#ifndef SALES_H #define SALES_H #include using std:: string; class Sales { private: /* Write your code here */ string id; int year; string name; int amountSold; public: // constructors /* Write your code here */ //default contructors Sales (); //overloaded cóntructors Sales (string id, int year, string name, int amountSold) // setters /* Write your code here */ void setId (string givenId); void setYear (int givenYear); void setName (string givenName); void setAmount (int givenAmount); // getters /* Write your code here */ string getId(); int getYear (); string getName(); int getAmount(); // other functions /* Write your code here */ void display() const; double detAmountEarned() const; double detCommissionPercent() const; #endif
The average amount Sold function is not run as expect. Please fix it.
The input file: sales.txt
header in picture. It is over 5000 char. I can't copy.
13492785 2017 Jane North; 1000
78534520 2012 Tim South; 950
20192756 2017 Linda East; 15000
19273458 2012 Paul West; 5000
78520192 2017 Mary Jane Doe; 5001
32278520 2012 Victor Smith; 7995
14278520 2012 Mary Johnson; 120
56192785 2017 Tom Baker; 1300
88278529 2012 Diana Newman; 1500
89278527 2012 William Peterson; 14200
98278528 2012 Jim Gaddis; 1200
99192785 2017 Laura King; 1000
43278524 2012 Ann McDonald; 2000
The output expect: in picture.
#include "Sales.h"
#include <iostream>
#include <sstream>
#include <iomanip>
#include <fstream>
#include <string>
using namespace std;
const int MAX_SIZE = 30;
void readData(string fileName, Sales salesArr[], int n);
double calcSalesAvg(Sales salesArr[], int n);
void displayOverAvg(Sales salesArr[], int n, double avg);
void writeReport(Sales salesArr[], int n, string fileName);
void showReport(string fileName);
int main()
{
Sales salesArr[MAX_SIZE];
int size = 0;
string fileName;
cout << "Please enter the input file's name: ";
getline(cin, fileName);
readData(fileName, salesArr, size);
double avg = calcSalesAvg(salesArr, size);
displayOverAvg(salesArr, size, avg);
writeReport(salesArr, size, fileName);
string option;
cout << "Show report?" << endl;
getline(cin, option);
if (option == "Y" || option == "y")
showReport(fileName);
return 0;
}
void readData(string fileName, Sales salesArr[], int n) {
ifstream inFile;
inFile.open(fileName);
if (!inFile) {
cout << "Input file: " << fileName << " not found!" << endl;
exit (101);
}
string line;
n = 0;
if (inFile.is_open()) {
