In this assignment you will develop a client-server application. The client will send the server an integer number to see whether the number is a prime number or not. The server will test the number and tell the client the result. You have to write a client code and a server code for the project using either UDP or TCP socket programming. Can I have this prime number program code in C++ or java with detailed explaination and outputs please? Can you make sure to input the prime number during the compilation. Thank you
In this assignment you will develop a client-server application. The client will send the server an integer number to see whether the number is a prime number or not. The server will test the number and tell the client the result. You have to write a client code and a server code for the project using either UDP or TCP socket programming. Can I have this prime number program code in C++ or java with detailed explaination and outputs please? Can you make sure to input the prime number during the compilation. Thank you
A+ Guide To It Technical Support
10th Edition
ISBN:9780357108291
Author:ANDREWS, Jean.
Publisher:ANDREWS, Jean.
Chapter18: Macos, Linux, And Scripting
Section: Chapter Questions
Problem 14TC
Related questions
Question
In this assignment you will develop a client-server application. The client will send the server an
integer number to see whether the number is a prime number or not. The server will test the number
and tell the client the result. You have to write a client code and a server code for the project using
either UDP or TCP socket
Can I have this prime number program code in C++ or java with detailed explaination and outputs please?
Can you make sure to input the prime number during the compilation.
Thank you
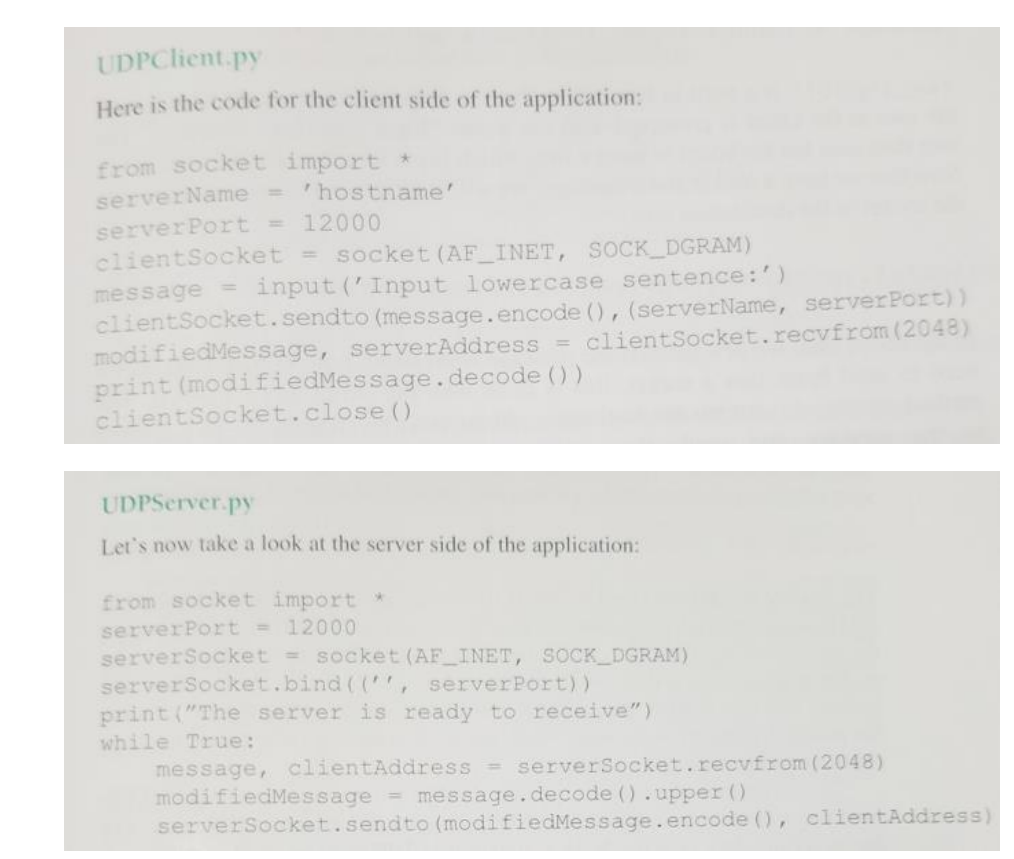
Transcribed Image Text:UDPClient.py
Here is the code for the client side of the application:
from socket import *
serverName = 'hostname'
serverPort = 12000
clientSocket = socket (AF INET, SOCK DGRAM)
