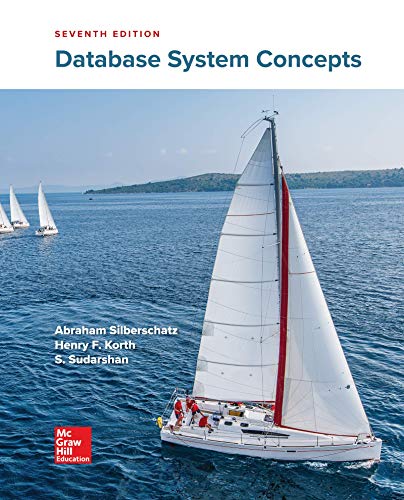
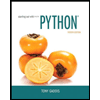
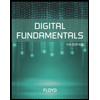
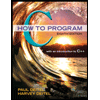
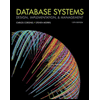
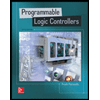