while (getline(inFile, line)) {
stringstream ss(line);
string id;
ss >> id;
int year;
ss >> year;
string name;
getline(ss, name, ';');
int amountSold;
ss >> amountSold;
salesArr[n].setId(id);
salesArr[n].setYear(year);
salesArr[n].setName(name);
salesArr[n].setAmount(amountSold);
n++;
}
}
inFile.close();
}
double calcSalesAvg(Sales salesArr[], int n) {
double sum = 0;
for (int i = 0; i < n; i++) {
sum += salesArr[i].getAmount();
}
return (sum / n);
}
void displayOverAvg(Sales salesArr[], int n, double avg) {
cout << "Average Sales: " << fixed << setprecision(2) << avg << endl;
cout << "Salespeople with above average sales:" << endl;
int count = 0;
for (int i = 0; i < n; i++) {
if (salesArr[i].getAmount() > avg) {
cout << salesArr[i].getName() << "($" << salesArr[i].getAmount() << ")" << endl;
count++;
}
}
if (count == 0)
cout << "N/A" << endl;
}
void writeReport(Sales salesArr[], int n, string fileName) {
string outfileName;
outfileName.insert(fileName.find("."), "Report");
ofstream out(outfileName);
out << "Sales Report\n"
<< setw(20) << left << "==================== =============\n"
<< setw(20) << left << "Name" << " " << setw(9) << right << "Amount Earned\n"
<< setw(20) << left << "==================== =============\n";
out << fixed << setprecision(2);
for (int i = 0; i < n; i++) {
out << setw(20) << left << salesArr[i].getName() << " ";
out << setw(9) << right << salesArr[i].getAmount() << endl;
}
out << setw(20) << left << "==================== =============\n"
<< "Number of salespeople: " << n << endl;
cout << "Report saved in: " << outfileName << endl;
}
void showReport(string fileName){
fileName.insert(fileName.find("."), "Report");
ifstream in(fileName);
if (in.fail()){
cout << "Input file: " << fileName << " not found!" << endl;
exit(EXIT_FAILURE);
}
string line;
while (getline(in, line)){
cout << line << endl;
}
in.close();
}
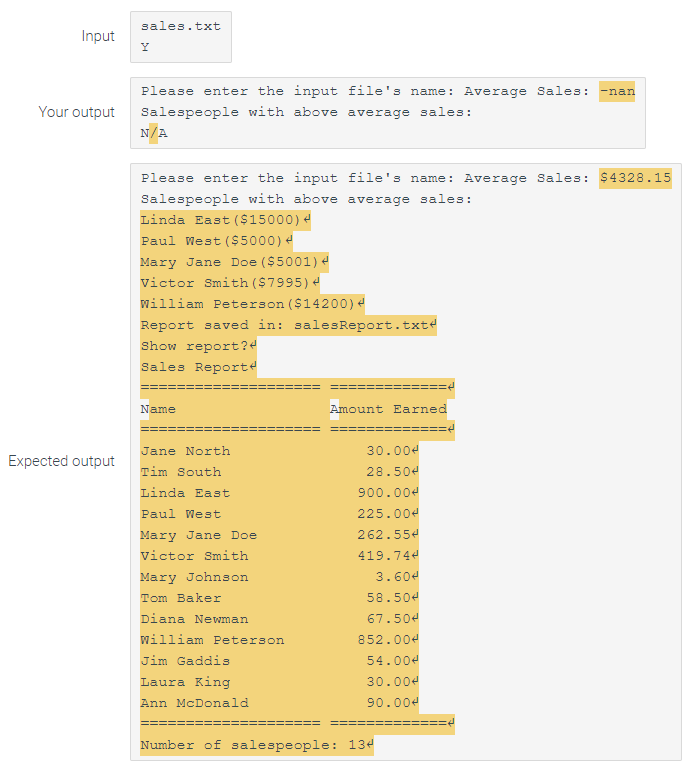
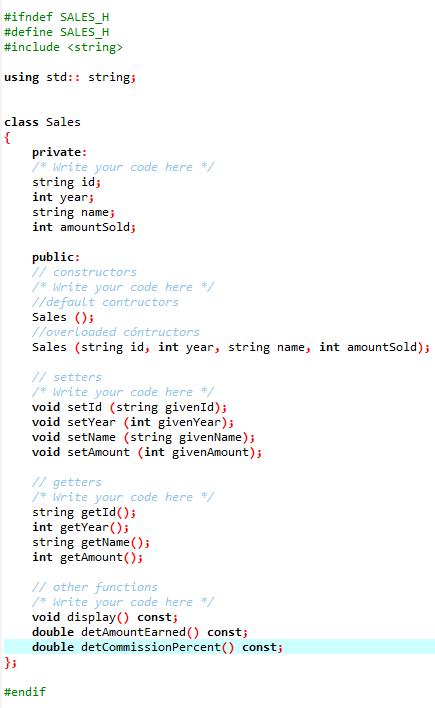

Here, I have to write a C++ program to the above question.
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

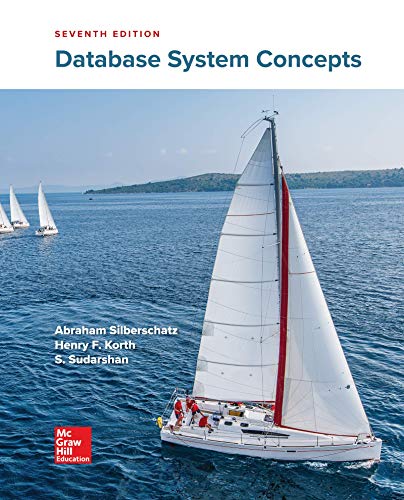
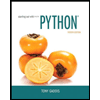
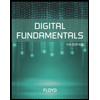
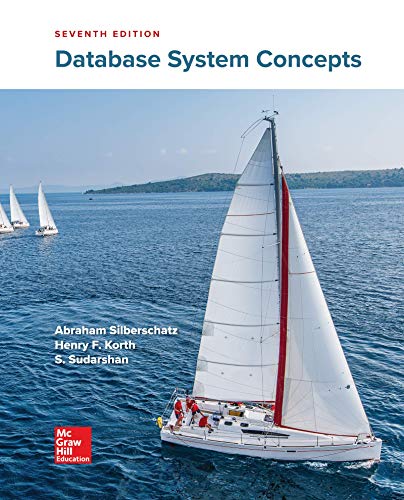
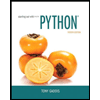
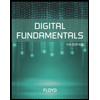
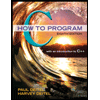
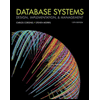
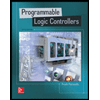