message = input ('Input lowercase sentence:')
clientSocket.sendto (message.encode (), (serverName, serverPort))
modifiedMessage, serverAddress = clientSocket.recvfrom (2048)
print (modifiedMessage.decode ())
clientSocket.close ()
UDPServer.py
Let's now take a look at the server side of the application:
from socket import
serverPort = 12000
serverSocket = socket (AF INET, SOCK DGRAM)
serverSocket.bind ((",
print ("The server is ready to receive")
serverPort))
while True:
message, clientAddress serverSocket.recvfrom (2048)
modifiedMessage = message.decode ().upper ()
serverSocket.sendto (modifiedMessage.encode (), clientAddress)
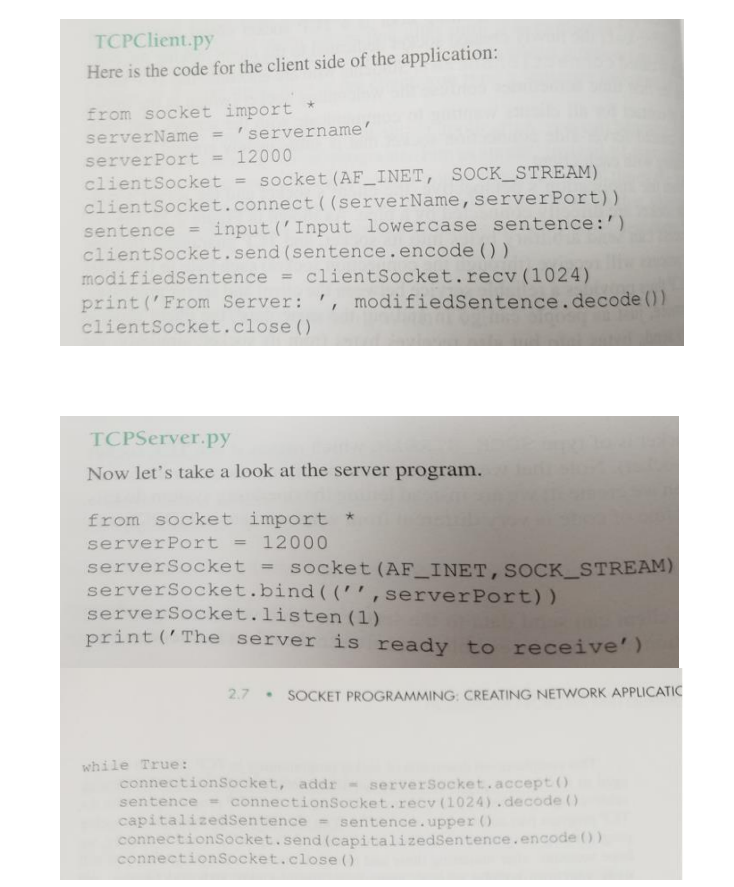
Transcribed Image Text:TCPClient.py
Here is the code for the client side of the application:
from socket import
serverName =
servername'
serverPort = 12000
clientSocket = socket (AF_INET, SOCK_STREAM)
clientSocket.connect((serverName,serverPort))
input ('Input lowercase sentence:')
sentence
%3D
clientSocket.send (sentence.encode ())
modifiedSentence = clientSocket.recv(1024)
print ('From Server: ', modifiedSentence.decode ())
clientSocket.close ()
TCPServer.py
Now let's take a look at the server program.
from socket import *
serverPort = 12000
socket (AF_INET,SOCK_STREAM)
serverSocket
serverSocket.bind (('',serverPort))
serverSocket.listen (1)
print ('The server is ready to receive')
2.7
SOCKET PROGRAMMING: CREATING NETWORK APPLICATIC
while True:
connectionSocket, addr - serverSocket.accept 0
sentence = connectionSocket.recv (1024).decode ()
capitalizedSentence sentence.upper ()
connectionSocket.send (capitalizedSentence.encode ())
connectionSocket.close ()
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
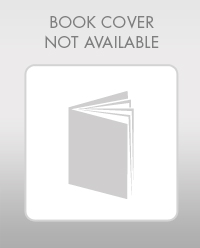
A+ Guide To It Technical Support
Computer Science
ISBN:
9780357108291
Author:
ANDREWS, Jean.
Publisher:
Cengage,
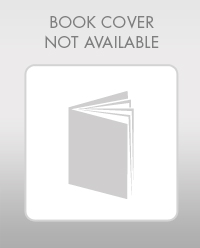
A+ Guide To It Technical Support
Computer Science
ISBN:
9780357108291
Author:
ANDREWS, Jean.
Publisher:
Cengage,