Improve this JavaScript code that will require input from the user. Below are the code that needs to be improved. //1. const square = (a) => { var res = 1; res = a * a; console.log("Num1"); console.log("Square of "+a+" is: " +res); console.log("\n"); } square(5);
Improve this JavaScript code that will require input from the user. Below are the code that needs to be improved.
//1.
const square = (a) => {
var res = 1;
res = a * a;
console.log("Num1");
console.log("Square of "+a+" is: " +res);
console.log("\n");
}
square(5);
//2.
const swap = (a,b) => {
console.log("Num2");
console.log("The value of a before swapping = ", a);
console.log("The value of b before swapping = ", b);
let temp;
temp = a; //swaps variables
a = b;
b = temp;
console.log("The value of a after swapping = ", a);
console.log("The value of b after swapping = ", b);
console.log("\n");
}
swap(7,8);
//3.
const reverse = (str) => {
const arr = []; //stores character in rev order
const len = str.length; //finds the length of the string
let i;
for(i = len; i >= 0; i--)
arr.push(str[i]); //adds the chars of str to arr
return arr.join('');
}
let stringTest, reverseString; //for dummy string
stringTest = "john gabriel";
reverseString = reverse(stringTest);
console.log("Num3");
console.log(`Reverse of the string "${stringTest}" is: "${reverseString}"`);
console.log("\n");
//4.
function check_Palindrome(str_entry){
var cstr = str_entry.toLowerCase().replace(/[^a-zA-Z0-9]+/g,''); //changes the str -> lowercase && rem all non-alpha numeric chars
var count = 0;
if(cstr === ""){
console.log("String is not found");
return false;
}
if((cstr.length) % 2 === 0){ //checks if the str is even or odd
count = (cstr.length) / 2;
} else {
console.log("Num4");
if(cstr.length === 1){ //if str length === 1 then it is palindrome
console.log("The string is a palindrome.");
return true;
} else {
count = (cstr.length - 1) / 2; //if the str length is odd, ignores the mid char
}
}
var p;
for(p = 0; p < count; p++){
if(cstr[p] != cstr.slice(-1-p)[0]){ //compares char that doesn't match
console.log("The string is not a palindrome.");
console.log("\n");
return false;
}
}
console.log("The string is a palindrome.");
return true;
}
check_Palindrome('010');
check_Palindrome('1011');
//5.
function alpha(str){
let res;
var arr = str.split(""); //splits the str
res = arr.sort().join(""); //sorts arr && forms to a str
return res;
}
console.log("Num5");
console.log(alpha("CSIT201"));

Step by step
Solved in 4 steps with 2 images

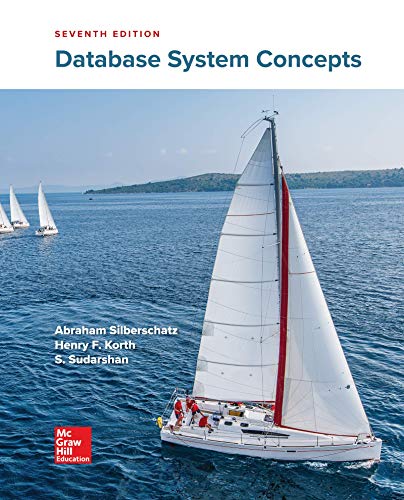
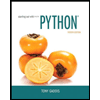
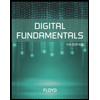
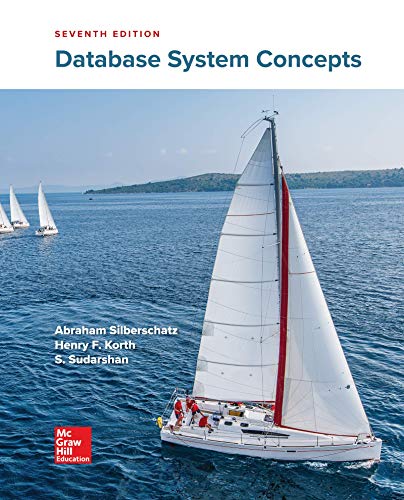
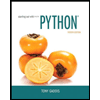
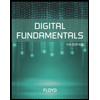
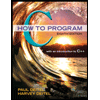
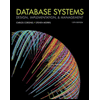
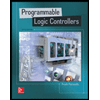