I need the Server code written in Python for the given client code. A sample working client code is given to you in Client.py. I need a corresponding Server code. The goal of the server is to get any string sent to it, and switch any letters from lower case to capital and vice-versa. Lastly it should then close gracefully when the client is done sending strings. The client code is provided below: import argparse from sys import argv import socket #First we use the argparse package to parse the aruments parser=argparse.ArgumentParser(description="""This is a very basic client program""") parser.add_argument('-f', type=str, help='This is the source file for the strings to reverse', default='source_strings.txt',action='store', dest='in_file') parser.add_argument('-o', type=str, help='This is the destination file for the reversed strings', default='results.txt',action='store', dest='out_file') parser.add_argument('server_location', type=str, help='This is the domain name or ip address of the server',action='store') parser.add_argument('port', type=int, help='This is the port to connect to the server on',action='store') args = parser.parse_args(argv[1:]) #next we create a client socket client_sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM) server_addr = (args.server_location, args.port) client_sock.connect(server_addr) #now we need to open both files with open(args.out_file, 'wb') as write_file: for line in open(args.in_file, 'rb'): #now we write whatever the server tells us to the out_file client_sock.sendall(line) answer = client_sock.recv(512) write_file.write(answer) #close the socket (note this will be visible to the other side) client_sock.close() Some output pictures would be appreciated to make sure that the code works properly. Thank You
I need the Server code written in Python for the given client code. A sample working client code is given to you in Client.py. I need a corresponding Server code. The goal of the server is to get any string sent to it, and switch any letters from lower case to capital and vice-versa. Lastly it should then close gracefully when the client is done sending strings. The client code is provided below: import argparse from sys import argv import socket #First we use the argparse package to parse the aruments parser=argparse.ArgumentParser(description="""This is a very basic client program""") parser.add_argument('-f', type=str, help='This is the source file for the strings to reverse', default='source_strings.txt',action='store', dest='in_file') parser.add_argument('-o', type=str, help='This is the destination file for the reversed strings', default='results.txt',action='store', dest='out_file') parser.add_argument('server_location', type=str, help='This is the domain name or ip address of the server',action='store') parser.add_argument('port', type=int, help='This is the port to connect to the server on',action='store') args = parser.parse_args(argv[1:]) #next we create a client socket client_sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM) server_addr = (args.server_location, args.port) client_sock.connect(server_addr) #now we need to open both files with open(args.out_file, 'wb') as write_file: for line in open(args.in_file, 'rb'): #now we write whatever the server tells us to the out_file client_sock.sendall(line) answer = client_sock.recv(512) write_file.write(answer) #close the socket (note this will be visible to the other side) client_sock.close() Some output pictures would be appreciated to make sure that the code works properly. Thank You
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
I need the Server code written in Python for the given client code.
A sample working client code is given to you in Client.py. I need a corresponding Server code. The goal of the server is to get any string sent to it, and switch any letters from lower case to capital and vice-versa. Lastly it should then close gracefully when the client is done sending strings. The client code is provided below:
import argparse
from sys import argv
import socket
#First we use the argparse package to parse the aruments
parser=argparse.ArgumentParser(description="""This is a very basic client program""")
parser.add_argument('-f', type=str, help='This is the source file for the strings to reverse', default='source_strings.txt',action='store', dest='in_file')
parser.add_argument('-o', type=str, help='This is the destination file for the reversed strings', default='results.txt',action='store', dest='out_file')
parser.add_argument('server_location', type=str, help='This is the domain name or ip address of the server',action='store')
parser.add_argument('port', type=int, help='This is the port to connect to the server on',action='store')
args = parser.parse_args(argv[1:])
#next we create a client socket
client_sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server_addr = (args.server_location, args.port)
client_sock.connect(server_addr)
#now we need to open both files
with open(args.out_file, 'wb') as write_file:
for line in open(args.in_file, 'rb'):
#now we write whatever the server tells us to the out_file
client_sock.sendall(line)
answer = client_sock.recv(512)
write_file.write(answer)
#close the socket (note this will be visible to the other side)
client_sock.close()
Some output pictures would be appreciated to make sure that the code works properly. Thank You.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
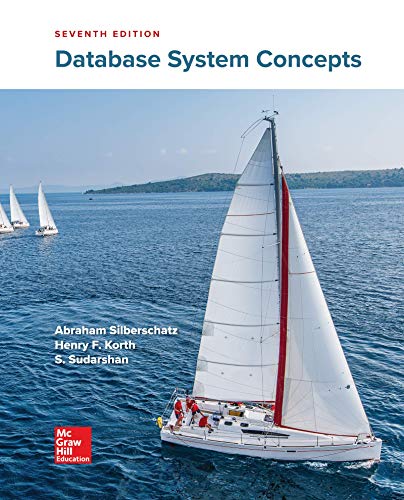
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
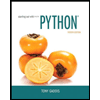
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
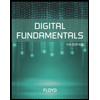
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
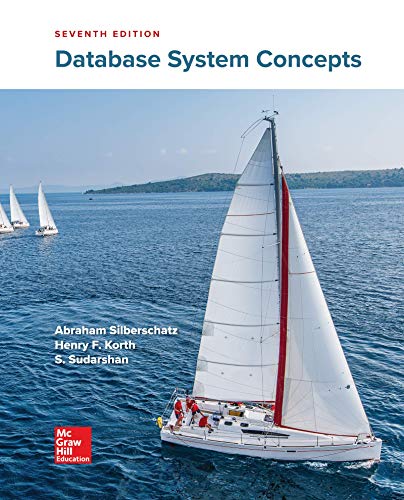
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
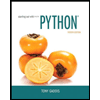
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
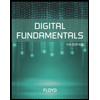
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
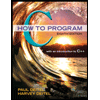
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
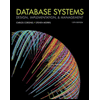
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
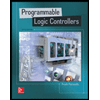
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education